How to Iterate through Specific Columns and Rows in Pandas Dataframe to Perform a Check
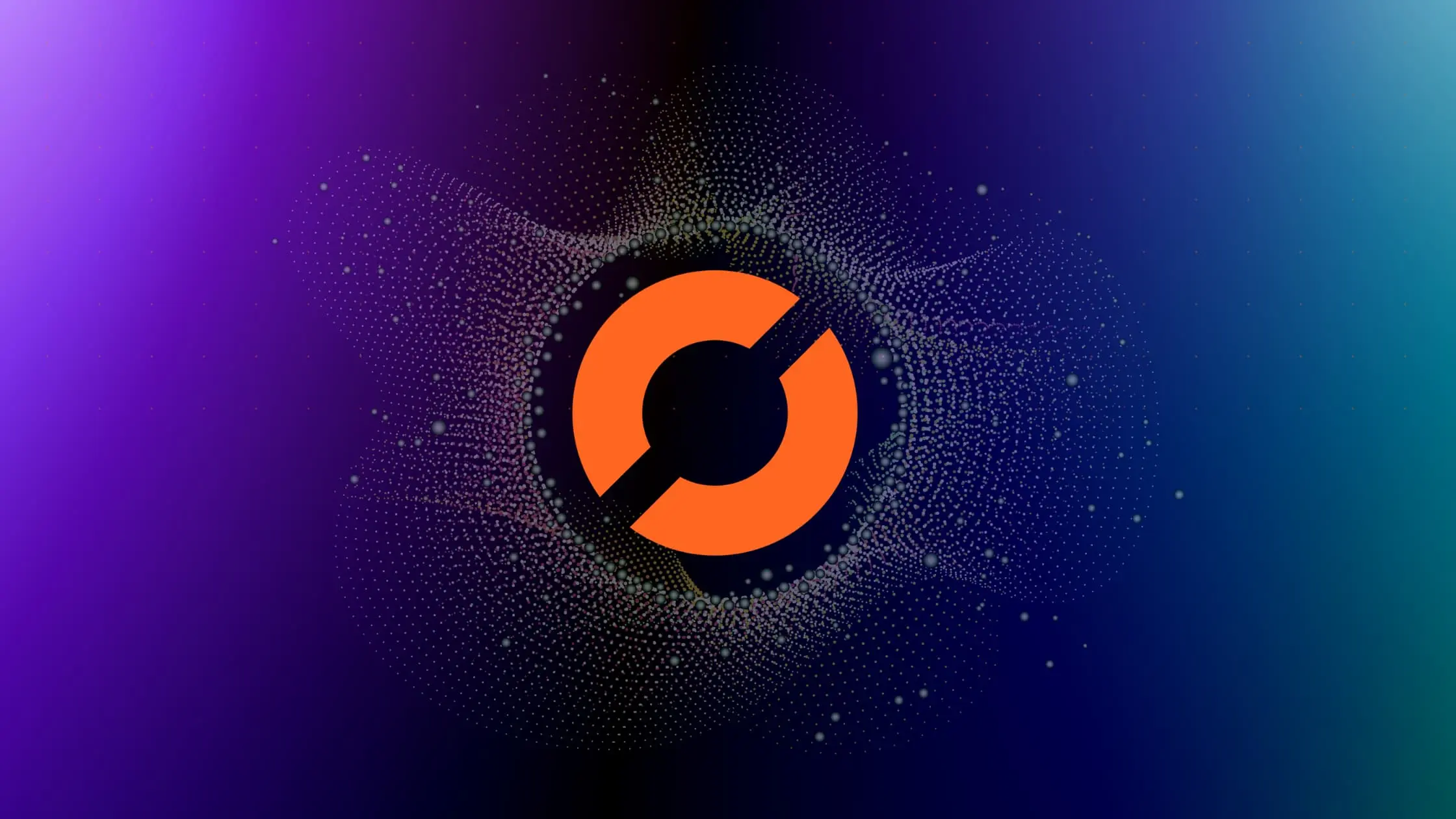
As a data scientist or software engineer, it’s common to work with datasets in various formats. One of the most popular data analysis libraries in Python is Pandas. Pandas provides data structures and functions to manipulate and analyze datasets, making data analysis tasks easier and more efficient.
When working with large datasets, it’s often necessary to iterate through specific columns and rows in a Pandas dataframe to perform a check or operation. In this post, we’ll explore how to iterate through specific columns and rows in a Pandas dataframe to perform a check.
What is a Pandas Dataframe?
Before we dive into how to iterate through specific columns and rows in a Pandas dataframe, let’s first define what a Pandas dataframe is. A Pandas dataframe is a two-dimensional labeled data structure with columns of potentially different types. It’s similar to a spreadsheet or SQL table, but with added functionality.
A Pandas dataframe can be created in many ways, such as from a CSV file, an Excel file, or a SQL query. Once created, the data can be manipulated in various ways using functions provided by Pandas.
Iterating through Specific Columns and Rows
Iterating through specific columns and rows in a Pandas dataframe can be done using the iterrows()
function. This function iterates over the rows of the dataframe, returning the index of each row and a series containing the data in the row.
import pandas as pd
# create a sample dataframe
df = pd.DataFrame({
'name': ['John', 'Jane', 'Bob'],
'age': [25, 30, 35],
'gender': ['male', 'female', 'male']
})
# iterate through rows of the dataframe
for index, row in df.iterrows():
print(row['name'], row['age'])
Output:
John 25
Jane 30
Bob 35
In this example, we create a sample dataframe with three columns: name, age, and gender. We then iterate through the rows of the dataframe using iterrows()
and print the name and age of each row.
If we only want to iterate through specific columns of the dataframe, we can use the loc
function to select the desired columns before iterating. For example:
# iterate through specific columns of the dataframe
for index, row in df.loc[:, ['name', 'age']].iterrows():
print(row['name'], row['age'])
Output:
John 25
Jane 30
Bob 35
In this example, we use the loc
function to select only the name and age columns of the dataframe before iterating.
Performing a Check
Once we’ve iterated through specific columns and rows of a Pandas dataframe, we can perform a check or operation on the data. For example, we can check if a value in a specific column meets a certain condition.
# check if age is greater than 30
for index, row in df.iterrows():
if row['age'] > 30:
print(row['name'], 'is over 30')
Output:
Bob is over 30
In this example, we iterate through the rows of the dataframe and check if the age of each row is greater than 30. If it is, we print the name of the person and a message indicating that they are over 30.
We can also perform more complex checks or operations using functions or libraries. For example, we can use the NumPy library to calculate the mean age of all people in the dataframe.
import numpy as np
# calculate the mean age of all people in the dataframe
ages = df['age'].values
mean_age = np.mean(ages)
print('Mean age:', mean_age)
Output:
Mean age: 30.0
In this example, we use the NumPy library to calculate the mean age of all people in the dataframe.
Conclusion
Iterating through specific columns and rows in a Pandas dataframe to perform a check is a common task in data analysis. Pandas provides a simple and efficient way to do this using the iterrows()
function. By selecting the desired columns using the loc
function, we can iterate through only the data we need. Once we’ve iterated through the data, we can perform checks or operations using functions or libraries.
In this post, we’ve covered some basic examples of how to iterate through specific columns and rows in a Pandas dataframe to perform a check. With these techniques, you’ll be able to efficiently analyze and manipulate datasets using Pandas.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.