How to Handle the 'ValueError: Input contains NaN, infinity or a value too large for dtype('float64')' Error in scikit-learn
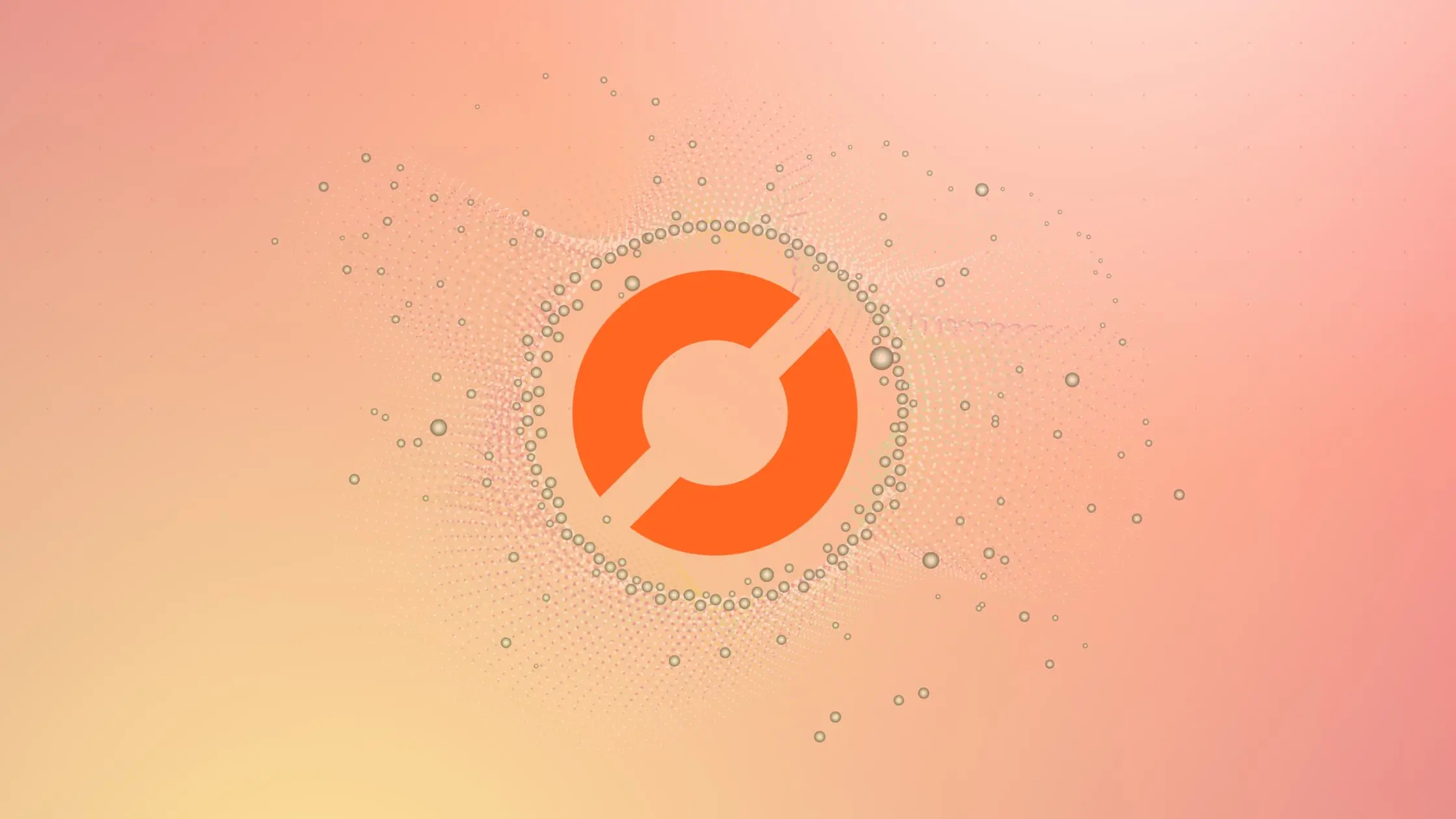
As a data scientist or software engineer, you may have encountered the error ValueError: Input contains NaN, infinity or a value too large for dtype('float64')
when using scikit-learn (sklearn) for machine learning tasks. This error occurs when there are missing values or infinite values in your dataset. In this article, we will discuss how to handle this error in scikit-learn.
