How to Get the Directory Where a Bash Script is Located
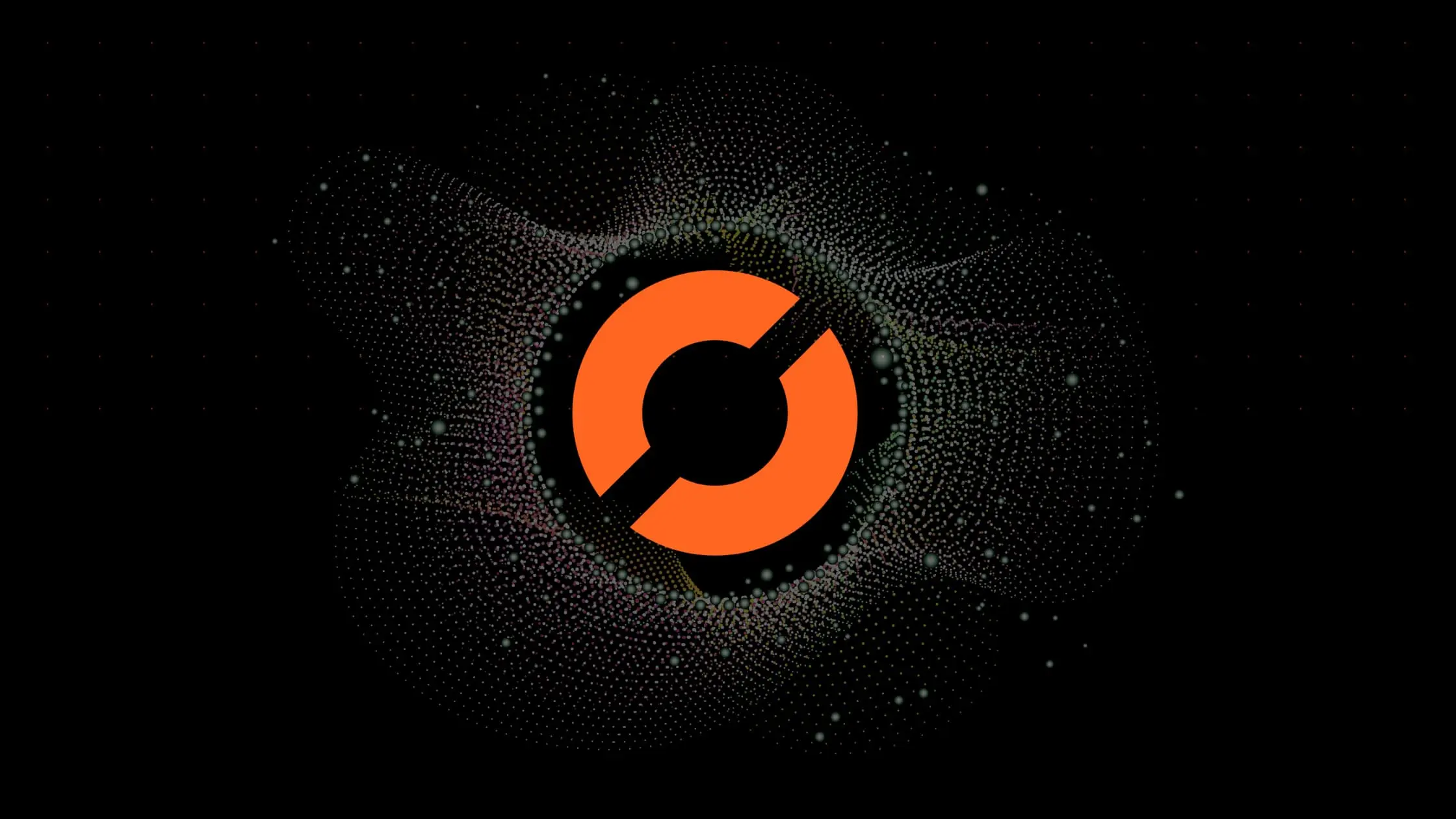
How to Get the Directory Where a Bash Script is Located
As a software engineer, you might have found yourself in a situation where you need to get the directory where a Bash script is located from within the script itself. This can be useful if you need to access files or directories relative to the location of the script. In this blog post, we will discuss various ways to get the directory where a Bash script is located.
Method 1: Using $0
The first method to get the directory where a Bash script is located is by using the $0
variable. The $0
variable contains the name of the currently executing script, including its path. We can use the dirname
command to extract the directory name from the path.
#!/bin/bash
SCRIPT_DIR="$( cd "$( dirname "${BASH_SOURCE[0]}" )" &> /dev/null && pwd )"
echo "Script directory: $SCRIPT_DIR"
In this example, we use the cd
command to change the directory to the script’s location, suppress any error messages with &> /dev/null
, and then use the pwd
command to get the current directory. Finally, we store the result in the SCRIPT_DIR
variable and print it.
Method 2: Using readlink
Another method to get the directory where a Bash script is located is by using the readlink
command. The readlink
command can be used to resolve symbolic links and return the absolute path of a file or directory.
#!/bin/bash
SCRIPT_DIR="$(dirname "$(readlink -f "$0")")"
echo "Script directory: $SCRIPT_DIR"
In this example, we use the readlink
command with the -f
option to get the absolute path of the script. We then use the dirname
command to extract the directory name from the path. Finally, we store the result in the SCRIPT_DIR
variable and print it.
Method 3: Using pwd and cd
The third method to get the directory where a Bash script is located is by using the pwd
and cd
commands.
#!/bin/bash
cd "$(dirname "$0")"
SCRIPT_DIR="$(pwd)"
echo "Script directory: $SCRIPT_DIR"
In this example, we use the dirname
command to extract the directory name from the script’s path. We then use the cd
command to change the current directory to the script’s location. Finally, we use the pwd
command to get the current directory and store the result in the SCRIPT_DIR
variable.
Conclusion
In this blog post, we discussed various ways to get the directory where a Bash script is located. The most common method is by using the $0
variable with the dirname
command. However, we also discussed using the readlink
command and the pwd
and cd
commands. These methods can be useful if you need to access files or directories relative to the location of the script.
It’s important to choose a method that is efficient and reliable. The $0
variable with the dirname
command is the most commonly used method and should work in most cases. However, if you are working with symbolic links or need to change the current directory, you may need to use one of the alternative methods discussed in this blog post.
We hope this blog post has been helpful in understanding how to get the directory where a Bash script is located. If you have any questions or comments, please feel free to leave them below. Happy scripting!
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.