How to Normalize Image Dataset Using PyTorch
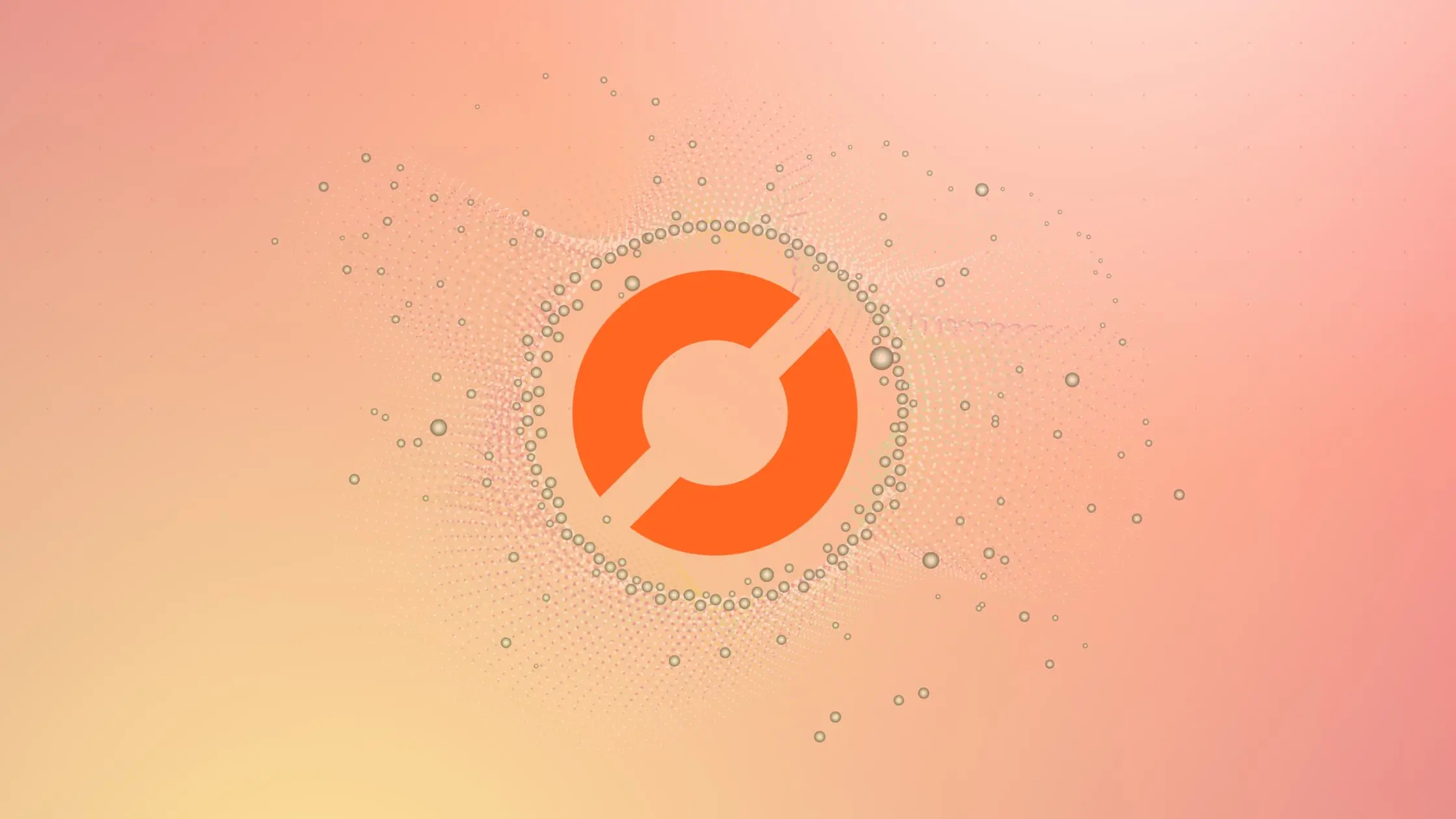
As a data scientist or software engineer, you might be working with image datasets that need to be normalized before they can be used for machine learning tasks. Normalizing the data ensures that the model receives consistent input, making it easier to train and improve its accuracy. In this article, we will explore how to normalize image datasets using PyTorch.
Table of Contents
- What is Image Normalization?
- Why Normalize Image Data?
- How to Normalize Image Data using PyTorch
- Common Errors in Image Data Normalization using PyTorch
- Conclusion
What is Image Normalization?
Image normalization is the process of adjusting the pixel values of an image to make it easier for a machine learning model to learn from it. This is done by scaling the pixel values to a common range or by subtracting the mean and dividing by the standard deviation. Normalizing the data ensures that the model receives consistent input, making it easier to train and improve its accuracy.
Why Normalize Image Data?
There are several reasons why we should normalize image data. Firstly, normalization helps to eliminate differences in brightness and contrast across different images, which can be caused by variations in lighting conditions, camera settings, and other factors. Secondly, normalizing the data helps to reduce the impact of outliers, which can skew the distribution of the data and make it harder for the model to learn from it. Finally, normalization can help to speed up the training process, as the model can converge more quickly when the input data is consistent and predictable.
