How to Process Large Pandas DataFrames in Parallel
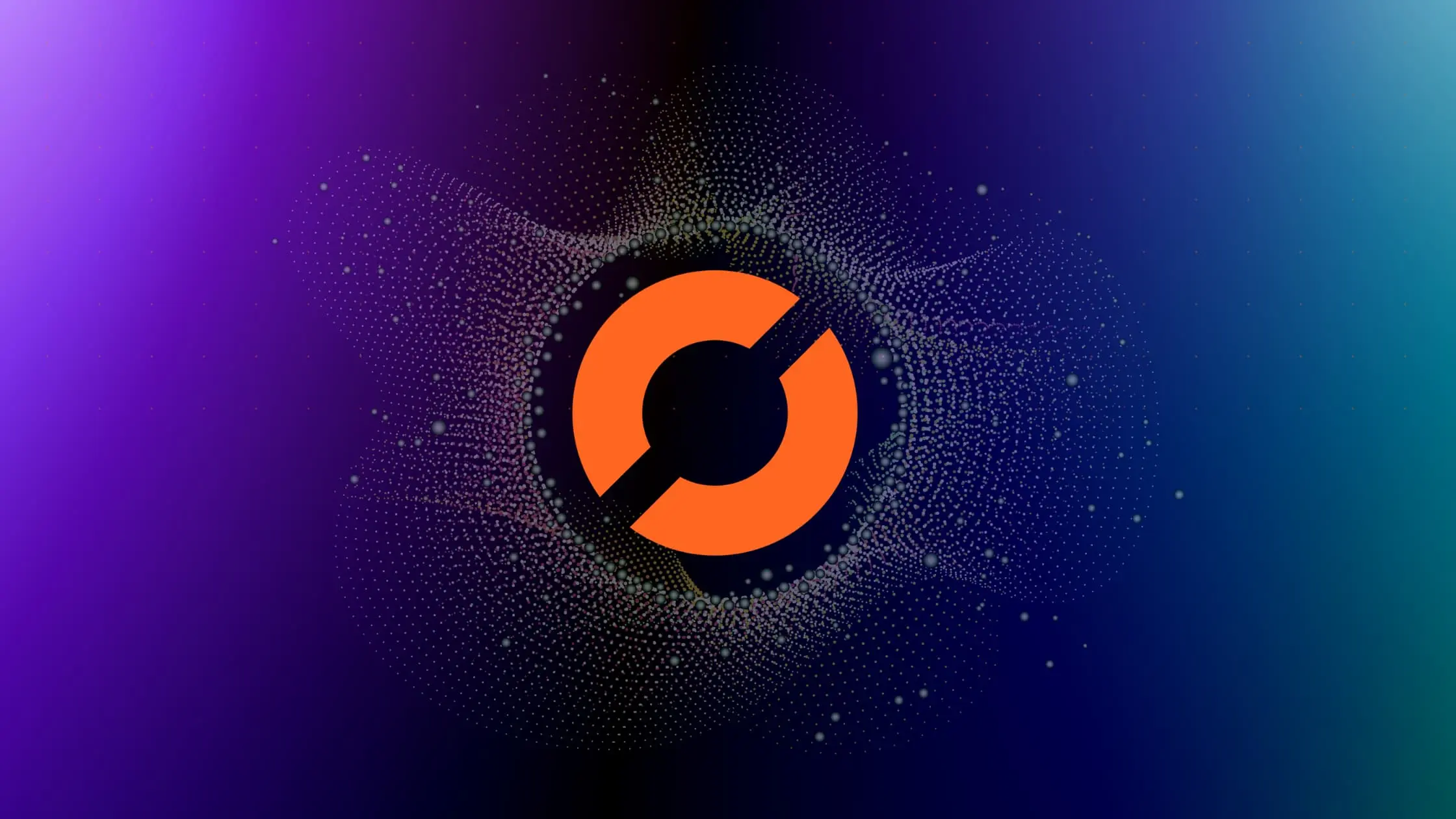
As a data scientist, you might have encountered a scenario where you need to process a large Pandas DataFrame. In such cases, the processing time can become a bottleneck, and you might need to optimize your code to make it faster. One of the ways to speed up the processing time is by parallelizing the code.
In this blog post, we will discuss how to process large Pandas DataFrames in parallel. We will cover the following topics:
Table of Contents
- Introduction to Parallel Processing
- Setting up the Environment
- Parallel Processing with Pandas
- Common Errors and How to Handle
- Conclusion
Introduction to Parallel Processing
Parallel processing involves dividing a task into smaller sub-tasks that can be executed simultaneously on multiple processing units. In the context of a Pandas DataFrame, parallel processing can be leveraged to break down the DataFrame into smaller chunks, processing them concurrently.
