How to Initialize Weights in PyTorch A Guide for Data Scientists
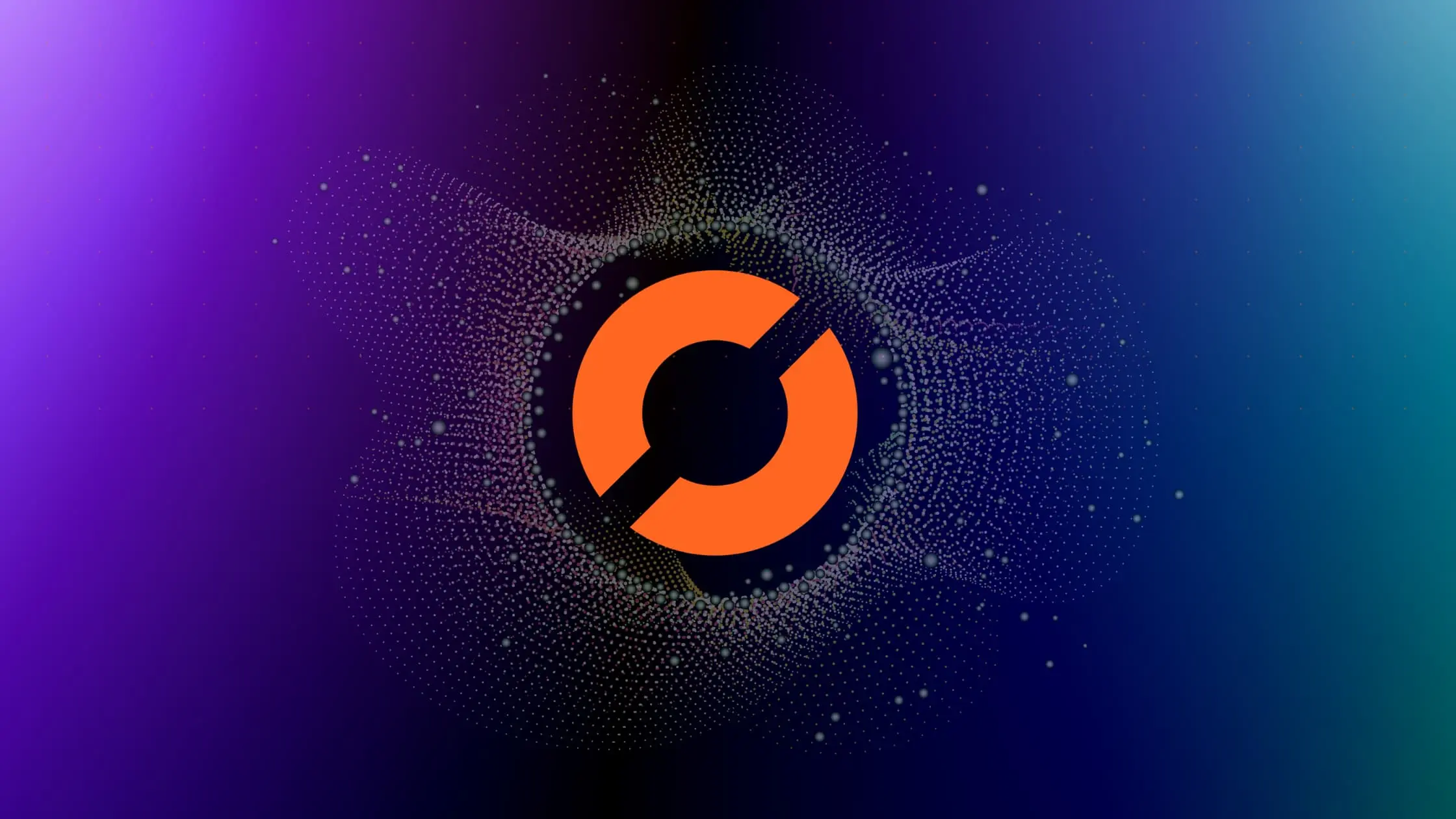
As a data scientist, you know that PyTorch is one of the most popular deep learning frameworks. It offers flexibility and ease of use, making it a go-to choice for many developers. In this post, we’ll explore one of the key components of building deep learning models: weight initialization.