How to Normalize Image Data using PyTorch
PyTorch is a popular deep learning framework that provides a wide range of tools for working with image datasets. One of the most common ways to normalize image data in PyTorch is by using the transforms.Normalize
function. This function takes two arguments: the mean and standard deviation of the dataset.
Step 1: Load the Image Dataset
The first step in normalizing an image dataset is to load the dataset into PyTorch. This can be done using the torchvision.datasets.ImageFolder
function, which automatically loads all the images in a folder and assigns them to their respective classes based on their folder names.
import torchvision.datasets as datasets
data_dir = "/path/to/dataset"
dataset = datasets.ImageFolder(data_dir)
Step 2: Calculate the Mean and Standard Deviation of the Dataset
The next step is to calculate the mean and standard deviation of the dataset. This can be done using the torch.utils.data.DataLoader
function and a custom function to calculate the mean and standard deviation of the dataset.
from torchvision import transforms
def get_mean_std(loader):
# Compute the mean and standard deviation of all pixels in the dataset
num_pixels = 0
mean = 0.0
std = 0.0
for images, _ in loader:
batch_size, num_channels, height, width = images.shape
num_pixels += batch_size * height * width
mean += images.mean(axis=(0, 2, 3)).sum()
std += images.std(axis=(0, 2, 3)).sum()
mean /= num_pixels
std /= num_pixels
return mean, std
data_transforms = transforms.Compose([
transforms.Resize((224, 224)),
transforms.ToTensor(),
])
batch_size = 32
loader = torch.utils.data.DataLoader(dataset, batch_size=batch_size, shuffle=True)
mean, std = get_mean_std(loader)
Step 3: Normalize the Dataset
Once the mean and standard deviation of the dataset have been calculated, they can be used to normalize the dataset using the transforms.Normalize
function.
data_transforms = transforms.Compose([
transforms.Resize((224, 224)),
transforms.ToTensor(),
transforms.Normalize(mean=mean, std=std)
])
dataset = datasets.ImageFolder(data_dir, transform=data_transforms)
Step 4: Train the Model
Once the image dataset has been normalized, it can be used to train a machine learning model. This can be done using PyTorch’s built-in functions for defining and training neural networks.
import torch.nn as nn
import torch.optim as optim
model = nn.Sequential(
nn.Conv2d(3, 32, kernel_size=3, stride=1, padding=1),
nn.ReLU(),
nn.MaxPool2d(kernel_size=2, stride=2),
nn.Conv2d(32, 64, kernel_size=3, stride=1, padding=1),
nn.ReLU(),
nn.MaxPool2d(kernel_size=2, stride=2),
nn.Flatten(),
nn.Linear(64 * 56 * 56, 128),
nn.ReLU(),
nn.Linear(128, 10)
)
criterion = nn.CrossEntropyLoss()
optimizer = optim.SGD(model.parameters(), lr=0.001, momentum=0.9)
epochs = 10
for epoch in range(epochs):
running_loss = 0.0
for i, (inputs, labels) in enumerate(loader):
optimizer.zero_grad()
outputs = model(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
running_loss += loss.item() * inputs.size(0)
epoch_loss = running_loss / len(dataset)
print(f"Epoch {epoch+1} loss: {epoch_loss:.4f}")
Common Errors in Image Data Normalization using PyTorch
Shape Mismatch during Mean and Standard Deviation Calculation
When calculating the mean and standard deviation of the dataset, it’s crucial to ensure that the shape of the images is compatible with the expected shape in the loader. A common error is overlooking the shape of the images in the loader and failing to adjust the calculations accordingly.
# Incorrect Calculation
batch_size, num_channels, height, width = images.shape
# Corrected Calculation
batch_size, num_channels, height, width = images.shape[0], images.shape[1], images.shape[2], images.shape[3]
Inconsistent Dataset Dimensions in Model Training
After normalization, it’s essential to confirm that the dimensions of the images align with the model’s input expectations. Failure to ensure consistency between the model input dimensions and the normalized dataset can result in runtime errors.
# Ensure the model input dimensions match the normalized image dimensions
model = nn.Sequential(
# ... existing layers ...
nn.Linear(64 * 56 * 56, 128), # Ensure this matches the flattened image dimensions
# ... remaining layers ...
)
Incorrect Channel Order in Normalization
The order of channels in the mean and standard deviation calculation must match the order of channels in the dataset. A mistake in the channel order can lead to inaccurate normalization.
# Incorrect Channel Order
transforms.Normalize(mean=mean, std=std)
# Corrected Channel Order
transforms.Normalize(mean=[mean[0], mean[1], mean[2]], std=[std[0], std[1], std[2]])
Incorrect Folder Structure for Image Dataset
Make sure the image dataset folder structure is set up correctly, and the path provided in data_dir
accurately points to the root of the dataset.
# Ensure the dataset folder structure is correct
data_dir = "/path/to/dataset" # Verify the correct path to the dataset
dataset = datasets.ImageFolder(data_dir)
Conclusion
Normalizing image datasets is an important preprocessing step in machine learning tasks that involve image data. PyTorch provides a convenient and flexible way to normalize image datasets using the transforms.Normalize
function. By following the steps outlined in this article, you can ensure that your machine learning models receive consistent input, making it easier to train and improve their accuracy.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.