How to Read Rows and Convert Float to Integer in Pandas
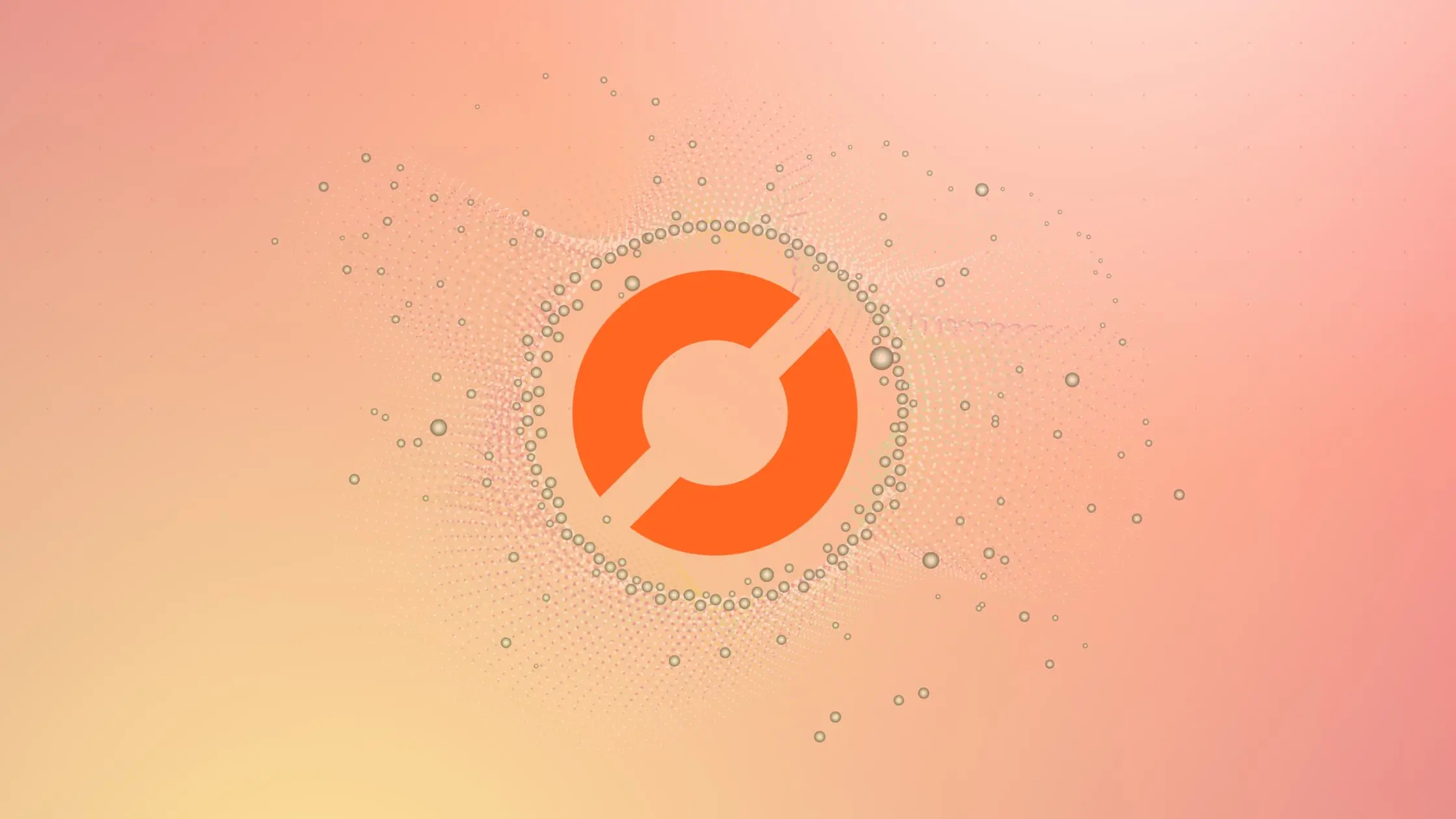
As a data scientist or software engineer, you have likely come across the need to read rows and convert float to integer in your data analysis and processing tasks. This can be a common task when working with datasets that contain numerical values, as it is often necessary to convert floating-point values to integers for various reasons, such as data cleaning, feature engineering, or data modeling.