Initializing weights is an important step in the training process of a neural network. A well-initialized network can help improve accuracy and reduce the time required for convergence. In this post, we’ll cover the basics of weight initialization in PyTorch and explore some of the most common techniques used by data scientists.
Table of Contents
What is Weight Initialization?
Weight initialization is the process of setting initial values for the weights of a neural network. In PyTorch, weights are the learnable parameters of a neural network that are updated during the training process. Initializing weights is important because it can affect the performance of the model during training.
There are several techniques for weight initialization, and PyTorch offers a range of options to customize this process. The goal of weight initialization is to find the optimal starting values for the weights so that the network can converge faster and achieve better accuracy.
Common Techniques for Weight Initialization
Here are some of the most common techniques for weight initialization in PyTorch:
1. Zero Initialization
Zero initialization is the simplest technique for weight initialization. It sets all the weights to zero. While this approach is simple to implement, it can cause problems during training. When all the weights are initialized to zero, the network will not be able to learn any meaningful patterns, making it difficult to achieve good accuracy.
import torch.nn as nn
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.fc1 = nn.Linear(784, 10)
nn.init.zeros_(self.fc1.weight)
nn.init.zeros_(self.fc1.bias)
Zero initialization sets all weights to zero. While it is straightforward to implement, it is not recommended for deep networks. Initializing all weights to zero makes it challenging for the network to learn meaningful patterns, hindering the model’s ability to achieve good accuracy. This method is suitable for very specific scenarios where a trivial initialization is acceptable, but it’s generally avoided in deep learning.
2. Random Initialization
Random initialization is a popular technique for weight initialization. It sets the weights to random values sampled from a normal distribution. This approach allows the network to explore a wider range of weights and can help prevent the network from getting stuck in local minima.
import torch.nn as nn
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.fc1 = nn.Linear(784, 10)
nn.init.normal_(self.fc1.weight, mean=0, std=0.01)
nn.init.normal_(self.fc1.bias, mean=0, std=0.01)
Random initialization involves setting weights to random values sampled from a normal distribution. This approach allows the neural network to explore a broader range of weight configurations, preventing it from being stuck in local minima during training. Random initialization is a common choice for various neural network architectures and is especially effective in preventing symmetry issues that can occur with zero initialization.
3. Xavier Initialization
Xavier initialization is a widely used technique for weight initialization. It sets the weights to random values sampled from a normal distribution with a mean of 0 and a variance of 1/n, where n is the number of inputs to the neuron. This approach ensures that the variance of the output of each neuron is approximately equal to the variance of its input.
import torch.nn as nn
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.fc1 = nn.Linear(784, 10)
nn.init.xavier_normal_(self.fc1.weight)
nn.init.zeros_(self.fc1.bias)
Xavier initialization sets weights to random values sampled from a normal distribution with a mean of 0 and a variance of 1/n, where n is the number of inputs to the neuron. This method strikes a balance between avoiding vanishing or exploding gradients, which is crucial for training deep networks. Xavier initialization is well-suited for networks with sigmoid or hyperbolic tangent (tanh) activation functions and helps in achieving stable convergence during training.
4. He Initialization
He initialization is similar to Xavier initialization, but it sets the variance of the weights to 2/n instead of 1/n. This approach is more suitable for rectified linear units (ReLU) because it prevents the gradients from vanishing during the training process.
import torch.nn as nn
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.fc1 = nn.Linear(784, 10)
nn.init.kaiming_normal_(self.fc1.weight, mode='fan_in', nonlinearity='relu')
nn.init.zeros_(self.fc1.bias)
He initialization is similar to Xavier initialization but adjusts the variance of weights to 2/n, which is particularly suitable for rectified linear units (ReLU) activation functions. ReLU can suffer from the vanishing gradient problem, and He initialization helps prevent this issue, making it a preferred choice for networks that utilize ReLU activation functions. This method is beneficial for deep networks, contributing to faster and more stable convergence during training.
Conclusion
In this post, we discussed the importance of weight initialization in PyTorch and explored some of the most common techniques used by data scientists. Initializing weights is a crucial step in building deep learning models, and the right technique can help improve accuracy and reduce the time required for convergence.
When choosing a weight initialization technique, it’s important to consider the architecture of the network and the type of activation function being used. By experimenting with different techniques, you can find the optimal starting values for the weights and achieve better performance in your models.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.