How to Improve Accuracy in Neural Networks with Keras
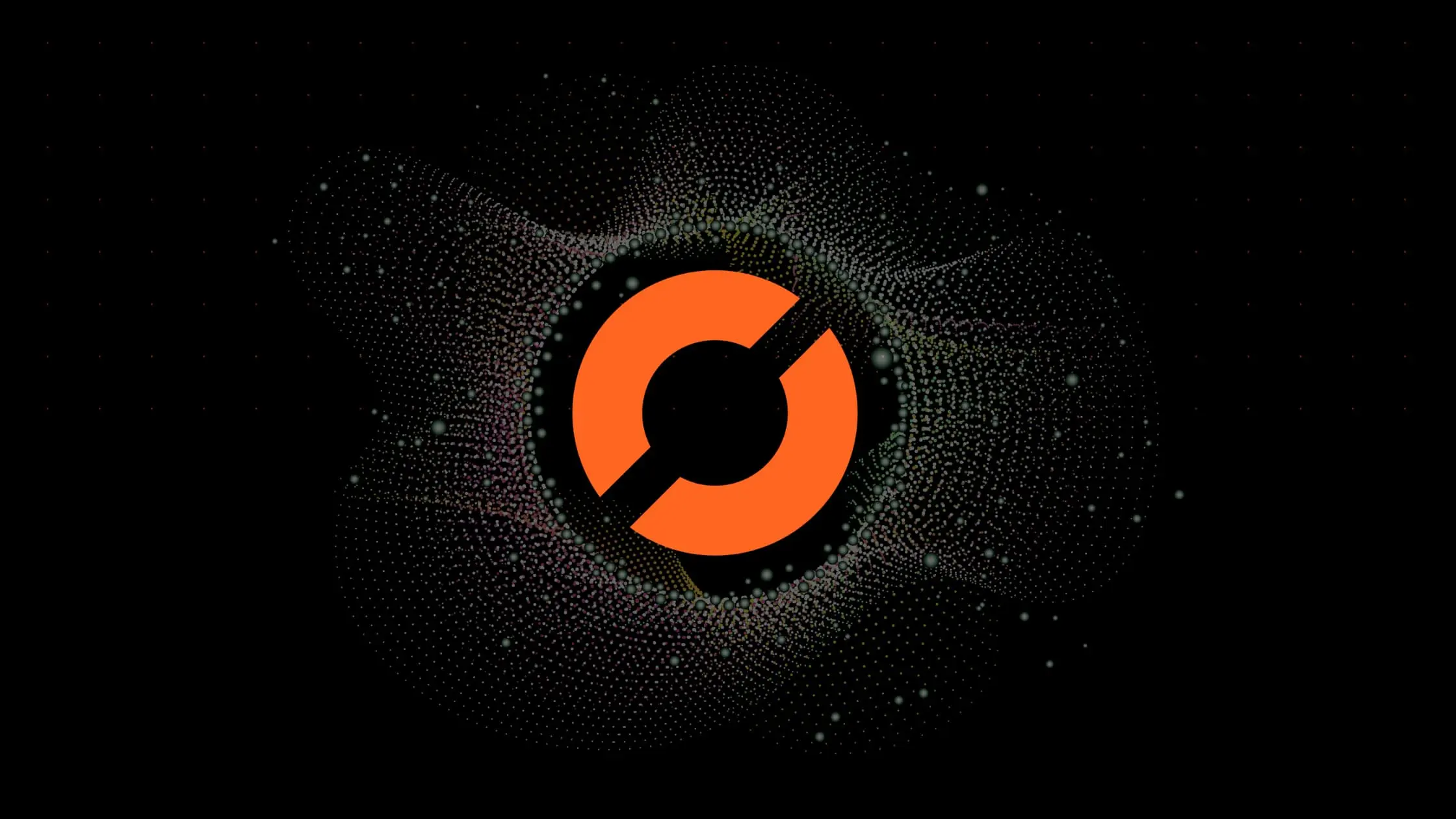
As a data scientist or software engineer, you know that neural networks are powerful tools for machine learning. However, building a neural network that accurately predicts outcomes can be a challenge. Fortunately, Keras provides a simple and efficient way to build and train neural networks. In this article, we will explore some techniques to improve the accuracy of neural networks built with Keras.
Table of Contents
- What is Keras?
- Understanding Accuracy
- Techniques to Improve Accuracy
- Common Errors and How to Handle Them
- Conclusion
What is Keras?
Keras is an open-source neural network library written in Python. It is designed to be user-friendly, modular, and extensible. It can run on top of TensorFlow, Theano, or CNTK. Keras provides a simple and high-level interface for building and training neural networks, making it a popular tool for data scientists and software engineers.
Understanding Accuracy
Before we dive into techniques to improve accuracy, it is important to understand what accuracy means in the context of neural networks. Accuracy is a measure of how well a neural network can predict outcomes. It is calculated as the percentage of correct predictions out of the total number of predictions.
For example, if a neural network correctly predicts 90 out of 100 outcomes, its accuracy is 90%. In general, a higher accuracy indicates a better-performing neural network.
Techniques to Improve Accuracy
Now that we understand accuracy, let’s explore some techniques to improve it in neural networks built with Keras.
1. Data Preprocessing
Data preprocessing is a crucial step in enhancing model performance. Ensure that your data is clean, well-structured, and appropriately scaled. Common techniques include normalization, handling missing values, and data augmentation for image datasets. Normalize the Input Data
To normalize the input data in Keras, we can use the normalize()
function from the sklearn.preprocessing
module. Here’s an example:
from sklearn.preprocessing import normalize
X_train_normalized = normalize(X_train, axis=0)
X_test_normalized = normalize(X_test, axis=0)
To perform the augmentation, we can use ImageGenerator
in Keras.
