How to Merge Two CSVs Using Pandas in Python
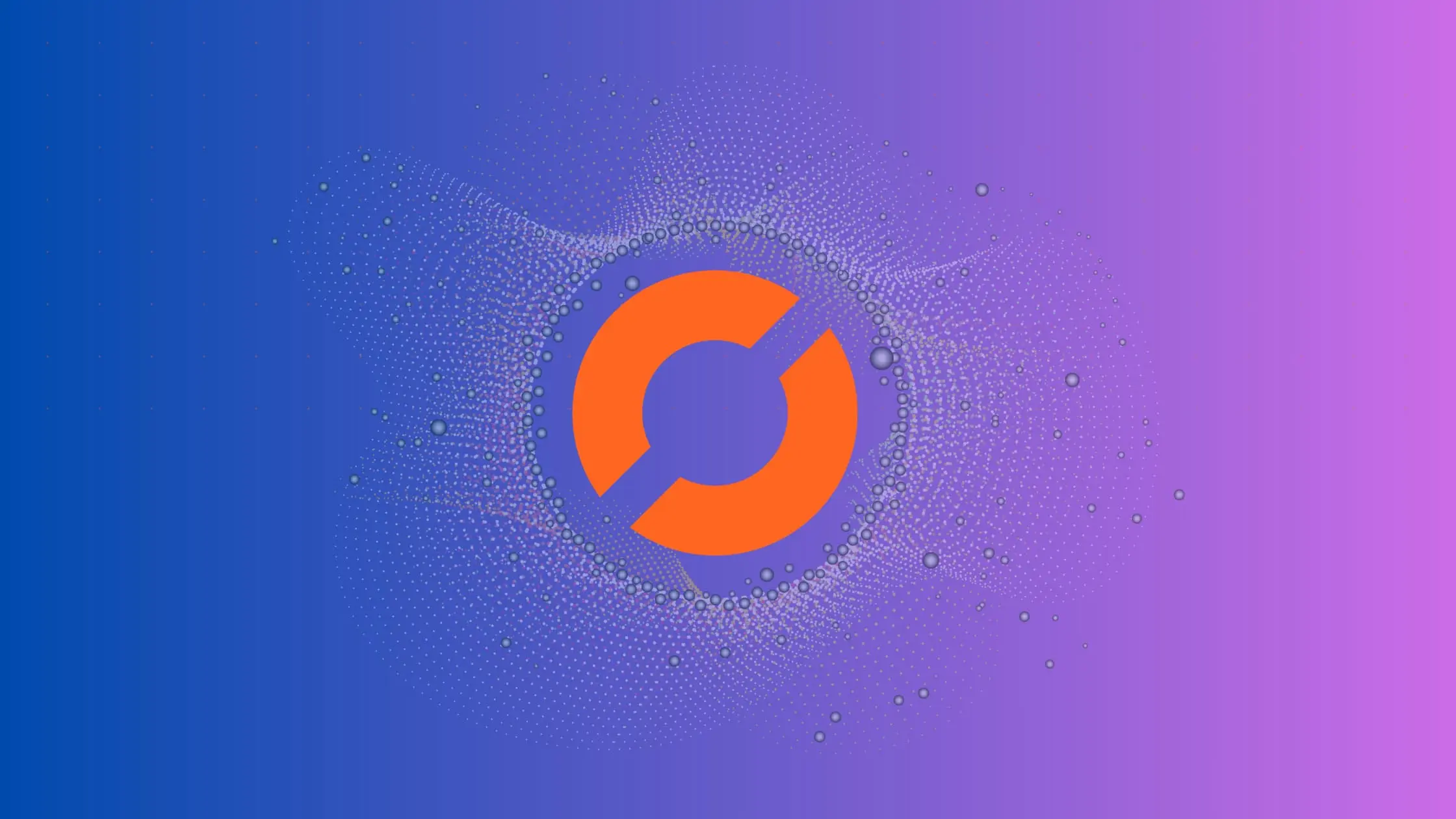
How to Merge Two CSVs Using Pandas in Python
As a data scientist or software engineer, you would often find yourself working with large sets of data. In many cases, you might have to combine or merge data from multiple sources. This is where the power of pandas in Python comes in handy. In this post, we will explore how to merge two CSV files using pandas in Python.
What is Pandas?
Pandas is a powerful Python library for data manipulation and analysis. It provides data structures for efficiently storing and manipulating large datasets. Pandas is built on top of numpy and provides an easy-to-use interface for data manipulation.
Pandas provides two main classes for storing data: Series and DataFrame. A Series is a one-dimensional data structure, while a DataFrame is a two-dimensional data structure. A DataFrame is similar to a spreadsheet or a SQL table, with rows and columns.
What is CSV?
CSV stands for Comma Separated Values. It is a file format for storing tabular data in plain text. Each line in a CSV file represents a row, and the values in each row are separated by commas. CSV files are widely used for data exchange between different software applications.
Merging Two CSV Files Using Pandas
Merging two CSV files using pandas is a straightforward process. The first step is to read the CSV files into pandas dataframes. We can do this using the read_csv()
function in pandas. Let’s assume we have two CSV files: file1.csv
and file2.csv
.
import pandas as pd
# Read CSV files into dataframes
df1 = pd.read_csv('file1.csv')
df2 = pd.read_csv('file2.csv')
Once we have the two dataframes, we can merge them using the merge()
function in pandas. The merge()
function takes two dataframes and a set of merge keys as inputs.
# Merge dataframes
merged_df = pd.merge(df1, df2, on='merge_key')
In the above code, merge_key
is the column in both dataframes that we want to merge on. The merge()
function combines the rows in the two dataframes that have the same value in the merge_key
column. By default, the merge()
function performs an inner join, which means that only the rows that have a match in both dataframes are included in the merged dataframe.
We can also specify the type of join we want to perform using the how
parameter. The possible values for how
are inner
, outer
, left
, and right
. An inner join is the default, but we can use the other options to perform different types of joins.
# Merge dataframes using left join
merged_df = pd.merge(df1, df2, on='merge_key', how='left')
In the above code, we are using a left join, which means that all the rows in the left dataframe (df1
) are included in the merged dataframe, and the rows in the right dataframe (df2
) are included only if they have a match in the left dataframe.
We can also specify the columns we want to include in the merged dataframe using the suffixes
parameter. By default, if two columns have the same name in both dataframes, pandas will add a suffix to distinguish them (_x
for the left dataframe and _y
for the right dataframe). We can specify our own suffixes using the suffixes
parameter.
# Merge dataframes with custom suffixes
merged_df = pd.merge(df1, df2, on='merge_key', suffixes=('_left', '_right'))
In the above code, we are using custom suffixes (_left
and _right
) to distinguish the columns in the left and right dataframes.
Conclusion
In this post, we explored how to merge two CSV files using pandas in Python. Pandas provides a simple and efficient way to combine data from multiple sources. We can use the merge()
function in pandas to perform different types of joins and specify the columns we want to include in the merged dataframe. With pandas, data manipulation and analysis become much easier and more efficient.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.