How to Remove Rows in a Spark Dataframe Based on Position: A Guide
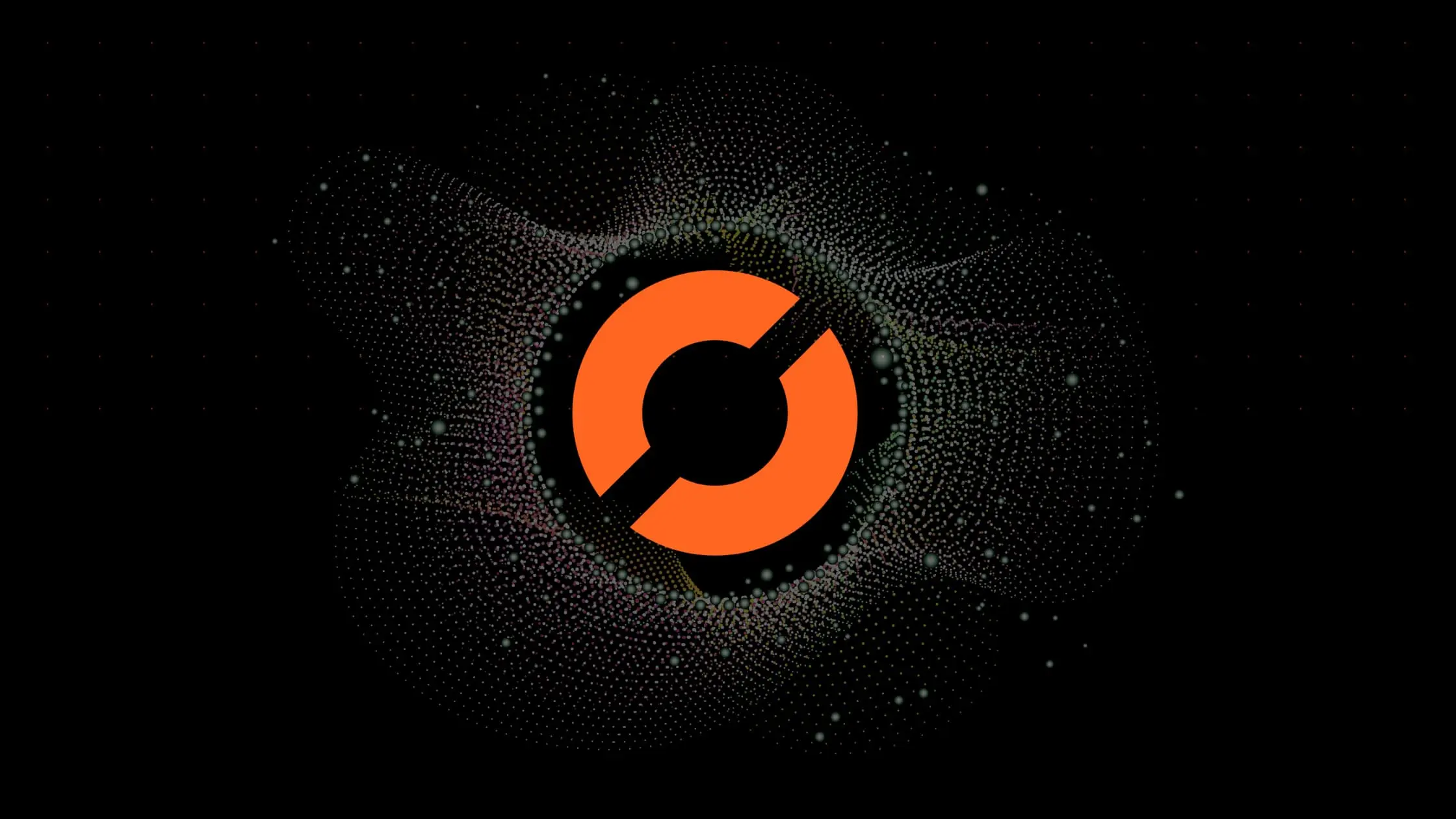
Spark is a powerful tool for data processing, but sometimes, you may find yourself needing to remove rows based on their position, not their value. This is not as straightforward as it might seem, but don’t worry, we’ve got you covered. In this blog post, we’ll walk you through the process step by step.