# Code example for data augmentation using Keras ImageDataGenerator
from keras.preprocessing.image import ImageDataGenerator
# Create an ImageDataGenerator instance
datagen = ImageDataGenerator(
rotation_range=40,
width_shift_range=0.2,
height_shift_range=0.2,
shear_range=0.2,
zoom_range=0.2,
horizontal_flip=True,
fill_mode='nearest'
)
# Apply data augmentation to your dataset
datagen.fit(train_data)
2. Increase the Number of Layers
Increasing the number of layers in a neural network can improve its accuracy. Adding more layers can help the neural network learn more complex patterns in the data, which can lead to better predictions.
To add more layers to a neural network in Keras, we can use the add()
method of the Sequential
class. Here’s an example:
from keras.models import Sequential
from keras.layers import Dense
model = Sequential()
model.add(Dense(units=64, activation='relu', input_dim=100))
model.add(Dense(units=64, activation='relu'))
model.add(Dense(units=1, activation='sigmoid'))
3. Increase the Number of Neurons
Increasing the number of neurons in a neural network can also improve its accuracy. Adding more neurons can help the neural network learn more complex patterns in the data, which can lead to better predictions.
To increase the number of neurons in a layer in Keras, we can specify the units
parameter when adding the layer. Here’s an example:
from keras.models import Sequential
from keras.layers import Dense
model = Sequential()
model.add(Dense(units=128, activation='relu', input_dim=100))
model.add(Dense(units=1, activation='sigmoid'))
4. Use Dropout Regularization
Overfitting is a common problem in machine learning, where a model becomes too complex and starts to memorize the training data instead of learning to generalize. Dropout regularization is a technique that can help prevent overfitting and improve the accuracy of a neural network.
Dropout regularization involves randomly dropping out some neurons during training. This forces the neural network to learn more robust representations of the data, as it cannot rely on any single neuron to make predictions.
To use dropout regularization in Keras, we can add a Dropout
layer after a Dense
layer. Here’s an example:
from keras.models import Sequential
from keras.layers import Dense, Dropout
model = Sequential()
model.add(Dense(units=64, activation='relu', input_dim=100))
model.add(Dropout(0.5))
model.add(Dense(units=1, activation='sigmoid'))
5. Increase the Number of Epochs
Training a neural network involves iterating over the training data multiple times, which are called epochs. Increasing the number of epochs can improve the accuracy of a neural network, as it allows the neural network to learn more from the data.
To increase the number of epochs in Keras, we can specify the epochs
parameter when calling the fit()
method. Here’s an example:
model.fit(X_train, y_train, epochs=10, batch_size=32)
6. Hyperparameter Tuning
Optimizing hyperparameters is an ongoing process. Experiment with learning rates, batch sizes, and epochs to find the best combination for your specific task. Utilize tools like grid search or random search for efficient exploration.
from keras.models import Sequential
from keras.layers import Dense
from keras.wrappers.scikit_learn import KerasClassifier
from sklearn.model_selection import GridSearchCV
from sklearn.datasets import make_classification
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
# Generate a sample dataset
X, y = make_classification(n_samples=1000, n_features=20, n_classes=2, random_state=42)
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Standardize the data
scaler = StandardScaler()
X_train = scaler.fit_transform(X_train)
X_test = scaler.transform(X_test)
# Define a function to create the Keras model
def create_model(optimizer='adam', activation='relu'):
model = Sequential()
model.add(Dense(12, input_dim=20, activation=activation))
model.add(Dense(1, activation='sigmoid'))
model.compile(loss='binary_crossentropy', optimizer=optimizer, metrics=['accuracy'])
return model
# Create a KerasClassifier wrapper for Scikit-learn
model = KerasClassifier(build_fn=create_model, epochs=10, batch_size=32, verbose=0)
# Define the hyperparameters to tune
param_grid = {
'optimizer': ['adam', 'sgd', 'rmsprop'],
'activation': ['relu', 'tanh'],
'batch_size': [16, 32, 64],
'epochs': [10, 20, 30]
}
# Use GridSearchCV to find the best combination of hyperparameters
grid = GridSearchCV(estimator=model, param_grid=param_grid, cv=3)
grid_result = grid.fit(X_train, y_train)
# Display the best hyperparameters and corresponding accuracy
print(f"Best Parameters: {grid_result.best_params_}")
print(f"Best Accuracy: {grid_result.best_score_}")
Common Errors and How to Handle Them
Overfitting
Overfitting occurs when a model learns the training data too well but performs poorly on new, unseen data. To address this, use techniques like dropout, data augmentation, and reduce model complexity.
Underfitting
Underfitting happens when a model is too simple to capture the underlying patterns. Increase model complexity, add more layers or neurons, and optimize hyperparameters to overcome underfitting.
Vanishing or Exploding Gradients
Vanishing or exploding gradients can hinder training. Implement techniques such as gradient clipping, weight initialization, or use architectures designed to handle gradient-related issues.
Poor Data Quality
Low-quality data can degrade model performance. Address missing values, outliers, and inconsistencies in the dataset. Consider data augmentation to artificially increase the size of your training set.
Inadequate Training Data
Insufficient training data can lead to poor generalization. Augment your dataset, use transfer learning, or explore techniques like semi-supervised learning when dealing with limited data.
Conclusion
Building a neural network that accurately predicts outcomes can be a challenge. However, with Keras, we can build and train neural networks efficiently. In this article, we explored some techniques to improve the accuracy of neural networks built with Keras. By normalizing the input data, increasing the number of layers and neurons, using dropout regularization, and increasing the number of epochs, we can build neural networks that perform better on our data.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.