How to Parse XML with Python Pandas
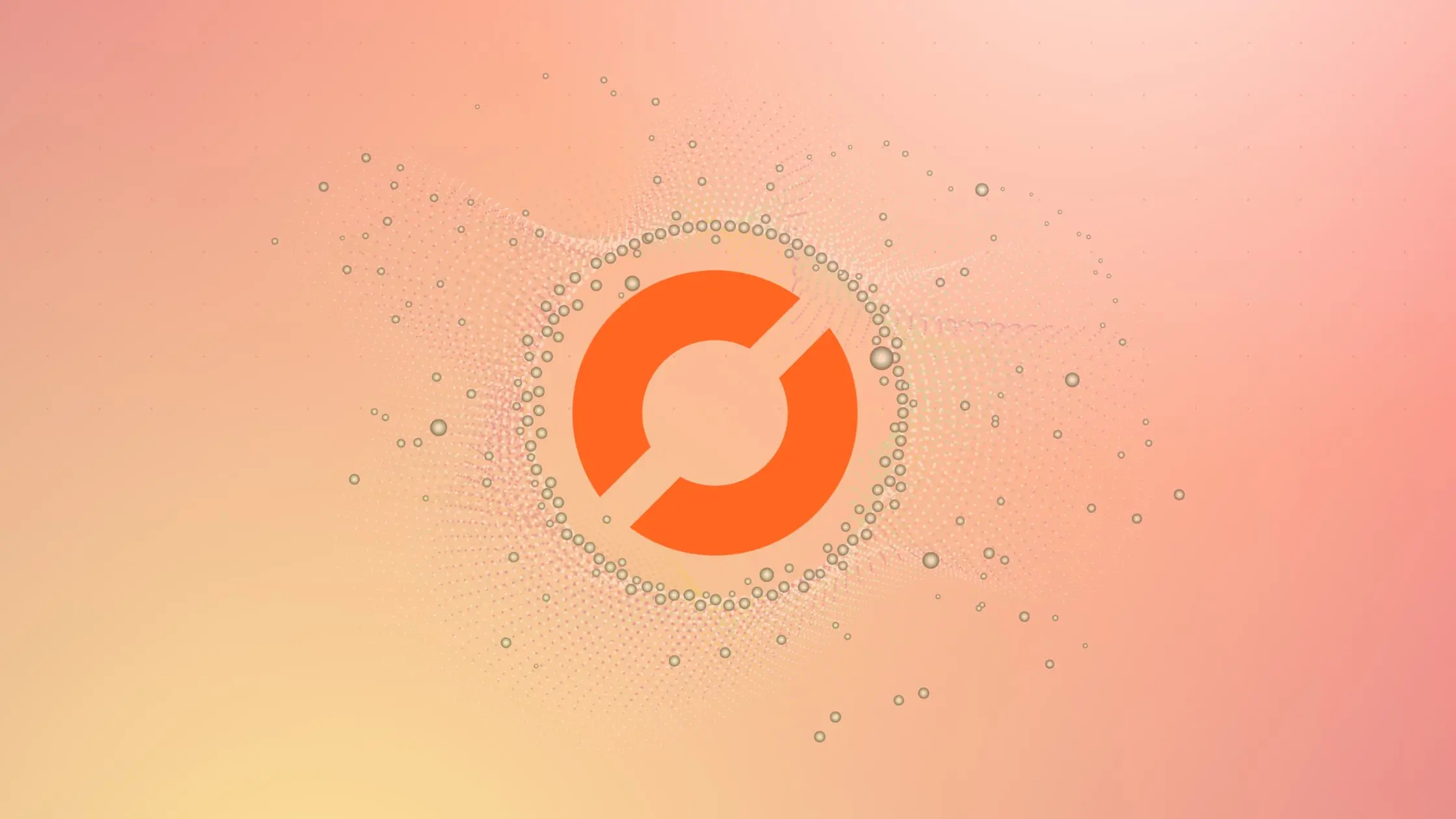
As a data scientist or software engineer, you may come across XML files in your work. XML (Extensible Markup Language) is a popular format for storing and exchanging data on the web. However, parsing XML files can be a tricky task, especially when you need to extract specific data from a large XML file. In this article, we will explore how to parse XML with Python Pandas and get a complete block of tags in one row.
What is XML Parsing?
XML parsing is the process of analyzing an XML document and extracting information from it. The process involves identifying the structure of the XML document, locating the tags and attributes, and extracting the data associated with them. XML parsing is commonly used in web development, data extraction, and data analysis.
Why Use Python Pandas for XML Parsing?
Python Pandas is a powerful data analysis library that provides tools for reading, writing, and manipulating data in various formats, including XML. Pandas provides a simple and efficient way to parse XML files and extract data from them. Using Pandas for XML parsing allows you to perform complex data analysis tasks with ease.
How to Parse XML with Python Pandas
Let’s consider the following xml file:
<?xml version="1.0" encoding="utf-8"?>
<root>
<person>
<name>
John Doe
</name>
<age>
30
</age>
</person>
</root>
Method 1: Using xml.etree.ElementTree
import xml.etree.ElementTree as ET
import pandas as pd
tree = ET.parse("sample.xml")
root = tree.getroot()
data = []
for person in root.findall("person"):
name = person.find("name").text
age = person.find("age").text
data.append({"Name": name, "Age": age})
df = pd.DataFrame(data)
print(df)
This method uses the xml.etree.ElementTree
module to parse XML and convert it to a Pandas DataFrame. It involves manually iterating through the XML structure and extracting data.
Output:
Name Age
0 John Doe 30
3. Method 2: Using xmltodict
Make sure that xmltodict
has been installed. If not, you can install it by running:
pip install xmltodict
import xmltodict
import pandas as pd
with open("sample.xml") as xml_file:
data_dict = xmltodict.parse(xml_file.read())
data = []
# Check if there is more than one person in the XML
persons = data_dict.get("root", {}).get("person", [])
if not isinstance(persons, list):
persons = [persons] # If only one person, convert it to a list for consistency
for person in persons:
name = person.get("name")
age = person.get("age")
data.append({"Name": name, "Age": age})
df = pd.DataFrame(data)
print(df)
This method leverages the xmltodict
library, which simplifies the XML parsing process by converting XML to a Python dictionary. It checks whether there is more than one person in the XML. If there is only one person, it converts it to a list for consistency. Additionally, it uses the get method to safely retrieve values from the dictionary.
Output:
Name Age
0 John Doe 30
Common Errors and Solutions
Error 1: Malformed XML
Description: The XML file has syntax errors.
Solution: Validate XML syntax using online tools or an XML validator. Correct the errors in the XML file.
Error 2: Missing Tags
Description: Some XML tags or attributes are missing.
Solution: Check for missing tags or attributes in the XML file. Update the file to include the necessary elements.
Error 3: Namespace Issues
Description: XML file contains namespaces that are not handled properly.
Solution: Use namespace-aware parsing libraries or remove namespaces from the XML file if not needed.
Conclusion
Parsing XML with Python Pandas opens up opportunities for efficiently handling structured data. Whether you choose the simplicity of xml.etree.ElementTree
or the convenience of xmltodict
, understanding the methods, best practices, and potential pitfalls is crucial for successful XML parsing. Choose the method that best suits your data structure and complexity, keeping in mind the trade-offs between simplicity and flexibility. By following best practices and addressing common errors, you can streamline the XML parsing process and enhance the reliability of your data analysis workflows.
To parse XML with Python Pandas, you will need to install the lxml library. Lxml is a Python library that provides a fast and efficient way to parse XML files. You can install lxml using pip, the Python package manager.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.