How to Release Memory Used by a Pandas DataFrame
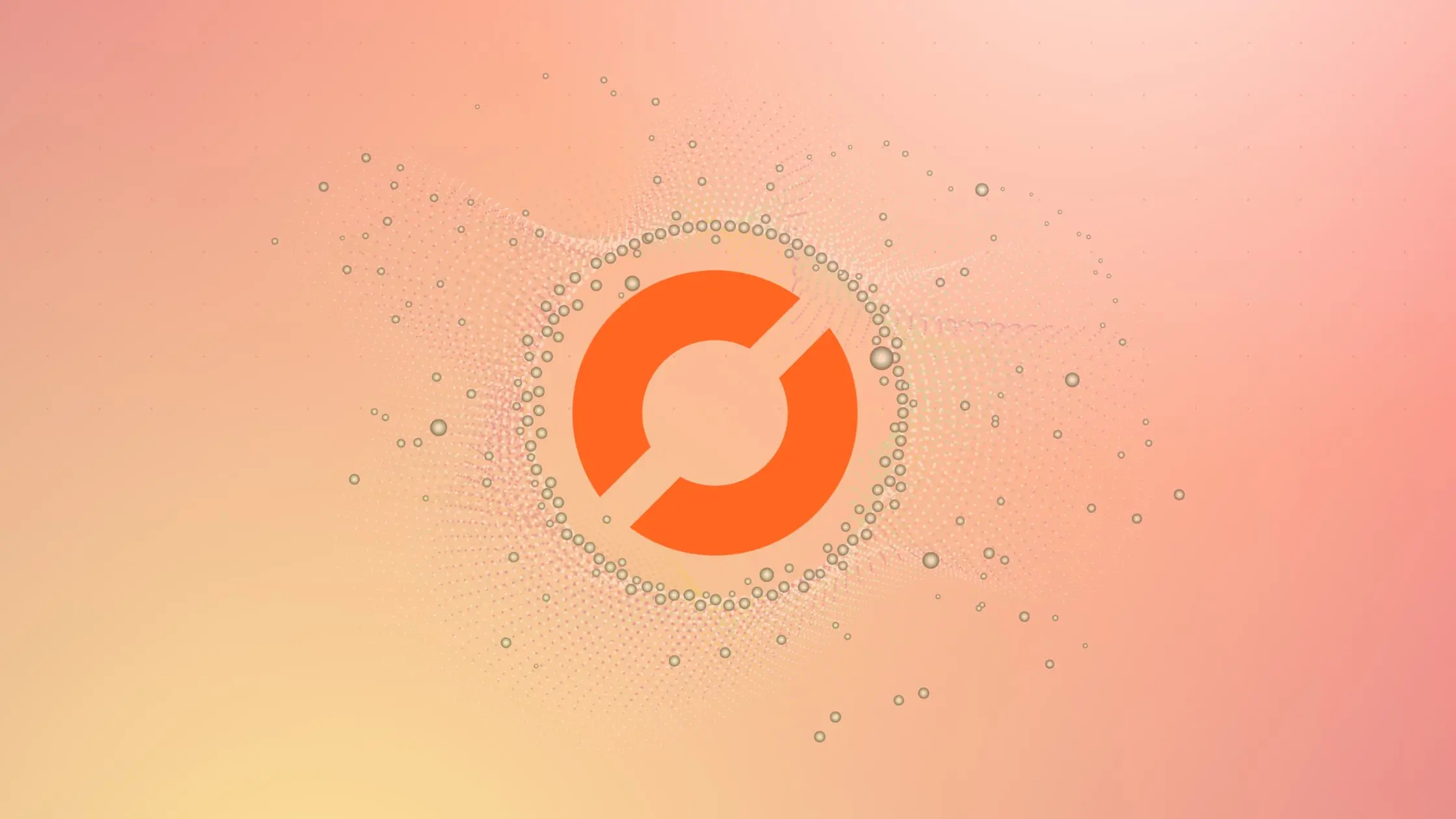
As a data scientist or software engineer, you may have encountered situations where you are working with large datasets in Pandas and have noticed that your computer’s memory usage is higher than expected. This can lead to slow performance and even crashes if your system runs out of memory. In this article, we will explore how to release memory used by a Pandas DataFrame, helping you to optimize your code and improve performance.
Table of Contents
- What is a Pandas DataFrame?
- How Pandas Handles Memory
- How to Release Memory Used by a Pandas DataFrame
- Pros and Cons Comparison
- Conclusion
What is a Pandas DataFrame?
Before we dive into the specifics of how to release memory in Pandas, let’s first define what a Pandas DataFrame is. In Pandas, a DataFrame is a two-dimensional labeled data structure with columns of potentially different types. You can think of it like a spreadsheet or SQL table, where each column represents a feature or variable and each row represents an observation.
How Pandas Handles Memory
Pandas is a powerful library for data manipulation, but it can consume a lot of memory, especially when working with large datasets. This is because Pandas stores data in memory as a contiguous block of memory, making it faster to access and manipulate. However, this also means that Pandas needs to allocate enough memory to store the entire DataFrame, even if you are only working with a subset of the data.
How to Release Memory Used by a Pandas DataFrame
There are several ways to release memory used by a Pandas DataFrame, depending on your specific use case and the size of your data. Here are some of the most common methods:
a. Using del
Statement
One simple way to release memory used by a Pandas DataFrame is to use the del
statement to delete the DataFrame object. This will remove the DataFrame from memory and free up the memory it was using.
# Example
import pandas as pd
df = pd.DataFrame({'A': range(1000), 'B': range(1000)})
# Release memory using del
del df
b. Garbage Collection (gc
Module)
Another way to release memory used by a Pandas DataFrame is to use the gc
module to manually trigger garbage collection. Garbage collection is the process of freeing memory that is no longer being used by the program. By manually triggering garbage collection, you can release memory that is no longer needed.
# Example
import gc
import pandas as pd
df = pd.DataFrame({'A': range(1000), 'B': range(1000)})
# Release memory using gc
gc.collect()
c. Dropping Unused Columns
Removing columns that are not needed can significantly reduce memory usage. Use the drop
method to eliminate unnecessary columns.
# Example
import pandas as pd
df = pd.DataFrame({'A': range(1000), 'B': range(1000)})
# Drop unused columns
df.drop(columns=['B'], inplace=True)
d. Optimizing Data Types
If you have a DataFrame with columns of different data types, you can use the astype
method to convert the data to a more memory-efficient data type. For example, you can convert a column of integers to a column of unsigned integers, which will use less memory.
# Example
import pandas as pd
df = pd.DataFrame({'A': range(1000), 'B': range(1000)})
# Optimize data types
df['A'] = df['A'].astype('int32')
Pros and Cons Comparison
Method | Pros | Cons |
---|---|---|
del Statement | - Simple and straightforward | - May not work well with complex data structures |
Garbage Collection (gc ) | - Automatic and systematic memory cleanup | - Can have performance overhead |
Dropping Unused Columns | - Effective for reducing memory footprint | - Irreversible, may lose data |
Optimizing Data Types | - Efficient use of memory | - Potential data loss or precision issues |
Conclusion
Efficiently managing memory is crucial for optimal performance when working with large datasets in Pandas DataFrames. By understanding and implementing the methods discussed in this article, you can release memory effectively, ensuring your code runs smoothly even with extensive data.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.