How to Improve Pandas Merge Performance Tips and Tricks
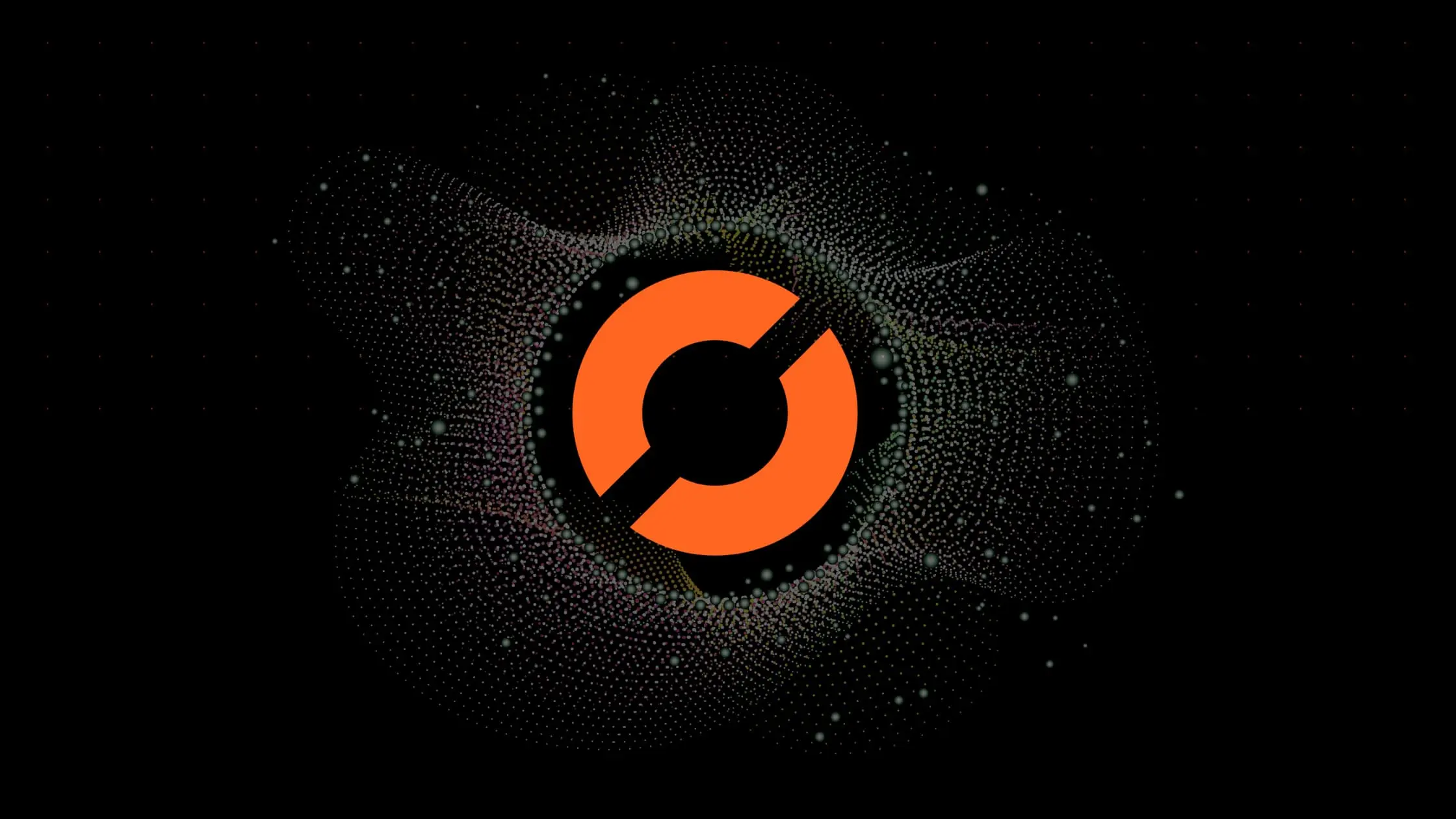
As a data scientist or software engineer, you’re probably familiar with the powerful Pandas library for data manipulation and analysis. One of the most common tasks in data science is merging or joining datasets, which can be computationally expensive when working with large datasets. In this article, we’ll explore some tips and tricks for improving the performance of Pandas merge operations.
Table of Contents
- Introduction
- Why is Pandas Merge Performance Important
- Tips for Improving Pandas Merge Performance
- Common Errors and Troubleshooting
- Conclusion
What is Pandas Merge?
Pandas merge is a function that combines two or more dataframes based on a common column or index. This operation is similar to the SQL join operation and is essential in data analysis when working with multiple datasets. The merge function in Pandas allows you to perform various types of joins, such as inner join, outer join, left join, and right join.
Why is Pandas Merge Performance Important?
