How to Remove Special Characters in Pandas Dataframe
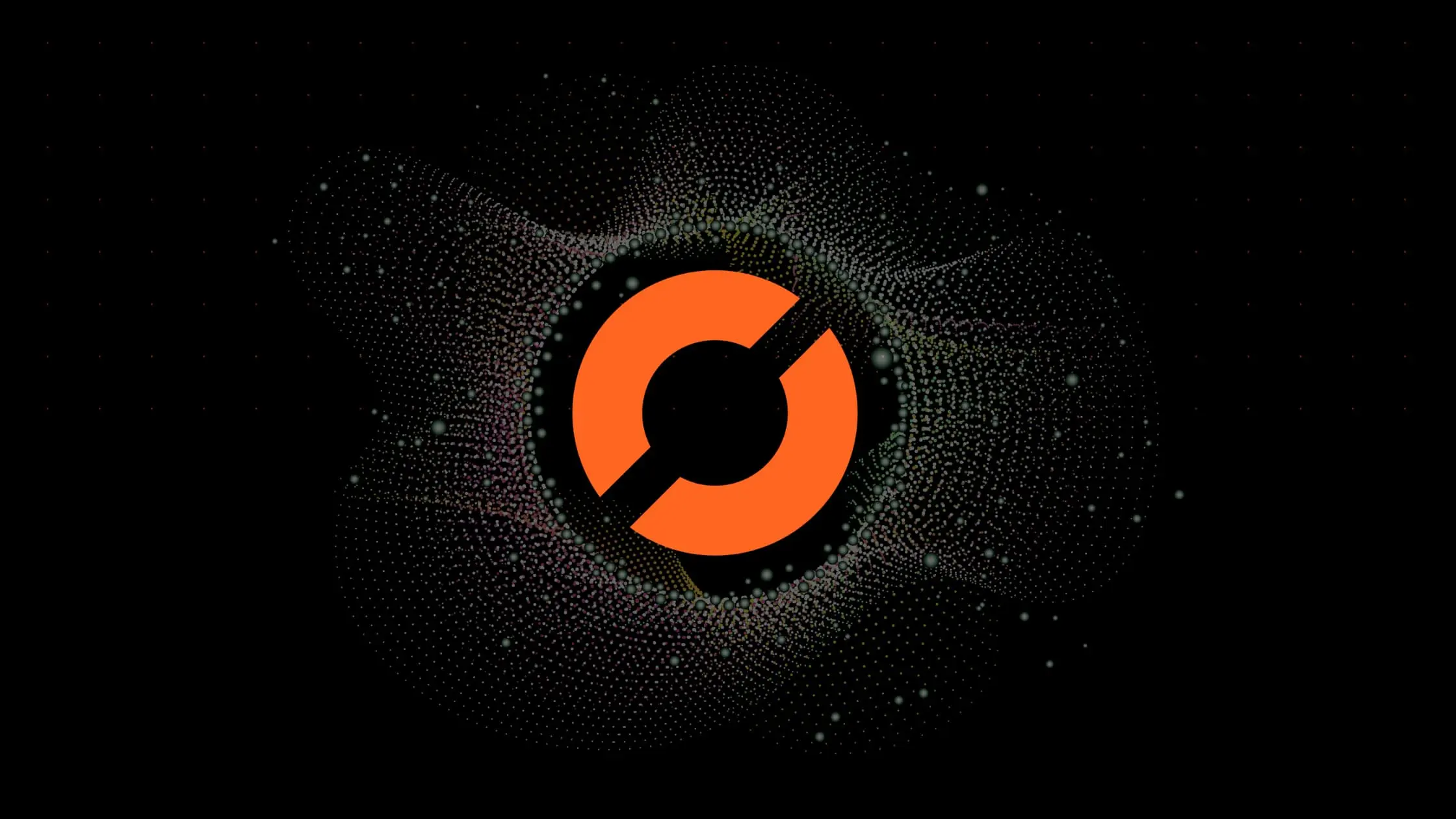
As a data scientist or software engineer, you may encounter datasets that contain special characters or symbols that can cause issues when performing data analysis. These special characters can be anything from punctuation marks to emojis that do not add any value to the data analysis process but can cause problems when trying to manipulate the data.
