How to Load PyTorch Dataloader into GPU
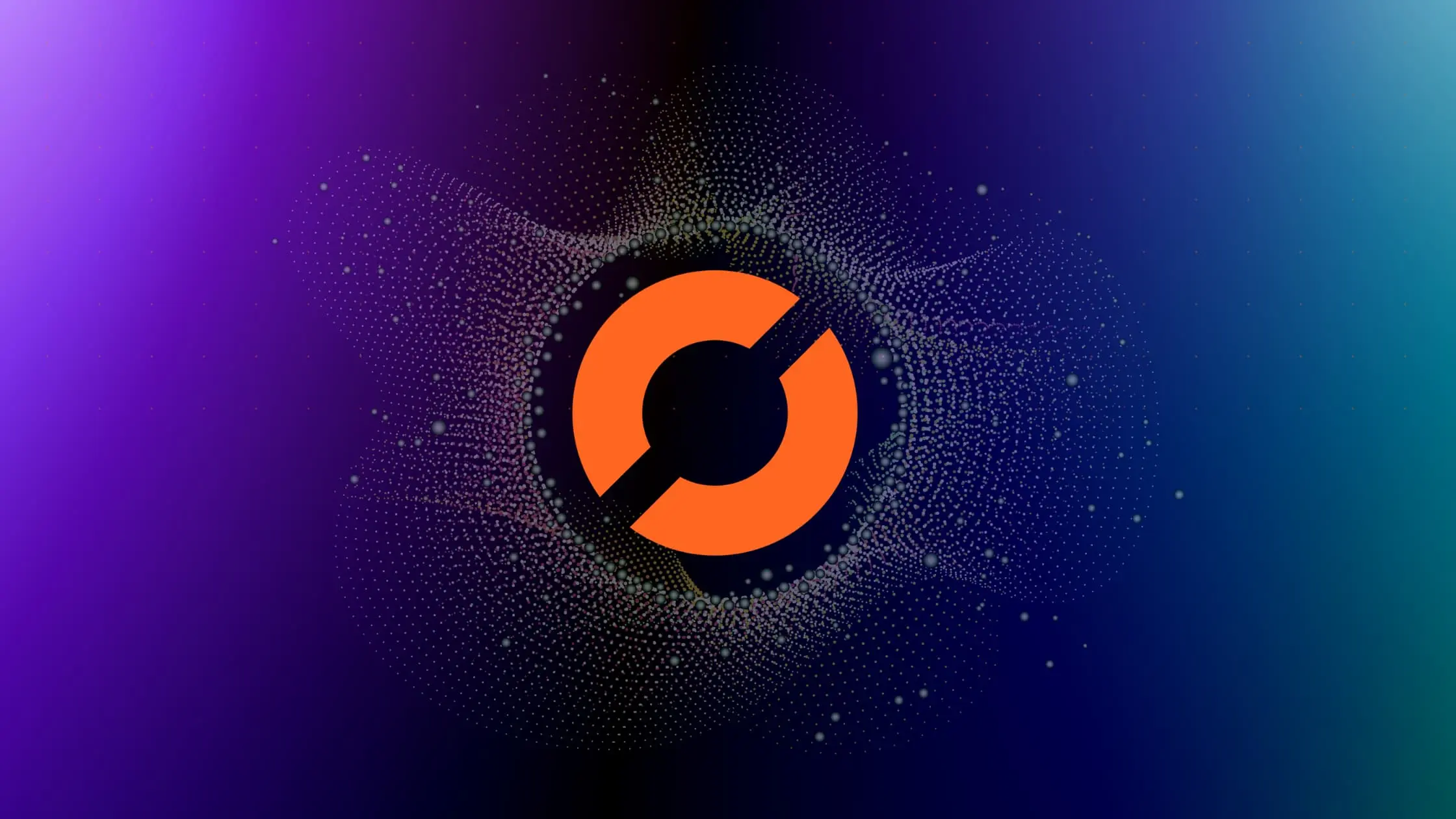
As a data scientist or software engineer, you might have encountered the challenge of processing large datasets in PyTorch. The PyTorch DataLoader is a powerful tool that enables efficient data loading and processing. However, loading the data into the GPU can be a bottleneck, especially when dealing with large datasets.
In this tutorial, we will guide you through the process of loading PyTorch DataLoader into the GPU. We will cover the basics of PyTorch, GPU architecture, and the steps required to load the data into the GPU.
Table of Contents
- Introduction to PyTorch
- Understanding GPU Architecture
- Steps to Load PyTorch DataLoader into GPU
- Common Errors and Solutions
- Conclusion
Introduction to PyTorch
PyTorch is an open-source machine learning library that is widely used for data processing, deep learning, and neural network modeling. PyTorch is known for its flexibility, ease of use, and speed. It is built on top of the Torch library and uses tensors to represent data.