While parallel processing can significantly reduce the processing time of large DataFrames, it’s essential to note that not all tasks can be parallelized. Some tasks inherently require sequential processing, and attempting to parallelize them may lead to incorrect results.
Setting up the Environment
Before delving into parallel processing techniques, it’s crucial to set up the environment. This guide utilizes the following libraries:
- Pandas
- Dask
- Multiprocessing
You can install these libraries using pip.
pip install pandas dask
Parallel Processing with Pandas
Pandas provides various functionalities to process DataFrames in parallel. Let’s look at some of the ways to parallelize our code using Pandas.
Using the apply()
function
The apply()
function in Pandas can be used to apply a function to each row or column of a DataFrame. We can use the apply()
function along with the multiprocessing module to parallelize the code.
import pandas as pd
import multiprocessing
# Create a DataFrame
df = pd.DataFrame({'A': [1, 2, 3, 4, 5],
'B': [6, 7, 8, 9, 10]})
# Define a function to be applied to each row
def process_row(row):
# Process the row here
return row
# Set up multiprocessing
pool = multiprocessing.Pool()
# Apply the function to each row in parallel
result = pool.map(process_row, df.itertuples(index=False))
# Convert the result back to a DataFrame
result_df = pd.DataFrame(result, columns=df.columns)
# Close the multiprocessing pool
pool.close()
In the above code, we create a DataFrame and define a function to be applied to each row. We then set up a multiprocessing pool and use the map() function to apply the function to each row in parallel. Finally, we convert the result back to a DataFrame and close the multiprocessing pool.
Using the chunksize parameter
Another way to parallelize the code in Pandas is by using the chunksize parameter in the read_csv()
function. The chunksize parameter allows us to read a large DataFrame in chunks and process them in parallel.
import pandas as pd
import multiprocessing
# Set up multiprocessing
pool = multiprocessing.Pool()
# Read the large DataFrame in chunks
chunks = pd.read_csv('large_dataframe.csv', chunksize=100000)
# Define a function to be applied to each chunk
def process_chunk(chunk):
# Process the chunk here
return chunk
# Apply the function to each chunk in parallel
result = pool.map(process_chunk, chunks)
# Concatenate the result into a single DataFrame
result_df = pd.concat(result)
# Close the multiprocessing pool
pool.close()
In the above code, we set up a multiprocessing pool and use the read_csv()
function with the chunksize parameter to read the large DataFrame in chunks. We then define a function to be applied to each chunk and use the map() function to apply the function to each chunk in parallel. Finally, we concatenate the result into a single DataFrame and close the multiprocessing pool.
Using Dask
Dask is a parallel computing library that is built on top of Pandas. It provides scalable parallel processing for large DataFrames and arrays. Let’s see how we can use Dask to parallelize our code.
import dask.dataframe as dd
# Read the large DataFrame using Dask
df = dd.read_csv('large_dataframe.csv')
# Define a function to be applied to each partition
def process_partition(partition):
# Process the partition here
return partition
# Apply the function to each partition in parallel
result_df = df.map_partitions(process_partition).compute()
In the above code, we use the read_csv()
function from Dask to read the large DataFrame. We then define a function to be applied to each partition of the DataFrame and use the map_partitions() function to apply the function to each partition in parallel. Finally, we use the compute() function to compute the result.
Common Errors and How to Handle
When implementing parallel processing, common errors may arise. Here are some potential issues and ways to handle them:
Race Conditions: Ensure that shared resources are accessed in a thread-safe manner to avoid race conditions. Use appropriate locking mechanisms if necessary.
Memory Consumption: Large DataFrames processed in parallel may lead to increased memory consumption. Monitor memory usage and consider optimizing the code or using chunked processing to mitigate this issue.
Compatibility Issues: Verify compatibility between libraries and versions to prevent conflicts. Ensure that all libraries used in parallel processing are compatible with each other.
Task Dependency: Be mindful of task dependencies and ensure that parallelized tasks do not interfere with each other. Carefully design parallelized functions to avoid unexpected dependencies.
Error Handling: Implement robust error handling to gracefully manage exceptions. Log errors for debugging purposes and provide informative error messages.
Conclusion
In this blog post, we discussed how to process large Pandas DataFrames in parallel. We covered various techniques such as using the apply()
function, the chunksize parameter, and Dask. Parallel processing can significantly reduce the processing time of a large DataFrame. However, it is essential to note that not all tasks can be parallelized, and attempting to parallelize them can lead to incorrect results.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.