How to Remove Duplicate Columns from pandas.read_csv()
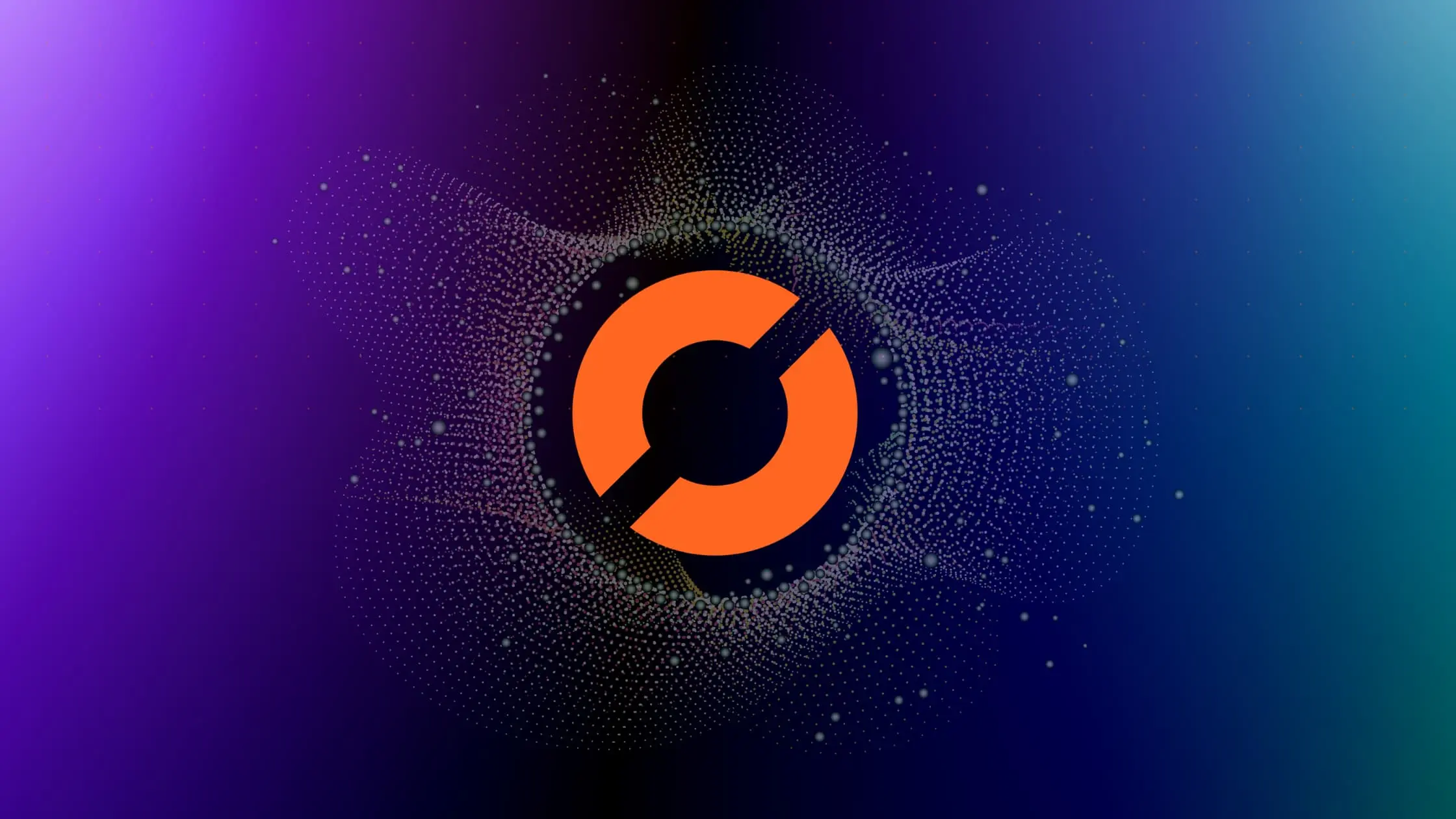
As a data scientist or software engineer, you know that data cleaning is an essential step in any data analysis project. One common issue you may encounter when working with large datasets is the presence of duplicate columns. Duplicate columns can skew your analysis results and waste valuable computational resources, so it’s important to remove them before proceeding with your analysis.
In this article, we’ll explore how to remove duplicate columns from a CSV file using the pandas library in Python. Specifically, we’ll focus on the pandas.read_csv()
function, which is a popular method for reading data from CSV files into pandas dataframes.
What are Duplicate Columns?
Duplicate columns are columns in a dataset that have identical values for every row. These columns provide no additional information and can lead to redundancy and computational inefficiencies. For example, consider the following CSV file:
Name Age Gender Age
0 Alice 25 F 25
1 Bob 30 M 30
2 Charlie 35 F 35
In this dataset, the Age
column is duplicated. Removing the duplicate column would result in the following dataset:
Name Age Gender
0 Alice 25 F
1 Bob 30 M
2 Charlie 35 F
How to Remove Duplicate Columns from pandas.read_csv()
?
To remove duplicate columns from pandas.read_csv()
, we can use the duplicated()
method.
Here’s an example code snippet that demonstrates how to remove duplicate columns from a CSV file using pandas.read_csv()
:
import pandas as pd
# Load CSV file into pandas dataframe
df = pd.read_csv('my_data.csv')
# Remove duplicate columns
df = df.loc[:, ~df.columns.duplicated()]
# Display the cleaned DataFrame
print(df)
Output:
Name Age Gender
0 Alice 25 F
1 Bob 30 M
2 Charlie 35 F
Let’s break down this code snippet step-by-step:
First, we import the pandas library using the
import pandas as pd
statement.Next, we read our CSV file into a pandas dataframe using the
pd.read_csv()
function. In this example, we assume that our CSV file is namedmy_data.csv
.We then use the
loc
method to select all rows (:
) and only columns that are not duplicated (~df.columns.duplicated()
). The~
symbol negates the boolean values returned by thedf.columns.duplicated()
method, so we end up selecting only the columns that are not duplicated.Finally, we show the cleaned dataframe.
Equivalently, you can do like this:
import pandas as pd
# Load data from CSV
df = pd.read_csv('my_data.csv')
# Identify duplicate columns
duplicate_columns = df.columns[df.columns.duplicated()]
print("Duplicate Columns:", duplicate_columns)
# Remove duplicate columns
df = df.drop(columns=duplicate_columns)
# Display the cleaned DataFrame
print(df)
Output:
Name Age Gender
0 Alice 25 F
1 Bob 30 M
2 Charlie 35 F
In the provided code snippet, we initially detect duplicate columns in the DataFrame by utilizing the duplicated()
method. This method generates a boolean Series that highlights the columns which have duplicates. Subsequently, we eliminate these duplicate columns by employing the drop()
method, where we specify the names of the columns you wish to discard.
Conclusion
In this article, we’ve shown you how to remove duplicate columns from a CSV file using the pandas.read_csv()
function in Python. By using the duplicates()
, we can easily identify the duplicated columns so we can easily remove them and obtain a cleaned dataframe that is ready for analysis.
Data cleaning is an essential step in any data analysis project, and removing duplicate columns is just one of the many techniques that you can use to ensure that your data is accurate, consistent, and reliable. With the power of pandas and Python, you can quickly and efficiently clean your data and get started with your analysis.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.