Pandas merge performance is crucial because merging large datasets can be computationally intensive and time-consuming. In some cases, a poorly optimized merge operation can cause the Python interpreter to run out of memory or even crash. Therefore, it’s essential to optimize your merge operations to improve the performance of your data analysis pipeline.
Tips for Improving Pandas Merge Performance
Tip #1: Use the Correct Join Type
The first tip to improve Pandas merge performance is to use the correct join type. Pandas supports various types of joins, and each one has its own performance characteristics. In general, the inner join is the fastest and most efficient type of join, followed by the left join and then the outer join. The right join is the slowest and least efficient type of join.
Therefore, if you’re not sure which join type to use, start with the inner join. This type of join only returns the rows that have matching values in both dataframes, which reduces the size of the output dataframe and speeds up the merge operation.
Tip #2: Sort Dataframes Before Merging
Another tip to improve Pandas merge performance is to sort the dataframes before merging. Sorting the dataframes can significantly reduce the merge time, especially when using the merge function with the outer join or the left join. When you sort your dataframes, Pandas can use a more efficient merge algorithm called the merge-join algorithm.
To sort a dataframe, use the sort_values
method and specify the column or columns to sort by. For example, to sort a dataframe by the ‘id’ column, you can use the following code:
df.sort_values('id', inplace=True)
Tip #3: Reduce Dataframe Size
Another way to improve Pandas merge performance is to reduce the size of the dataframes before merging. One way to reduce the size of a dataframe is to select only the columns that you need for the merge operation. This reduces the memory usage and speeds up the merge operation.
To select specific columns from a dataframe, use the loc
method and specify the column names. For example, to select the ‘id’ and ‘name’ columns from a dataframe, you can use the following code:
df = df.loc[:, ['id', 'name']]
Tip #4: Utilize Categorical Data Types
Categorical data types are a powerful feature in Pandas that can significantly improve merge performance. Categorical data types can reduce memory usage and speed up merge operations by storing the data as integers instead of strings. This reduces the amount of memory required to store the data and speeds up comparison operations.
To use categorical data types in Pandas, use the astype
method and specify the data type as ‘category’. For example, to convert the ‘gender’ column to a categorical data type, you can use the following code:
df['gender'] = df['gender'].astype('category')
Tip #5: Employ the merge Method with the query Parameter
Finally, another way to improve Pandas merge performance is to use the merge
method with the query
parameter. The query
parameter allows you to filter the dataframes before merging, which reduces the size of the dataframes and speeds up the merge operation.
To use the query
parameter, specify a Boolean expression that filters the rows in the dataframe. For example, to merge only the rows where the ‘age’ column is greater than 18, you can use the following code:
df1.merge(df2.query('age > 18'), on='id', how='left')
Handling Common Errors
Duplicate Key Values:
Duplicate key values can lead to unexpected results during the merge operation. Identifying and handling these duplicates is crucial for maintaining data integrity.
Solution
# Detect duplicate
duplicates = df[df.duplicated('key')]
# Handle Duplicates:
# Drop duplicates if they are redundant:
df.drop_duplicates('key', inplace=True)
# Aggregate or resolve duplicates based on the business logic:
df_agg = df.groupby('key').agg({'column1': 'sum', 'column2': 'mean'})
Missing Values:
Missing values in the merged columns can impact analysis and downstream operations. Proper handling of missing values is essential for obtaining accurate results.
Solution:
# Find missing values
missing_values = merged_df.isnull().sum()
# Handle Missing Values:
# Drop rows with missing values:
merged_df.dropna(inplace=True)
# Fill missing values using specific strategies:
merged_df.fillna(value, inplace=True)
#Interpolate missing values for numerical columns:
merged_df.interpolate(inplace=True)
Memory Errors:
For large datasets, memory errors may occur during the merge operation, hindering the process. It’s crucial to address these issues to ensure smooth execution.
Solution: Use Dask for Parallelization**: Dask is a parallel computing library that enables parallel and distributed computing. It can handle larger-than-memory datasets by breaking them into smaller tasks.
import dask.dataframe as dd
ddf1 = dd.from_pandas(df1, npartitions=n)
ddf2 = dd.from_pandas(df2, npartitions=m)
merged_dask = dd.merge(ddf1, ddf2, on='key').compute()
Adjust n and m based on available resources and dataset size.
Conclusion
In conclusion, Pandas merge performance is an essential aspect of data analysis and can significantly impact the efficiency of your data analysis pipeline. By following the tips and tricks outlined in this article, you can improve the performance of your Pandas merge operations and speed up your data analysis pipeline. Remember to use the correct join type, sort the dataframes before merging, reduce the size of the dataframes, use categorical data types, and use the merge
method with the query
parameter to filter the dataframes.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.