How to Get the Value of a Tensor in PyTorch
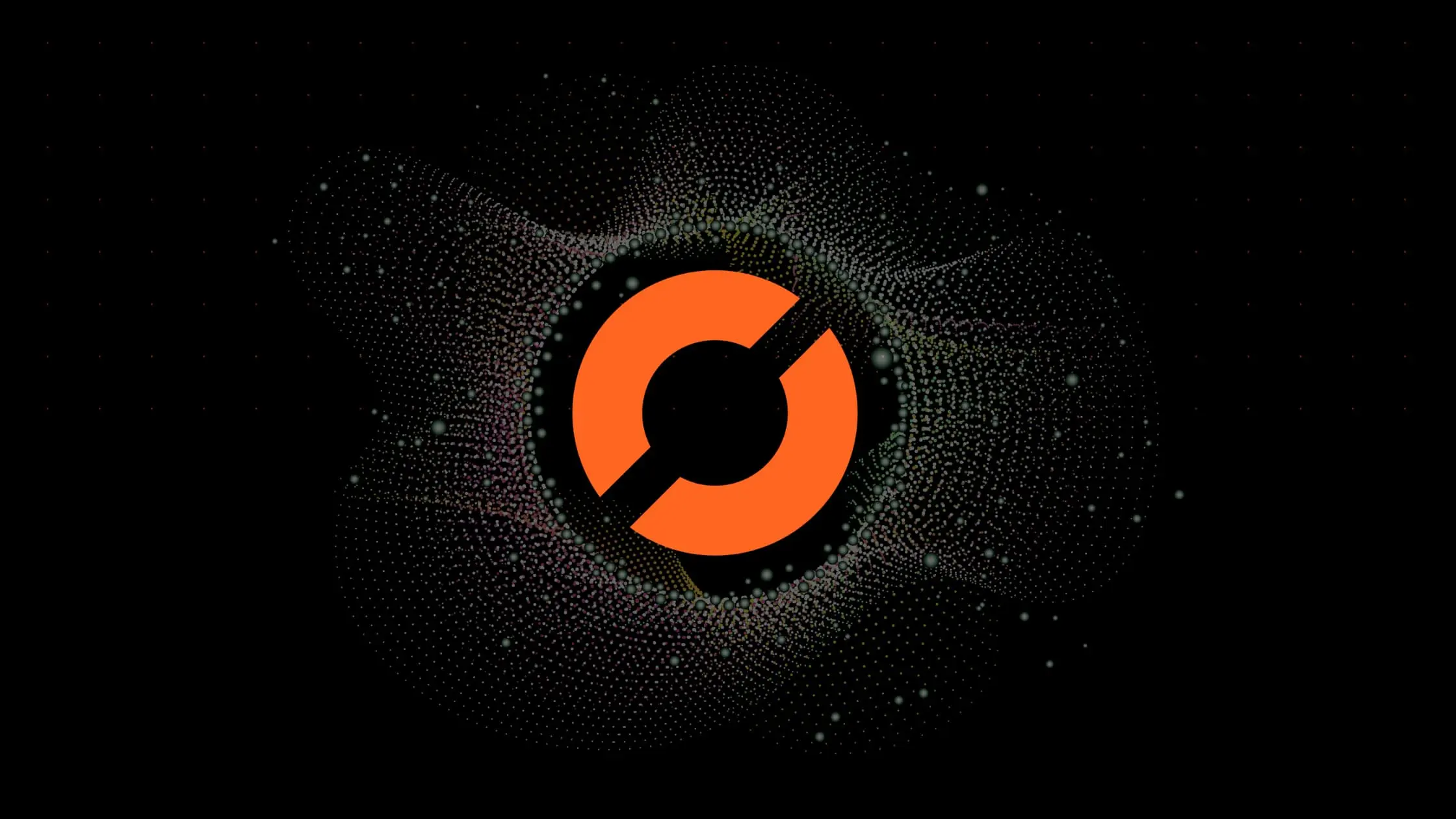
If you’re a data scientist or software engineer working with PyTorch, you’re probably familiar with tensors. Tensors are multi-dimensional arrays that are the basic building blocks of PyTorch. Getting the value of a tensor is a fundamental operation in PyTorch, but if you’re new to the library, you might be wondering how to do it. In this blog post, I’ll explain how to get the value of a tensor in PyTorch.
Table of Contents
- Introduction
- What Is a Tensor?
- Getting the Value of a Tensor
- Best Practices for Working with Tensors in PyTorch
- Conclusion
What Is a Tensor?
A tensor is a multi-dimensional array that can be used to represent data in PyTorch. Tensors are similar to NumPy arrays, but they can be used on GPUs to accelerate the computation of deep neural networks. PyTorch tensors can have any number of dimensions, and each element in the tensor is a numerical value.
Getting the Value of a Tensor
To get the value of a tensor in PyTorch, you can use the .item()
method. This method returns the value of a tensor as a Python scalar. Here’s an example:
import torch
# Create a tensor
x = torch.tensor([1, 2, 3])
# Get the value of an element in the tensor
value = x[0].item()
print(value)
Output:
1
In this example, we created a tensor x
with the values [1, 2, 3]
. We then used the .item()
method to get the value of the firts element in the tensor, which is 1
.
If you have a tensor with more than one element, you can specify the index of the element you want to get the value of. Here’s an example:
import torch
# Create a tensor
x = torch.tensor([[1, 2], [3, 4]])
# Get the value of an element in the tensor
value = x[0, 1].item()
print(value)
Output:
2
In this example, we created a tensor x
with the values [[1, 2], [3, 4]]
. We then used the indexing operator to get the value of the element at row 0 and column 1, which is 2
.
