How to Merge Two DataFrame Columns into One in Pandas
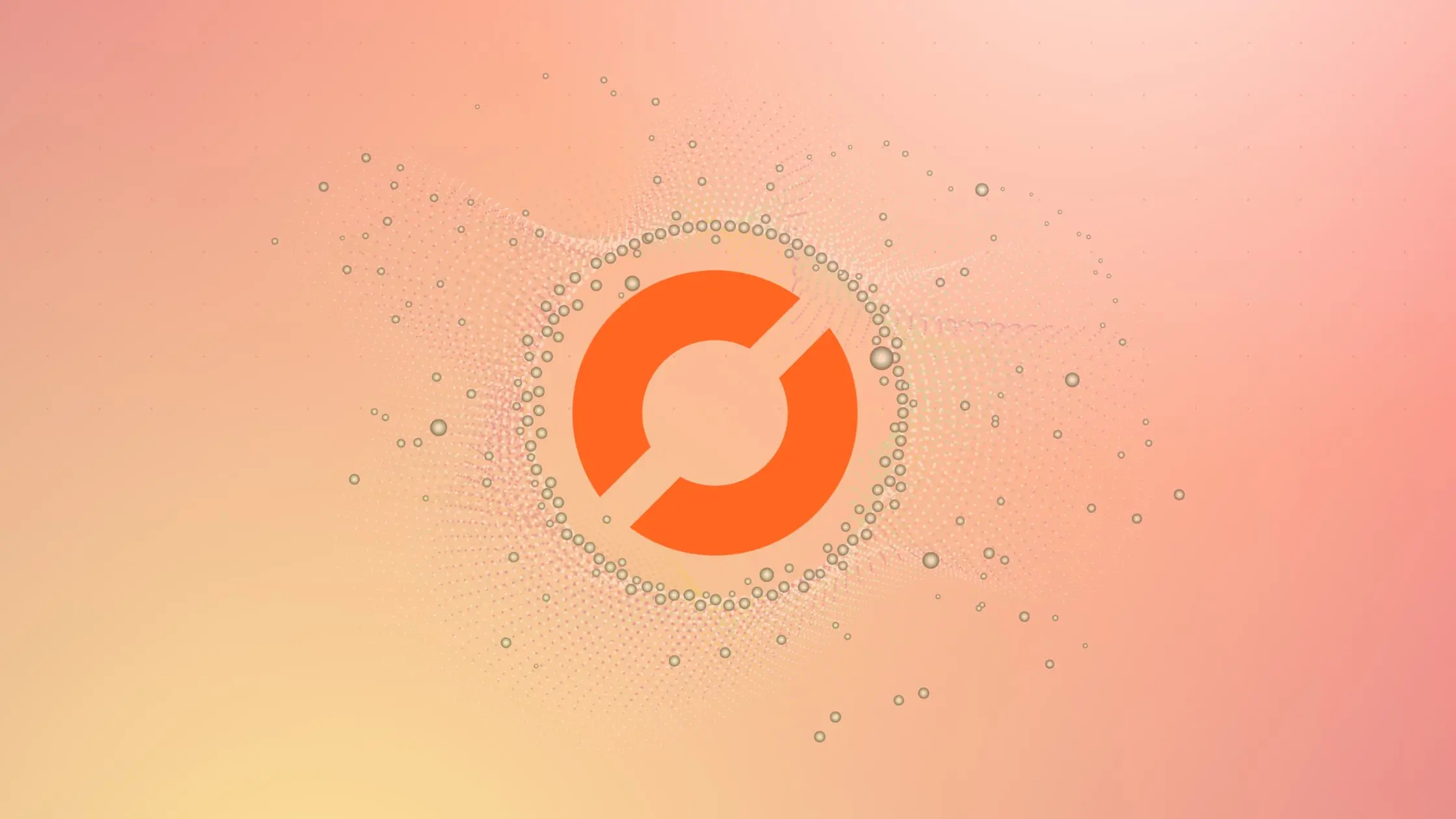
What is Pandas?
Pandas is a Python library that provides data structures for efficiently storing and manipulating large datasets. The two most important data structures in Pandas are the Series and DataFrame. A Series is a one-dimensional array-like object that can hold any data type, while a DataFrame is a two-dimensional table-like data structure that consists of rows and columns.
Pandas offers a wide range of functions and methods for manipulating data, including filtering, sorting, grouping, merging, and reshaping. One of the most powerful features of Pandas is its ability to handle missing data, which is a common problem in real-world datasets.
How to Merge Two Columns into One in Pandas
Merging two columns into one is a common task when working with tabular data. In Pandas, this can be done using the concat()
function or the join()
method. The concat()
function is used to concatenate two or more DataFrames or Series along a particular axis, while the join()
method is used to join two or more DataFrames based on a common index or column.
In this blog post, we will focus on using the concat()
function to merge two columns into one. The concat()
function is straightforward to use and can be applied to both Series and DataFrames.
Merging Two Columns of a DataFrame
Suppose we have a DataFrame df
with two columns, col1
and col2
, and we want to merge these two columns into a single column. We can do this using the concat()
function as follows:
import pandas as pd
# create a sample DataFrame
df = pd.DataFrame({'col1': [1, 2, 3], 'col2': [4, 5, 6]})
# merge the two columns into one
df['merged'] = pd.concat([df['col1'], df['col2']])
In this example, we first create a sample DataFrame df
with two columns, col1
and col2
. We then use the concat()
function to concatenate the two columns along the default axis (axis=0). Finally, we assign the concatenated Series to a new column merged
in the original DataFrame df
.
Merging Two Columns of a Series
Merging two columns of a Series is similar to merging two columns of a DataFrame. The only difference is that we need to create a DataFrame with the two columns first and then merge them into a single Series using the concat()
function.
import pandas as pd
# create a sample Series
s1 = pd.Series([1, 2, 3])
s2 = pd.Series([4, 5, 6])
# create a DataFrame with the two columns
df = pd.concat([s1, s2], axis=1)
# merge the two columns into one
s_merged = pd.concat([s1, s2])
In this example, we first create two sample Series s1
and s2
. We then use the concat()
function to concatenate the two Series along the default axis (axis=0) and assign the concatenated Series to a new variable s_merged
.
Conclusion
Merging two columns into one is a common task when working with tabular data, and Pandas provides several functions and methods to accomplish this task. In this blog post, we explored how to merge two DataFrame columns into one using the concat()
function. We also showed how to merge two columns of a Series by first creating a DataFrame with the two columns and then merging them using the concat()
function. With these techniques, you can easily merge two or more columns into a single column in Pandas.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.