How to Get Row Number in Dataframe in Pandas
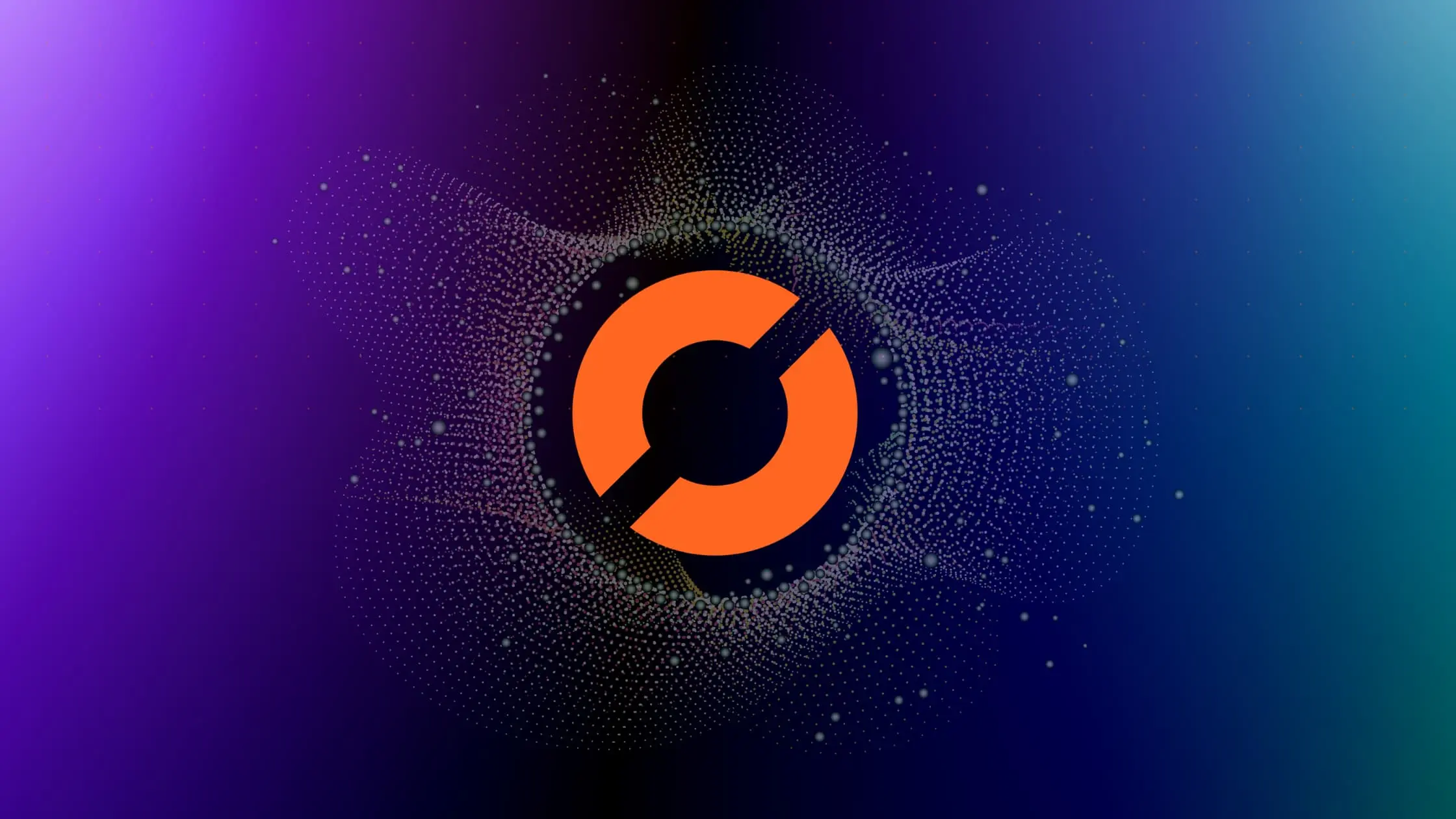
As a data scientist or software engineer working with data, it’s common to need to get the row number in a Pandas dataframe. This can be useful for various reasons such as identifying specific rows, filtering data, or performing calculations on specific rows. In this article, we’ll discuss how to get row number in a dataframe in Pandas.
Table of Contents
- What is Pandas?