Table of Contents
- What is scikit-learn?
- What Causes the
ValueError: Input contains NaN, infinity or a value too large for dtype(‘float64’)
Error? - How to Handle the
ValueError: Input contains NaN, infinity or a value too large for dtype(‘float64’)
Error - Best Practices for Handling NaN, Infinity, or Large Values
- Conclusion
What is scikit-learn?
Scikit-learn is a popular Python library for machine learning. It provides a range of tools for data preprocessing, feature selection, model selection, and performance evaluation. Scikit-learn is widely used in the industry for building and deploying machine learning models.
What Causes the ValueError: Input contains NaN, infinity or a value too large for dtype('float64')
Error?
The ValueError: Input contains NaN, infinity or a value too large for dtype('float64')
error occurs when there are missing or infinite values in your dataset. Scikit-learn requires that all input data be numeric and finite. If there are any missing or infinite values in your dataset, scikit-learn will raise this error.
How to Handle the ValueError: Input contains NaN, infinity or a value too large for dtype('float64')
Error
There are several ways to handle the ‘ValueError: Input contains NaN, infinity or a value too large for dtype(‘float64’)’ error in scikit-learn.
1. Remove Rows with Missing Values
One way to handle the error is to remove all rows that contain missing values. This can be done using the dropna()
method in pandas. For example:
import pandas as pd
# Load dataset
data = pd.read_csv('dataset.csv')
# Remove rows with missing values
data.dropna(inplace=True)
# Split data into features and target
X = data.drop('target', axis=1)
y = data['target']
This approach is simple and easy to implement, but it may result in a loss of data. If there are many missing values in your dataset, removing all rows with missing values may not be a viable option.
2. Impute Missing Values
Another way to handle missing values is to impute them. Imputation is the process of filling in missing values with estimated values. There are several methods for imputing missing values, such as mean imputation, median imputation, and k-Nearest Neighbors (k-NN) imputation.
For example, to impute missing values with the mean value of each column, you can use the SimpleImputer
class in scikit-learn:
from sklearn.impute import SimpleImputer
# Load dataset
data = pd.read_csv('dataset.csv')
# Impute missing values with mean
imputer = SimpleImputer(strategy='mean')
imputed_data = imputer.fit_transform(data)
# Split data into features and target
X = imputed_data.drop('target', axis=1)
y = imputed_data['target']
This approach preserves all rows in the dataset and can improve the accuracy of your machine learning model. However, imputation introduces bias into the dataset, and the imputed values may not be accurate reflections of the true values.
3. Scale the Data
The ‘ValueError: Input contains NaN, infinity or a value too large for dtype(‘float64’)’ error can also occur if the data in your dataset is not scaled. Scaling is the process of transforming the data so that it has a mean of zero and a standard deviation of one. This is important for some machine learning algorithms, such as those that use distance-based metrics.
To scale the data, you can use the StandardScaler
class in scikit-learn:
from sklearn.preprocessing import StandardScaler
# Load dataset
data = pd.read_csv('dataset.csv')
# Scale data
scaler = StandardScaler()
scaled_data = scaler.fit_transform(data)
# Split data into features and target
X = scaled_data.drop('target', axis=1)
y = scaled_data['target']
This approach can prevent the ValueError: Input contains NaN, infinity or a value too large for dtype('float64')
error and improve the performance of your machine learning model.
Best Practices for Handling NaN, Infinity, or Large Values:
4.1. Data Inspection and Cleaning:
One of the fundamental steps is to inspect your data for missing values. Use tools like pandas to identify and handle NaN values by either removing or imputing them.
4.2. Imputation Techniques:
Imputation involves filling in missing values with estimated ones. Techniques like mean, median, or more advanced methods such as K-Nearest Neighbors (KNN) can be employed.
4.3. Scaling and Normalization:
Scaling and normalization methods ensure that all features are on a similar scale, preventing issues with excessively large values. StandardScaler or MinMaxScaler in scikit-learn can be applied.
Conclusion
The ValueError: Input contains NaN, infinity or a value too large for dtype('float64')
error can be a common issue when using scikit-learn for machine learning tasks. In this article, we discussed several ways to handle this error, including removing rows with missing values, imputing missing values, and scaling the data. By implementing these approaches, you can prevent this error and improve the accuracy of your machine learning models.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.