Table of Contents
- Introduction to Apache Spark
- Understanding Spark DataFrames
- Removing Rows in Spark DataFrame
- Common Errors and Solutions
- Conclusion
Introduction to Apache Spark
Apache Spark is a unified analytics engine for large-scale data processing. It provides an interface for programming entire clusters with implicit data parallelism and fault tolerance. Spark’s core functionality is its in-memory computing capability, which increases the processing speed of applications.
Understanding Spark DataFrames
A DataFrame in Spark is a distributed collection of data organized into named columns. It is conceptually equivalent to a table in a relational database or a data frame in Python, but with optimizations for speed and functionality.
While Spark DataFrame provides a flexible interface for distributed data manipulation, it doesn’t directly support row-level deletion. However, with a bit of creativity, we can achieve this.
Removing Rows in Spark DataFrame
To remove rows based on their position, we’ll need to add an index column to the DataFrame, which will allow us to identify each row’s position. Once we have this, we can filter out the rows we don’t want.
Here’s a step-by-step guide:
Step 1: Add an Index Column
First, we need to add an index column to our DataFrame. We can do this using the zipWithIndex
method in RDD and then convert it back to DataFrame.
from pyspark.sql import SparkSession
from pyspark.sql.functions import monotonically_increasing_id
# Create SparkSession
spark = SparkSession.builder.appName('RemoveRows').getOrCreate()
# Load DataFrame
df = spark.read.csv('data.csv', header=True, inferSchema=True)
# Add index
df = df.withColumn('index', monotonically_increasing_id())
df.show()
Output:
+-------+---+------+-----+
| Name|Age|Salary|index|
+-------+---+------+-----+
| John| 25| 50000| 0|
| Alice| 30| 60000| 1|
| Bob| 28| 55000| 2|
| Eva| 35| 70000| 3|
|Charlie| 22| 48000| 4|
| David| 32| 62000| 5|
| Sophia| 29| 58000| 6|
| Frank| 40| 75000| 7|
| Grace| 26| 52000| 8|
| Oliver| 33| 64000| 9|
+-------+---+------+-----+
Step 2: Identify Rows to Remove
Next, we need to identify the rows we want to remove. Let’s say we want to remove the first and third rows. We create a list with these index values.
rows_to_remove = [0, 2]
Step 3: Remove Rows
Now, we can filter out the rows we don’t want. We’ll use the filter
method to do this.
df = df.filter(~df.index.isin(rows_to_remove))
Step 4: Drop the Index Column
Finally, we can drop the index column as it’s no longer needed.
df = df.drop('index')
df.show()
Output:
+-------+---+------+-----+
| Name|Age|Salary|index|
+-------+---+------+-----+
| Alice| 30| 60000| 1|
| Eva| 35| 70000| 3|
|Charlie| 22| 48000| 4|
| David| 32| 62000| 5|
| Sophia| 29| 58000| 6|
| Frank| 40| 75000| 7|
| Grace| 26| 52000| 8|
| Oliver| 33| 64000| 9|
+-------+---+------+-----+
And that’s it! You’ve successfully removed rows from a Spark DataFrame based on their position.
Common Errors and Solutions
Error: Column not found
This error occurs when the specified column is not present in the DataFrame. Ensure the column exists or check for typos.
- Solution:
# Verify column names
print(df.columns)
Error: Index out of range
If you encounter an Index out of range
error, it means you are trying to access an index that does not exist in the DataFrame.
Solution:
# Check the DataFrame length before accessing an index
df_length = df.count()
if position_to_remove < 1 or position_to_remove > df_length:
print("Invalid position.")
Conclusion
While Apache Spark doesn’t directly support row-level deletion based on position, we’ve seen how we can achieve this with a bit of creativity and understanding of Spark’s functionality. By adding an index column, identifying the rows to remove, and using the filter
method, we can effectively remove rows based on their position in a Spark DataFrame.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.