How to Read Data dat file with Pandas
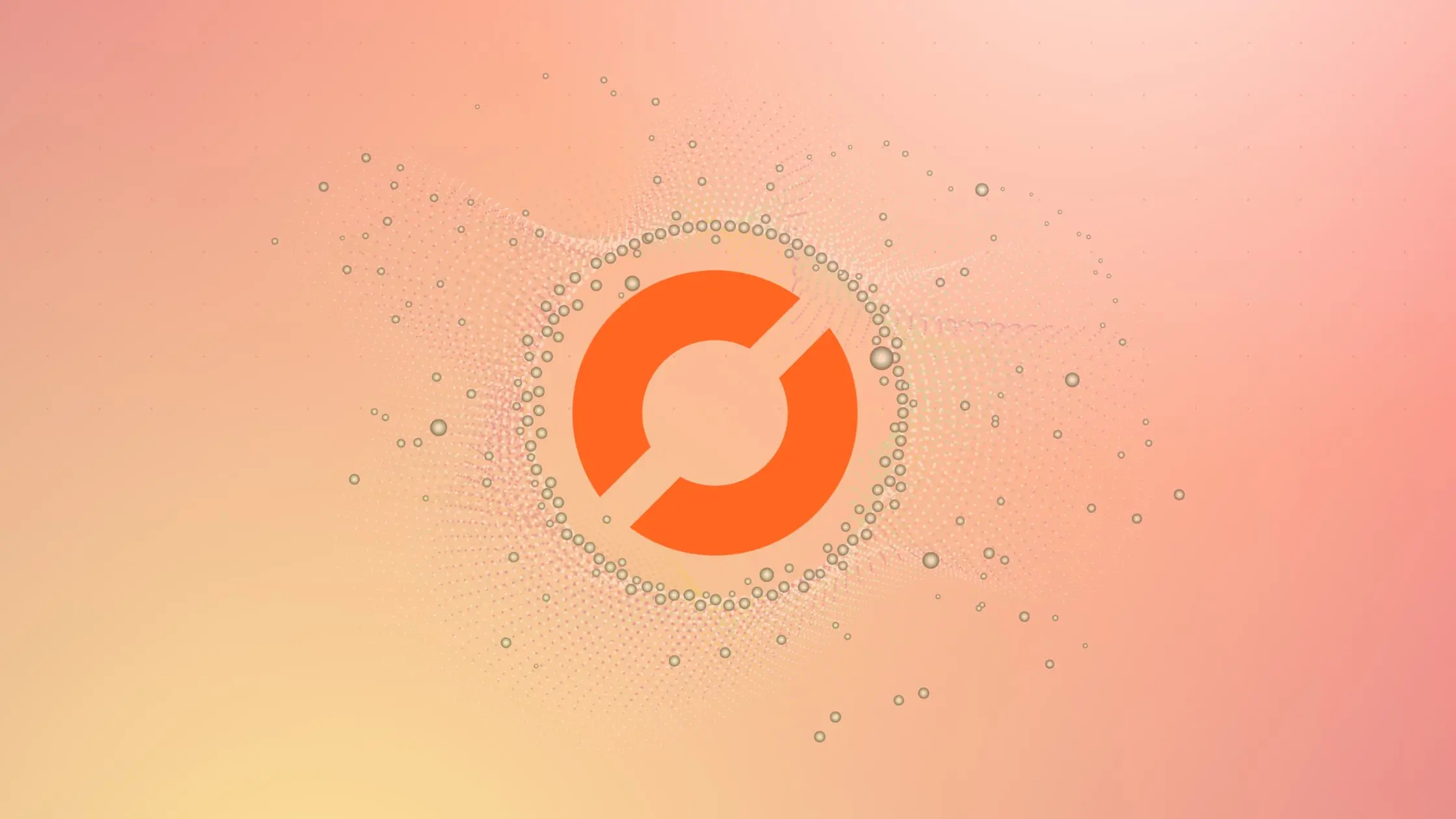
As a data scientist or software engineer, reading data from various file formats is an essential skill. One of the common file formats in data analysis and machine learning is the .dat file format. In this article, we will explore how to read data from .dat files using Pandas, a popular data analysis library in Python.
What is a .dat file?
A .dat file is a generic file format that stores data in binary format. It can contain any type of data, ranging from text to images and audio. The .dat file format is not standardized, and the data encoding format can vary depending on the application that created the file.
Why use Pandas to read .dat files?
Pandas is a popular Python library for data analysis and manipulation. It provides a powerful set of tools to read, manipulate, and analyze data in various formats, including .dat files. Pandas can read data from .dat files efficiently and convert them into a structured form that is easy to analyze and visualize.
How to read data from a .dat file using Pandas
The process of reading data from a .dat file using Pandas is straightforward. We need to use the read_csv
function provided by Pandas, which can read various file formats, including .dat files. Here are the steps to read a .dat file using Pandas:
- Import the Pandas library.
import pandas as pd
- Use the
read_csv
function to read the .dat file. We need to specify the file path and the delimiter used in the file.
df = pd.read_csv('path/to/file.dat', delimiter='\t')
In this example, we assume that the .dat file is located in the same directory as the Python script. The delimiter
parameter specifies the delimiter used in the .dat file. In most cases, the delimiter used in .dat files is the tab character ('\t'). However, it can vary depending on the application that created the file.
- Inspect the data using Pandas functions.
# Print the first five rows of the data frame
print(df.head())
The head()
function prints the first five rows of the data frame. We can use other Pandas functions to explore and analyze the data further, such as describe()
and info()
.
Example: Reading data from a .dat file using Pandas
Let’s consider an example of reading data from a .dat file using Pandas. Suppose we have a .dat file named ‘data.dat’ that contains the following data:
1.0 2.0 3.0
4.0 5.0 6.0
7.0 8.0 9.0
Here are the steps to read the data using Pandas:
- Import the Pandas library.
import pandas as pd
- Use the
read_csv
function to read the .dat file.
df = pd.read_csv('data.dat', delimiter='\t')
- Inspect the data using Pandas functions.
# Print the first five rows of the data frame
print(df.head())
The output of the above code will be:
1.0 2.0 3.0
0 4.0 5.0 6.0
1 7.0 8.0 9.0
In this example, we read the data from the ‘data.dat’ file and converted it into a Pandas data frame. The head()
function printed the first five rows of the data frame, which matches the data in the .dat file.
Conclusion
Reading data from a .dat file using Pandas is a simple and efficient process. We can use the read_csv
function provided by Pandas to read .dat files and convert them into a structured form that is easy to analyze and visualize. By mastering this skill, we can efficiently analyze and manipulate data in various file formats, including .dat files.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.