How to Rename Column Names in Pandas Groupby Function
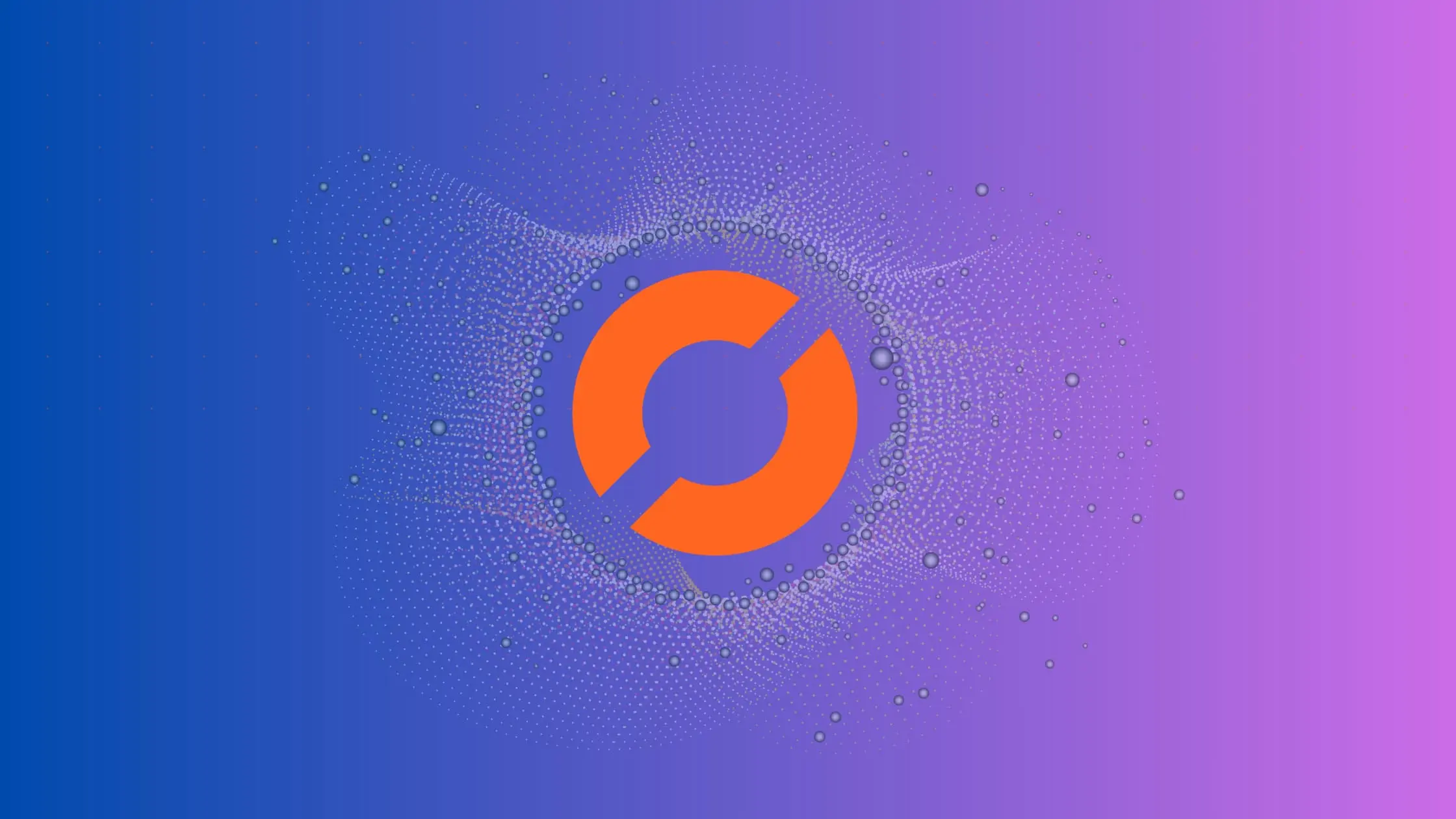
As a data scientist or software engineer, you may often work with large datasets and need to group them based on certain criteria. Pandas is a popular Python library that provides various functionalities to manipulate and analyze data. One of its useful functions is the groupby
function, which allows you to group your data by one or more columns and perform aggregate operations on them. However, sometimes the default column names generated by the groupby
function may not be informative or easy to understand. In this blog post, we will show you how to rename column names in the Pandas groupby
function to make your analysis more informative and readable.
