How to Perform a Pandas Join on Columns with Different Names
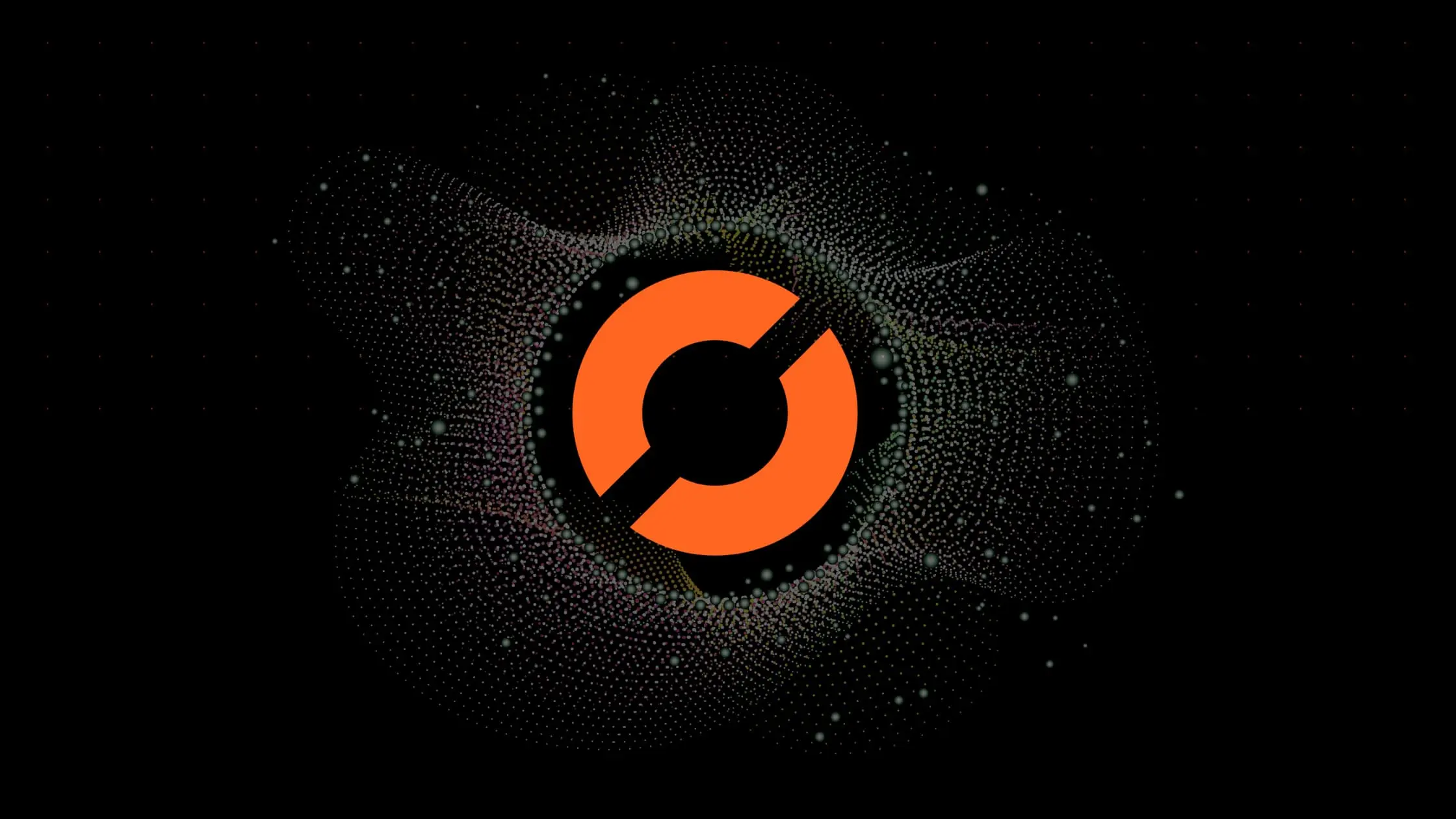
As a data scientist or software engineer, you are likely familiar with the Pandas library, which is a powerful tool for data manipulation and analysis. One of the most common tasks in data analysis is joining data from multiple sources, and Pandas provides several functions for this purpose, including merge()
and join()
. However, when trying to join data on columns with different names, things can become a bit more complicated. In this article, we will discuss how to perform a Pandas join on columns with different names.
What is a Pandas join?
A join is a way of combining data from two or more tables based on a common column or set of columns. In Pandas, a join operation is performed using the merge()
or join()
function. These functions take two or more DataFrames as input and return a new DataFrame that combines the data based on the specified columns.
The problem with joining on columns with different names
When joining data from multiple sources, it is common for the columns to have different names. For example, you may have one DataFrame with a column named “ID” and another DataFrame with a column named “CustomerID”. In order to join these two DataFrames, you need to specify which columns to use as the join keys.
By default, Pandas will try to join the DataFrames based on columns with the same name. If the columns have different names, you need to specify which columns to use for the join explicitly. This can be a bit confusing, especially if you are new to Pandas.
The solution: specifying the column names explicitly
To join two DataFrames on columns with different names, you need to specify which columns to use explicitly. This can be done using the left_on
and right_on
parameters of the merge()
or join()
function. The left_on
parameter specifies the column(s) to use from the left DataFrame, while the right_on
parameter specifies the column(s) to use from the right DataFrame.
For example, let’s say we have two DataFrames, df1
and df2
, with the following columns:
df1:
| ID | Name | Age |
|----|--------|-----|
| 1 | Alice | 25 |
| 2 | Bob | 30 |
| 3 | Charlie| 35 |
df2:
| CustomerID | City |
|------------|-----------|
| 1 | New York |
| 2 | San Diego |
| 3 | Chicago |
To join these two DataFrames on the ID
and CustomerID
columns, we can use the following code:
merged_df = pd.merge(df1, df2, left_on='ID', right_on='CustomerID')
This will create a new DataFrame, merged_df
, that contains the columns from both DataFrames, joined on the ID
and CustomerID
columns:
merged_df:
| ID | Name | Age | CustomerID | City |
|----|--------|-----|------------|-----------|
| 1 | Alice | 25 | 1 | New York |
| 2 | Bob | 30 | 2 | San Diego |
| 3 | Charlie| 35 | 3 | Chicago |
As you can see, the left_on
parameter specifies the ID
column from the left DataFrame (df1
), while the right_on
parameter specifies the CustomerID
column from the right DataFrame (df2
).
Conclusion
Performing a Pandas join on columns with different names is a common task in data analysis, and it can be a bit confusing if you are new to Pandas. However, by specifying the column names explicitly using the left_on
and right_on
parameters of the merge()
or join()
function, you can easily join DataFrames on columns with different names.
In summary, to perform a Pandas join on columns with different names:
- Identify the columns to use as the join keys.
- Use the
left_on
andright_on
parameters of themerge()
orjoin()
function to specify the columns to use from the left and right DataFrames, respectively. - Perform the join and create a new DataFrame that contains the joined data.
With this knowledge, you can confidently join data from multiple sources in Pandas, even when the columns have different names.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.