- Getting Started with Pandas
- How to Get Row Number in a Pandas Dataframe
- Best Practices
- Common Errors and How to Handle Them
- Conclusion
What is Pandas?
Pandas is a Python library used for data manipulation and analysis. It provides data structures for efficiently storing and manipulating large datasets, as well as tools for data cleaning, preprocessing, and analysis. Pandas is widely used in data science and machine learning projects due to its simplicity and flexibility.
Getting Started with Pandas
Before we dive into how to get the row number in a Pandas dataframe, let’s cover some basics of working with Pandas dataframes.
Installing Pandas
If you haven’t yet installed Pandas, you can do so by running the following command in your terminal:
pip install pandas
Importing Pandas
To use Pandas in your Python code, you need to import it first. You can do so by adding the following line at the beginning of your Python script:
import pandas as pd
How to Get Row Number in a Pandas Dataframe
Now that we have covered the basics of working with Pandas, let’s dive into how to get row number in a Pandas dataframe.
Using the .index Attribute
Pandas dataframes have an .index
attribute that returns the index of each row in the dataframe. The index starts at 0 and increments by 1 for each row in the dataframe.
To get the row number of a specific row in a Pandas dataframe, you can use the .index
attribute along with the .get_loc()
method. The .get_loc()
method returns the integer location of the row in the dataframe’s index.
import pandas as pd
# Create a sample dataframe
data = {'name': ['John', 'Jane', 'Bob', 'Alice'],
'age': [25, 30, 35, 40]}
df = pd.DataFrame(data)
# Get the row number of the row with name 'Bob'
row_number = df.index.get_loc(df[df['name'] == 'Bob'].index[0])
print(row_number)
Output:
2
In the above example, we first create a sample dataframe with two columns, name and age. Then, we use the .index
attribute along with the .get_loc()
method to get the row number of the row with name ‘Bob’. The output of the code is 2, which is the row number of the row with name ‘Bob’.
Using the .iterrows() Method
Another way to get the row number in a Pandas dataframe is to use the .iterrows()
method. The .iterrows()
method returns an iterator that iterates over the rows of the dataframe, yielding the index and row data for each row.
import pandas as pd
# Create a sample dataframe
data = {'name': ['John', 'Jane', 'Bob', 'Alice'],
'age': [25, 30, 35, 40]}
df = pd.DataFrame(data)
# Get the row number of the row with name 'Bob'
for i, row in df.iterrows():
if row['name'] == 'Bob':
row_number = i
break
print(row_number)
Output:
2
Using the .index()
Method
Another approach involves using the .index()
method, which directly returns the row indices matching a given condition.
import pandas as pd
# Create a sample dataframe
data = {'name': ['John', 'Jane', 'Bob', 'Alice'],
'age': [25, 30, 35, 40]}
df = pd.DataFrame(data)
# Get the row number of the row with name 'Bob'
row_number = df.index[df['name'] == 'Bob'][0]
print(row_number)
Best Practices
- Evaluate Performance: Consider the size of your dataframe when choosing a method.
- Avoid Chaining Operations: Be cautious with chaining multiple operations, as it may impact performance.
Common Errors and How to Handle Them
Error 1: Non-Existent Value in .get_loc()
If the specified condition in .get_loc()
does not exist in the dataframe, it may result in an error. Always check for the existence of the condition before using it.
Error 2: Memory Overhead with .iterrows()
Using .iterrows()
for large dataframes can lead to memory overhead. If performance is crucial, consider alternative methods.
Error 3: Incorrect Usage of .index()
Ensure the proper usage of the .index()
method, as incorrect conditions may lead to unexpected results. Always validate the conditions applied.
In the above example, we use the .iterrows()
method to iterate over the rows of the dataframe. For each row, we check if the value in the ‘name’ column is ‘Bob’. If it is, we set row_number
to the index of the row and break out of the loop. The output of the code is 2, which is the row number of the row with name ‘Bob’.
Conclusion
In this article, we have discussed how to get row number in a Pandas dataframe. We covered two methods: using the .index
attribute along with the .get_loc()
method, and using the .iterrows()
method. Both methods are useful for different situations, and it’s important to choose the one that best suits your needs.
Pandas is a powerful library for data manipulation and analysis, and knowing how to get row number in a dataframe is just one of the many useful skills you can learn. We hope this article has been helpful in your data science journey!
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.