PyTorch supports both CPU and GPU processing. GPUs are known for their parallel processing capabilities, which are essential for deep learning and other intensive data processing tasks. GPUs can process large amounts of data in parallel, which makes them ideal for processing large datasets.
Understanding GPU Architecture
Before we dive into the process of loading PyTorch DataLoader into the GPU, it is important to understand the GPU architecture. GPUs are designed to handle parallel processing tasks, which means they can efficiently process large datasets. A GPU consists of multiple cores, each of which can process data in parallel.
When loading PyTorch DataLoader into the GPU, the data is first transferred from the CPU to the GPU memory. The GPU memory is then divided into multiple blocks, each of which is assigned to a specific core. The data is then processed in parallel by each core, which speeds up the processing time.
Steps to Load PyTorch DataLoader into GPU
Now that we have covered the basics of PyTorch and GPU architecture, let’s dive into the steps required to load PyTorch DataLoader into the GPU.
Step 1: Define the Dataset and DataLoader
The first step is to define the dataset and DataLoader. The dataset contains the raw data that we want to process, while the DataLoader is responsible for loading the data and preprocessing it.
import torch
from torch.utils.data import Dataset, DataLoader
class CustomDataset(Dataset):
def __init__(self):
self.data = # Load your data here
def __getitem__(self, index):
return self.data[index]
def __len__(self):
return len(self.data)
dataset = CustomDataset()
dataloader = DataLoader(dataset, batch_size=32, shuffle=True)
In the above code, we define a CustomDataset class that loads our data and defines the __getitem__
and __len__
methods. We then create a DataLoader object that loads our dataset with a batch size of 32 and shuffles the data.
Step 2: Define the Model
The next step is to define the model. The model is responsible for processing the data and generating the output.
import torch.nn as nn
class CustomModel(nn.Module):
def __init__(self):
super(CustomModel, self).__init__()
self.fc1 = nn.Linear(10, 5)
self.fc2 = nn.Linear(5, 2)
def forward(self, x):
x = self.fc1(x)
x = self.fc2(x)
return x
model = CustomModel()
In the above code, we define a CustomModel class that consists of two fully connected layers. The forward
method defines how the data is processed by the model.
Step 3: Define the Loss Function and Optimizer
The next step is to define the loss function and optimizer. The loss function calculates the difference between the predicted output and the actual output. The optimizer is responsible for updating the model parameters based on the calculated loss.
criterion = nn.CrossEntropyLoss()
optimizer = torch.optim.SGD(model.parameters(), lr=0.001)
In the above code, we define the CrossEntropyLoss as our loss function and the Stochastic Gradient Descent (SGD) optimizer with a learning rate of 0.001.
Step 4: Load Data into GPU
The final step is to load the data into the GPU. We can do this by calling the to
method on the model and the data.
device = torch.device("cuda:0" if torch.cuda.is_available() else "cpu")
model.to(device)
for data in dataloader:
inputs = data.to(device)
labels = data.to(device)
optimizer.zero_grad()
outputs = model(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
In the above code, we first check if a GPU is available and assign it to the device
variable. We then load the model into the GPU using the to
method. Finally, we loop through the data using the DataLoader and load the data into the GPU using the to
method. We then calculate the loss, backpropagate the gradients, and update the model parameters using the optimizer.
Common Errors and Solutions
"RuntimeError: Input type (torch.FloatTensor) and weight type (torch.cuda.FloatTensor) should be the same"
Ensure that both your model and data are on the same device. Convert the model to GPU using .to()
or torch.device()
.
# Solution
model = model.to(device)
Out of Memory Error
Error: This error occurs when the GPU memory is insufficient to load the entire dataset or batch.
Solution: Reduce batch size or use method 2 (loading batches) for large datasets.
# Adjusting batch size
dataloader = torch.utils.data.DataLoader(dataset, batch_size=32, shuffle=True, pin_memory=True)
Data Type Mismatch Error
Error: Incompatible data types between CPU and GPU.
Solution: Ensure data types match by converting tensors explicitly.
# Convert tensor to float32
data = data.to(torch.float32)
Conclusion
In this tutorial, we have covered the basics of PyTorch, GPU architecture, and the steps required to load PyTorch DataLoader into the GPU. We hope that this tutorial has provided you with a solid understanding of how to efficiently load and process large datasets in PyTorch using the GPU. By following the steps outlined in this tutorial, you can speed up your data processing tasks and improve the overall performance of your models.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.