How to Remove Time from DateTime Variable in Pandas
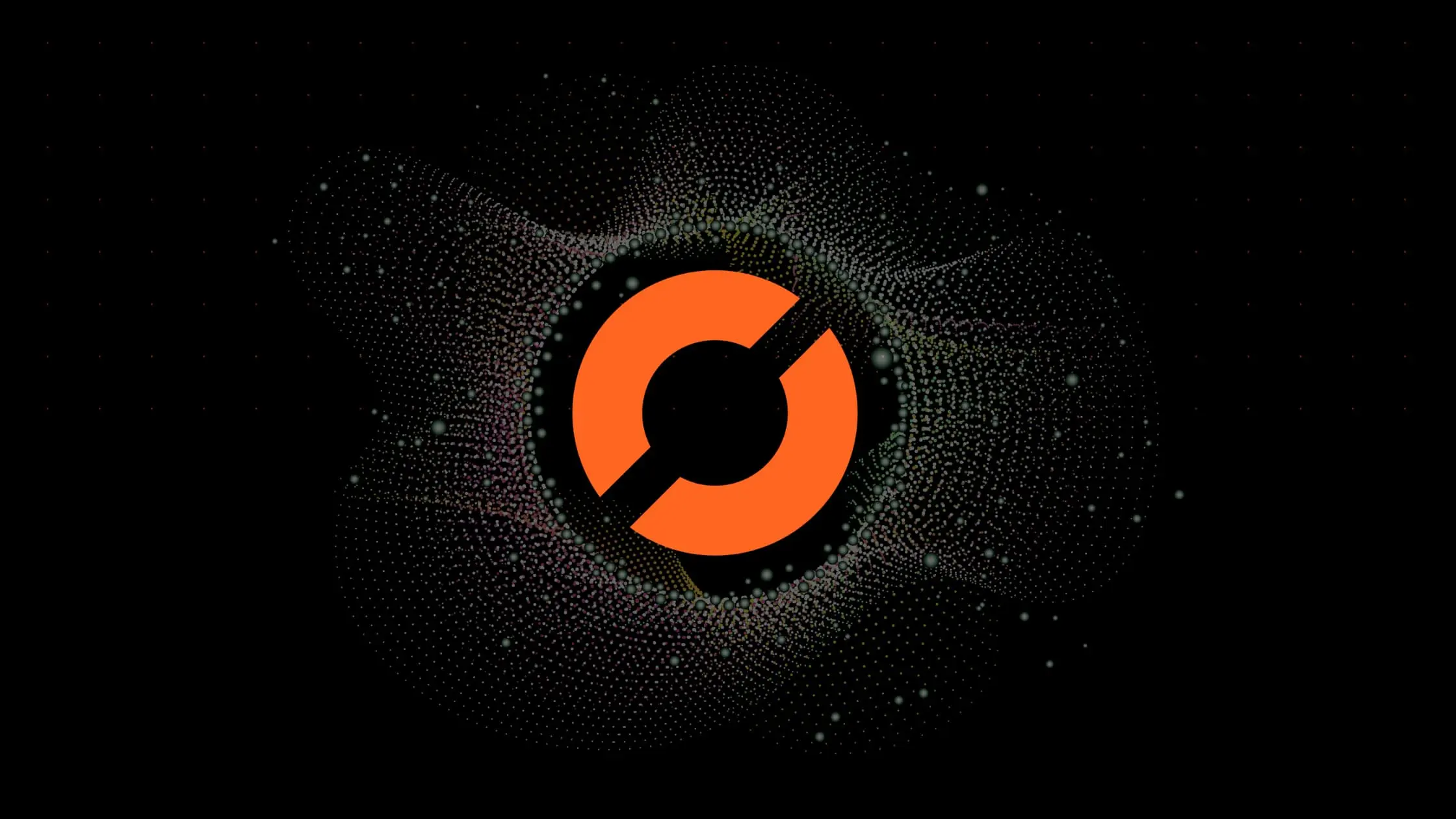
As a data scientist or software engineer, you may encounter scenarios where you need to work with date and time data in your Python code. Pandas is a popular data analysis library in Python that provides powerful tools for working with time-series data. In this post, we will explore how to remove time from a date&time variable in Pandas.
Understanding Date&Time Variables in Pandas
Before we dive into the steps of removing time from a date&time variable, let’s first understand how date&time variables work in Pandas.
In Pandas, date&time
variables are represented as datetime64[ns]
objects. These objects are based on the NumPy datetime64 data type and provide high precision datetime calculations. Pandas also provides a range of functions to perform various operations on datetime objects.
Steps to Remove Time from a Date&Time
Variable
Now that we have a basic understanding of date&time
variables in Pandas, let’s explore how to remove time from a date&time
variable. We can achieve this by using the dt
accessor provided by Pandas.
Here are the steps to remove time from a date&time
variable in Pandas:
Step 1: Convert the Variable to a Pandas Datetime Object
The first step is to convert the date&time
variable to a Pandas datetime object. We can achieve this by using the pd.to_datetime()
function provided by Pandas. This function takes a string or a sequence of strings and returns a Pandas datetime object.
import pandas as pd
# Sample date&time variable
date_time_var = '2022-06-18 10:30:00'
# Convert to Pandas datetime object
date_time_obj = pd.to_datetime(date_time_var)
Step 2: Use the dt.date
Property to Remove Time
Once we have the date&time
variable as a Pandas datetime object, we can use the dt
accessor to perform various operations on the object. To remove time from the date&time
variable, we can use the dt.date
property, which returns only the date component of the datetime object.
# Remove time from the datetime object
date_var = date_time_obj.dt.date
Step 3: Convert the Result to a String
The final step is to convert the result to a string if required. We can achieve this by using the strftime()
function provided by the datetime module in Python. This function takes a format string and returns a string representation of the date object.
# Convert the date object to a string
date_str = date_var.strftime('%Y-%m-%d')
Putting It All Together
Here’s the complete code to remove time from a date&time
variable in Pandas:
import pandas as pd
# Sample date&time variable
date_time_var = '2022-06-18 10:30:00'
# Convert to Pandas datetime object
date_time_obj = pd.to_datetime(date_time_var)
# Remove time from the datetime object
date_var = date_time_obj.date()
# Convert the date object to a string
date_str = date_var.strftime('%Y-%m-%d')
print(date_str)
Output:
2022-06-18
This code will output 2022-06-18
, which is the date component of the original date&time
variable.
Conclusion
In this post, we explored how to remove time from a date&time
variable in Pandas. We learned that Pandas represents date&time
variables as datetime64[ns]
objects and provides a range of functions to perform various operations on these objects. We also learned how to use the dt
accessor to remove time from a date&time
variable.
By following the steps outlined in this post, you can easily remove time from a date&time
variable in Pandas and perform further analysis on the date component of the variable.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.