How to Get the Average of a Groupby with Pandas
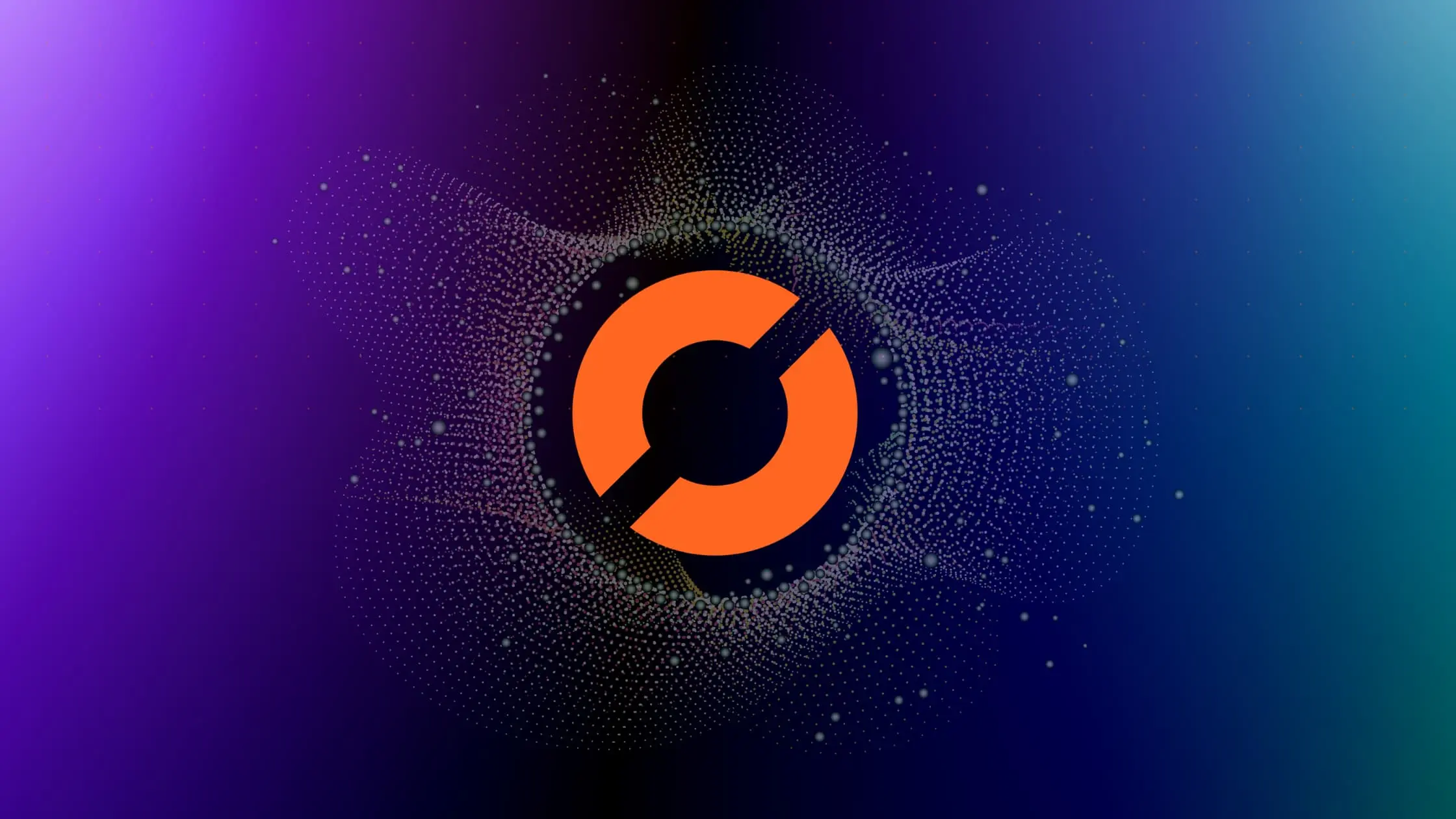
As a data scientist or software engineer, you are likely familiar with the pandas library in Python. Pandas is a powerful tool for data manipulation and analysis, and it is widely used in the data science and software engineering communities.
One common task in data analysis is to group data by a certain column or set of columns and then calculate some summary statistics for each group. For example, you may want to calculate the average value of a certain variable for each group. In this blog post, we will explore how to get the average of a groupby with pandas.
What is Groupby in Pandas?
Before we dive into how to get the average of a groupby with pandas, let’s first understand what groupby is and how it works in pandas.
Groupby is a powerful feature in pandas that allows you to group a DataFrame based on one or more columns. Once you have grouped a DataFrame, you can perform a variety of operations on each group, such as calculating summary statistics, applying functions, or filtering the data.
To group a DataFrame in pandas, you use the groupby
method and specify the column or columns that you want to group by. For example, the following code groups a DataFrame by the category
column:
import pandas as pd
df = pd.DataFrame({
'product': ['A', 'B', 'C', 'A', 'B', 'C'],
'region': ['North', 'North', 'North', 'South', 'South', 'South'],
'sales': [100, 200, 300, 400, 500, 600]
})
grouped = df.groupby('product')
After running this code, grouped
is a pandas GroupBy
object that contains three groups: A
, B
, and C
.
How to Get the Average of a Groupby in Pandas
Now that we understand what groupby is and how it works in pandas, let’s explore how to get the average of a groupby.
To get the average of a groupby in pandas, you can use the mean()
method on the GroupBy
object. This method calculates the mean of each numeric column for each group.
We can group this DataFrame by the product
column and then calculate the average sales for each product:
average_sales = grouped.mean()
print(average_sales)
This will return a new DataFrame that contains the average sales for each product:
sales
product
A 250.0
B 350.0
C 450.0
As you can see, the mean()
method has calculated the average sales for each product.
Groupby with Multiple Columns
In some cases, you may want to group a DataFrame by multiple columns. For example, you may want to group the sales data by both the product and region columns.
To do this, you can pass a list of column names to the groupby()
method:
grouped = df.groupby(['product', 'region'])
average_sales = grouped.mean()
This will group the DataFrame by both the “product” and “region” columns and return a new DataFrame that contains the average sales for each combination of product and region:
sales
product region
A North 100.0
South 400.0
B North 200.0
South 500.0
C North 300.0
South 600.0
Conclusion
In this blog post, we explored how to get the average of a groupby with pandas. We learned that groupby is a powerful feature in pandas that allows you to group a DataFrame based on one or more columns and then perform various operations on each group.
We also learned that to get the average of a groupby in pandas, you can use the mean()
method on the GroupBy
object. This method calculates the mean of each numeric column for each group.
Finally, we saw how to group a DataFrame by multiple columns by passing a list of column names to the groupby()
method.
Groupby is a powerful tool in pandas that can help you perform complex data analysis tasks. By understanding how to use groupby and other pandas features, you can become a more effective data scientist or software engineer.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.