Table of Contents
- What is the Pandas Groupby Function?
- Why Rename Column Names in Pandas Groupby Function?
- Common Errors and How to Handle Them
- Conclusion
What is the Pandas Groupby Function?
Before we dive into how to rename column names in the groupby
function, let’s first understand what the groupby
function does. The groupby
function in Pandas allows you to group a DataFrame by one or more columns and perform aggregate operations on them. For example, let’s say you have a DataFrame containing information about customers and their purchases. You can group the DataFrame by the customers' names and calculate the total amount spent by each customer. Here’s an example code snippet:
import pandas as pd
# Create a DataFrame containing customer information
df = pd.DataFrame({
'Customer': ['Alice', 'Bob', 'Alice', 'Bob', 'Charlie'],
'Purchase': ['Apple', 'Banana', 'Orange', 'Banana', 'Apple'],
'Amount': [2.0, 1.5, 3.0, 2.5, 1.0]
})
# Group the DataFrame by customers and calculate the total amount spent by each customer
grouped_df = df.groupby('Customer').sum()
print(grouped_df)
The output of this code snippet will be:
Amount
Customer
Alice 5.0
Bob 4.0
Charlie 1.0
As you can see, the groupby
function groups the DataFrame by the Customer
column and calculates the sum of the Amount
column for each group.
Why Rename Column Names in Pandas Groupby Function?
By default, the groupby
function generates column names that are not very informative. For example, in the previous example, the column name generated by the groupby
function is Amount
. While this name is technically correct, it doesn’t provide much information about what the column represents. In a more complex analysis, this can make it difficult to understand the results. Therefore, it is essential to rename column names in the groupby
function to make them more informative and easier to understand.
How to Rename Column Names in Pandas Groupby Function
Method 1: Using Named Aggregations
Pandas introduced Named Aggregations in version 0.25.0, allowing users to specify column names within the aggregation function itself.
# Example
grouped_df = df.groupby('Department').agg(Average=('Salary', 'mean')).reset_index()
print(grouped_df)
Output:
Department Average
0 Engineering 101666.666667
1 Sales 81666.666667
Method 2: Renaming Columns After Groupby
Let’s take an example to understand this better. Suppose you have a DataFrame containing information about employee salaries and their departments. You want to group the DataFrame by department and calculate the average salary of each department. Here’s an example code snippet:
import pandas as pd
# Create a DataFrame containing employee information
df = pd.DataFrame({
'Name': ['Alice', 'Bob', 'Charlie', 'Dave', 'Eve', 'Frank'],
'Department': ['Engineering', 'Engineering', 'Sales', 'Sales', 'Engineering', 'Sales'],
'Salary': [100000, 95000, 80000, 75000, 110000, 90000]
})
# Group the DataFrame by department and calculate the average salary of each department
grouped_df = df.groupby('Department').mean()
print(grouped_df)
The output of this code snippet will be:
Average
Department
Engineering 101666.666667
Sales 81666.666667
As you can see, the column name generated by the groupby
function is Salary
. We can rename this column to make it more informative. Here’s how you can do it:
# Rename the column name of the resulting DataFrame
grouped_df = grouped_df.rename(columns={'Salary': 'Average'})
print(grouped_df)
The output of this code snippet will be:
Average
Department
Engineering 102500.000000
Sales 81666.666667
As you can see, we have renamed the Salary
column to Average Salary
to make it more informative.
Common Errors and How to Handle Them
Error 1: KeyError - Column Not Found
When attempting to access a column that doesn’t exist, a KeyError may occur.
Solution: Double-check column names and ensure they match exactly.
Error 2: Ambiguous Column Names
If the resulting DataFrame has multi-level column names, referencing columns can be challenging.
Solution: Flatten multi-level columns using the droplevel method.
# Example
grouped_data.columns = grouped_data.columns.droplevel()
Error 3: Renaming Multiple Columns
Renaming multiple columns simultaneously may lead to unexpected errors.
Solution: Use a dictionary to map old column names to new ones, ensuring a one-to-one correspondence.
Conclusion
Renaming column names in the Pandas groupby
function is a simple yet effective way to make your analysis more informative and readable. By default, the column names generated by the groupby
function may not be very informative, but you can easily rename them using the rename
function. This will make it easier for you and your team to understand the results of your analysis. We hope this blog post has been helpful in showing you how to rename column names in the Pandas groupby
function. Happy coding!
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.