In this article, we will discuss how to remove special characters in Pandas Dataframe to ensure that your data is clean and ready for analysis.
Table of Contents
- Introduction
- What are Special Characters?
- How to Remove Special Characters in Pandas Dataframe
- Conclusion
What are Special Characters?
Special characters are characters that are not letters or numbers. They can include punctuation marks such as commas, periods, and question marks, as well as symbols such as emojis, mathematical symbols, and currency symbols. Special characters can also include whitespace characters such as tabs and newlines.
Special characters can cause issues when working with data because they can affect the accuracy of the analysis. For example, if you are trying to perform a calculation on a column that contains special characters, you may encounter errors or incorrect results.
How to Remove Special Characters in Pandas Dataframe
Use regular expressions
To remove special characters in Pandas Dataframe, we can use regular expression. Firsty, we need define the regular expression patterns, then we use replace
method to remove special characters.
import pandas as pd
import re
# Create a sample DataFrame
df = pd.DataFrame({'text': ['This is a sample text!', 'This is another text with special characters 😊']})
# Define the regular expression pattern
pattern = r'[^\w\s]'
# Use regular expressions to remove special characters from the 'text' column
df['text'] = df['text'].replace(pattern, '', regex=True)
# Print the updated DataFrame
print(df)
Output:
text
0 This is a sample text
1 This is another text with special characters
In this method with regular expressions, the pattern r'[^\w\s]'
matches any character that is not a word character (letter or digit) or a whitespace character, effectively removing special characters.
Use Lambda Function:
import pandas as pd
# Create a sample DataFrame
df = pd.DataFrame({'text': ['This is a sample text!', 'This is another text with special characters 😊']})
# Use a lambda function to remove special characters from the 'text' column
df['text'] = df['text'].apply(lambda x: ''.join(char for char in x if char.isalnum() or char.isspace()))
# Print the updated DataFrame
print(df)
Output:
text
0 This is a sample text
1 This is another text with special characters
In this method with the lambda function, the lambda function lambda x: ‘'.join(char for char in x if char.isalnum()
or char.isspace()
) iterates through each character in the string, keeping only alphanumeric characters and spaces, and then joins them to form the new string.
Use Regex Substitution:
# Import the pandas library for data manipulation
import pandas as pd
# Import the regular expressions module
import re
# Create a sample DataFrame
df = pd.DataFrame({'text': ['This is a sample text!', 'This is another text with special characters 😊']})
# Define the regular expression pattern
pattern = r'[^\w\s]'
# Use regex substitution to remove special characters from the 'text' column
df['text'] = df['text'].apply(lambda x: re.sub(pattern, '', x))
# Print the updated DataFrame
print(df)
Output:
text
0 This is a sample text
1 This is another text with special characters
This expression uses the re.sub()
function from the regular expressions module to replace all characters that match the pattern r'[^\w\s]'
with an empty string (''). This pattern matches any character that is not an alphanumeric character (\w) or a space (\s), effectively removing special characters.
Use ASCII Filtering:
import pandas as pd
# Create a sample DataFrame
df = pd.DataFrame({'text': ['This is a sample text!', 'This is another text with special characters 😊']})
# Use ASCII filtering to remove non-ASCII characters from the 'text' column
df['text'] = df['text'].apply(lambda x: ''.join(char for char in x if ord(char) < 128))
# Print the updated DataFrame
print(df)
Output:
text
0 This is a sample text!
1 This is another text with special characters
This expression filters characters based on their ASCII values. Non-ASCII characters have ASCII values greater than 127, so this condition effectively removes those non-ASCII characters by filtering them out.
Feel free to adapt these methods based on your specific requirements and the types of special characters you want to remove.
Conclusion
In summary, this article explored various methods for removing special characters from a Pandas DataFrame, emphasizing the importance of cleaning data before analysis. The techniques presented, such as using regular expressions, lambda functions, Regex Substitution, and ASCII Filtering provide flexible approaches to ensure accurate results. It is crucial to choose the method that suits your specific needs to ensure data cleanliness and analysis reliability. By ensuring data quality, you contribute to more robust and meaningful data analyses.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.