How to Properly Copy a Pandas DataFrame into Another Variable: A Guide
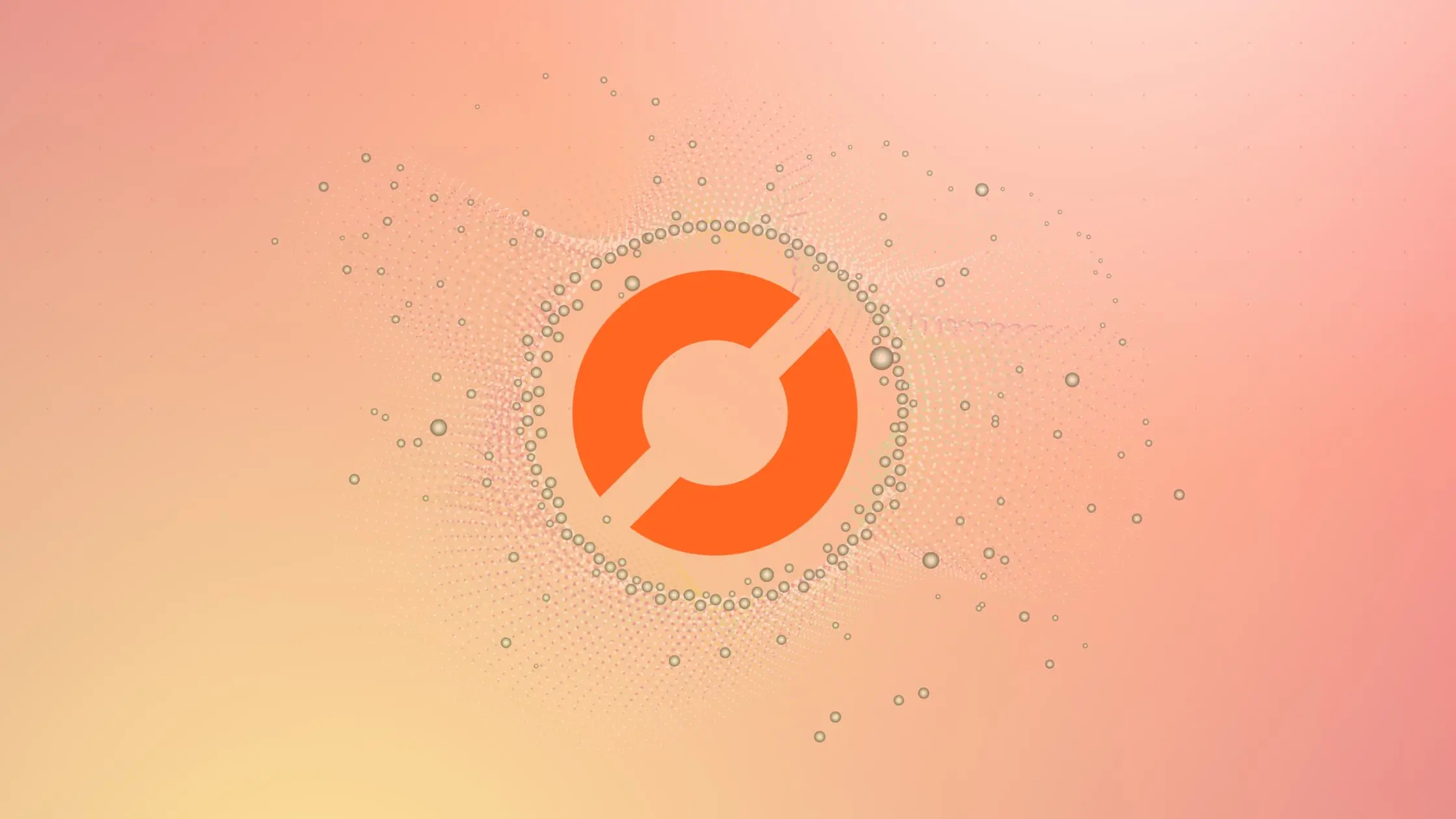
Data manipulation is a crucial part of any data scientist’s toolkit. One of the most common tasks is copying a pandas DataFrame into another variable. This might seem straightforward, but there are some nuances to consider. In this blog post, we’ll explore the correct ways to copy a pandas DataFrame, the pitfalls to avoid, and the reasons behind these best practices.
Understanding the Need for Copying DataFrames
Before we dive into the how, let’s understand the why. Copying a DataFrame is useful when you want to create a new DataFrame based on an existing one, but want to ensure that changes to the new DataFrame don’t affect the original. This is particularly important when working with large datasets, where accidental changes can be costly in terms of time and computational resources.
The Pitfalls of Improper Copying
A common mistake is to use the assignment operator (=
) to copy a DataFrame. For example:
df1 = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
df2 = df1
# Change a value in column A of df1
df1.loc[df1['A']==1, 'A'] = 10
print("\ndf1 after changing\n\n")
print(df1)
print("\ndf2 after changing df1\n")
print(df2)
Output:
df1 after changing
A B
0 10 4
1 2 5
2 3 6
df2 after changing df1
A B
0 10 4
1 2 5
2 3 6
This creates a new variable, df2
, that points to the same DataFrame as df1
. Any changes made to df2
will also affect df1
. This is known as shallow copying.
The Correct Way: Deep Copying
To create a true copy of the DataFrame, we need to use the copy()
method. This creates a new DataFrame that is a separate object from the original. Changes to the copied DataFrame will not affect the original. This is known as deep copying.
df1 = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
df2 = df1.copy()
# Change a value in column A of df1
df1.loc[df1['A']==1, 'A'] = 10
print("\ndf1 after changing\n\n")
print(df1)
print("\ndf2 after changing df1\n\n")
print(df2)
Output:
df1 after changing
A B
0 10 4
1 2 5
2 3 6
df2 after changing df1
A B
0 1 4
1 2 5
2 3 6
Now, any changes to df2
will not affect df1
.
Deep Copying with Parameters
The copy()
method also accepts parameters. The most commonly used parameter is deep
, which determines whether to make a deep copy (the default) or a shallow copy.
df1 = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
df2 = df1.copy(deep=True) # This is a deep copy
df3 = df1.copy(deep=False) # This is a shallow copy
In pandas, the copy()
method is used to create a copy of a DataFrame. The deep
parameter determines whether the copy is shallow or deep.
copy(deep=True)
: Creates a deep copy where the data and indices are copied, resulting in a completely independent DataFrame. Changes in the copied DataFrame do not affect the original.copy(deep=False)
: Produces a shallow copy, sharing the data and indices with the original DataFrame. Modifications to the copied DataFrame may impact the original one. It’s a more memory-efficient option but requires caution to avoid unintended side effects.
Conclusion
Copying pandas DataFrames correctly is crucial for data integrity and avoiding unexpected results. When working with Pandas DataFrames, it’s crucial to be mindful of how data is copied to avoid unintentional side effects. The copy() method provides a reliable way to create a new DataFrame with duplicated data, ensuring data integrity and preventing unexpected behavior.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.