In this article, we will explore how to read rows and convert float to integer in Pandas, a popular data manipulation library in Python. We will start by providing an overview of Pandas and its data structures, followed by a step-by-step guide on how to read rows and convert float to integer using Pandas.
Overview of Pandas
Pandas is a powerful and flexible open-source data manipulation library for Python. It provides data structures for efficiently storing and processing large datasets, as well as a wide range of functions and methods for data manipulation, cleaning, and analysis.
The two main data structures in Pandas are Series and DataFrame. A Series is a one-dimensional labeled array that can hold any data type, while a DataFrame is a two-dimensional labeled data structure with columns of potentially different data types.
Pandas provides numerous functions and methods for reading and writing data from various sources, including CSV, Excel, SQL databases, and JSON. It also allows for easy data cleaning, feature engineering, and data modeling, making it an essential tool for any data scientist or software engineer.
Reading Rows in Pandas
Before we can convert float to integer in Pandas, we need to first read in our data. Pandas provides several functions for reading data, with the most common being read_csv()
, which allows us to read in data from a CSV file.
CSV example:
Column1,Column2,Column3
1.5,2.7,3.0
4.2,5.1,6.8
7.3,8.0,9.5
import pandas as pd
# read CSV file
df = pd.read_csv('data.csv')
# print first few rows
print(df.head())
Output:
Column1 Column2 Column3
0 1.5 2.7 3.0
1 4.2 5.1 6.8
2 7.3 8.0 9.5
The read_csv()
function returns a DataFrame object containing the data from the CSV file. The head()
method allows us to print the first few rows of the DataFrame, giving us a glimpse of the data.
Converting Float to Integer in Pandas
Once we have read in our data, we can start converting float to integer using Pandas. The most common way to convert a float to an integer in Pandas is to use the astype()
method, which allows us to cast a column to a different data type.
# convert float to integer
df['Column1'] = df['Column1'].astype(int)
# print data types
print(df.dtypes)
Output:
Column1 int64
Column2 float64
Column3 float64
dtype: object
In the above example, we converted the values in Column1
from float to integer using the astype()
method. We then printed the data types of all columns in the DataFrame using the dtypes
attribute, which shows us that Column1
is now an integer.
Handling Missing Values
One thing to keep in mind when converting float to integer in Pandas is that any missing values (NaN) in the column will cause the conversion to fail. This is because NaN is not a valid integer value.
To handle missing values in Pandas, we can use the fillna()
method to replace NaN values with a valid value before converting the column to an integer.
CSV Example:
Column1,Column2,Column3
1.5,2.7,3.0
,5.1,6.8
7.3,8.0,9.5
# fill missing values with 0
df['Column1'] = df['Column1'].fillna(0).astype(int)
# print data types
print(df.dtypes)
Output:
Column1 int64
Column2 float64
Column3 float64
dtype: object
In the above example, we used the fillna()
method to replace any missing values in Column1
with 0 before converting the column to an integer. This allows us to avoid any errors caused by missing values and ensure that all values in the column are valid integers.
Conclusion
In this article, we explored how to read rows and convert float to integer in Pandas. We started by providing an overview of Pandas and its data structures, followed by a step-by-step guide on how to read rows and convert float to integer using Pandas.
We also discussed how to handle missing values when converting float to integer and showed how to use the fillna()
method to replace missing values with a valid value.
By following these steps, you should now be able to read rows and convert float to integer in Pandas with ease, making it easier to manipulate and analyze numerical data in your data science or software engineering projects.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.