Pros and Cons of Extracting Tensor Values in PyTorch:
Pros
Simplicity and Readability: Using the
.item()
method provides a concise and readable way to extract scalar values from tensors, enhancing the clarity of your code.Versatility with Scalar Values: The method seamlessly converts tensor values into Python scalars, allowing for easy integration with other Python libraries and operations.
Efficient Single-Element Retrieval: For tensors containing a single element, the
.item()
method offers a straightforward solution, eliminating the need for complex indexing.Compatibility with Mathematical Operations: Extracted scalar values can be readily used in various mathematical operations and functions, facilitating the manipulation and analysis of tensor data.
Intuitive Indexing for Multi-Element Tensors: When dealing with tensors of multiple elements, the ability to specify indices for value extraction provides an intuitive and flexible approach.
Cons
Overhead for Large Tensors: In scenarios involving large tensors, extracting values using the
.item()
method for each element can result in computational overhead and may not be the most efficient approach.Potential for IndexError: Improper indexing can lead to
IndexError
if the specified indices are out of bounds. Careful consideration of tensor dimensions is essential to avoid such errors.Limited Support for Non-Scalar Tensors: The
.item()
method is designed for extracting scalar values. If you need to work with non-scalar tensors, alternative approaches or methods may be required, adding complexity to the code.
Using NumPy Conversion
Another approach to extract values from PyTorch tensors is by converting them to NumPy arrays. PyTorch seamlessly integrates with NumPy, allowing you to leverage the functionalities of both libraries. Here’s an example of how to use NumPy conversion to retrieve tensor values:
import torch
import numpy as np
# Create a tensor
x = torch.tensor([[1, 2], [3, 4]])
# Convert the tensor to a NumPy array
numpy_array = x.numpy()
# Get the value of an element in the tensor
value = numpy_array[0, 1]
print(value)
In this example, the .numpy()
method is used to convert the PyTorch tensor x into a NumPy array. After the conversion, you can access the elements using NumPy array indexing. This method provides an alternative way to extract values, especially when dealing with complex numerical operations or integration with existing NumPy-based code.
Pros of NumPy Conversion:
- Compatibility with NumPy: Enables seamless integration with existing NumPy-based workflows, libraries, and operations.
- Advanced NumPy Functionalities: Allows for leveraging advanced numerical functionalities available in NumPy for data manipulation and analysis.
- Multi-Library Interoperability: Facilitates interoperability between PyTorch and other libraries that support NumPy arrays.
Cons of NumPy Conversion:
- Potential Overhead: Converting tensors to NumPy arrays may introduce additional computational overhead, especially for large tensors.
- Memory Usage: Both the PyTorch tensor and NumPy array may exist simultaneously in memory, potentially leading to increased memory usage.
- Data Type Consistency: Care should be taken to ensure consistency in data types between PyTorch tensors and NumPy arrays.
Error Handling Considerations:
IndexError Handling: To mitigate the risk of IndexError when accessing specific elements, it’s essential to implement error-checking mechanisms, such as verifying that the specified indices are within the valid range.
Type Error Handling: Since the
.item()
method is designed for scalar values, it’s crucial to handle cases where non-scalar tensors are encountered. This may involve incorporating conditional statements or utilizing different methods based on the tensor type.Graceful Failure for Unexpected Inputs: Implementing a mechanism to gracefully handle unexpected input types or scenarios ensures that your code remains robust and resilient to unforeseen circumstances.
Documentation and Logging: Clearly documenting the expected input formats, valid indices, and potential errors, along with incorporating logging mechanisms, can assist in debugging and troubleshooting when issues arise.
Best Practices for Working with Tensors in PyTorch
- Use Descriptive Variable Names: When creating tensors, use variable names that convey the meaning of the data they represent. This enhances code readability and understanding, especially when working with complex models.
# Instead of:
x = torch.tensor([1, 2, 3])
# Prefer:
input_values = torch.tensor([1, 2, 3])
- Leverage Tensor Broadcasting: Take advantage of PyTorch’s broadcasting capabilities to perform element-wise operations on tensors of different shapes. This simplifies code and eliminates the need for explicit reshaping.
# Instead of using explicit reshaping:
a = torch.tensor([1, 2, 3])
b = torch.tensor([[4, 5, 6], [7, 8, 9]])
# Use broadcasting:
result = a + b
- Avoid Unnecessary .item() Calls: While the
.item()
method is convenient for obtaining scalar values, avoid unnecessary calls within loops or operations on large tensors. Instead, work with tensors directly when possible to maintain efficiency.
# Instead of:
for element in large_tensor:
value = element.item()
# Perform operations on value
# Prefer:
for element in large_tensor:
# Perform operations on element directly
- Explore In-Place Operations: PyTorch supports in-place operations, which can be more memory-efficient. Be cautious with in-place operations to avoid unintended side effects, but consider them for large-scale computations.
# Instead of creating a new tensor:
result = a + b
# Use in-place operation:
a.add_(b)
- Utilize GPU Acceleration: If available, leverage GPU acceleration for tensor operations to significantly improve computation speed, especially when dealing with large datasets or complex models.
# Move tensor to GPU:
cuda_device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
tensor_on_gpu = tensor.to(cuda_device)
- Regularly Check for Updates: PyTorch is an actively developed library, and updates may introduce new features or optimizations. Stay informed about the latest releases and update your PyTorch installation regularly.
# Update PyTorch using pip:
pip install --upgrade torch
Conclusion
Getting the value of a tensor in PyTorch is a simple operation that can be done using the .item()
method. This method returns the value of a tensor as a Python scalar. If you have a tensor with more than one element, you can specify the index of the element you want to get the value of. I hope this blog post has been helpful in explaining how to get the value of a tensor in PyTorch.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.