How to Get Current CPU GPU and RAM Usage of a Particular Program in Python
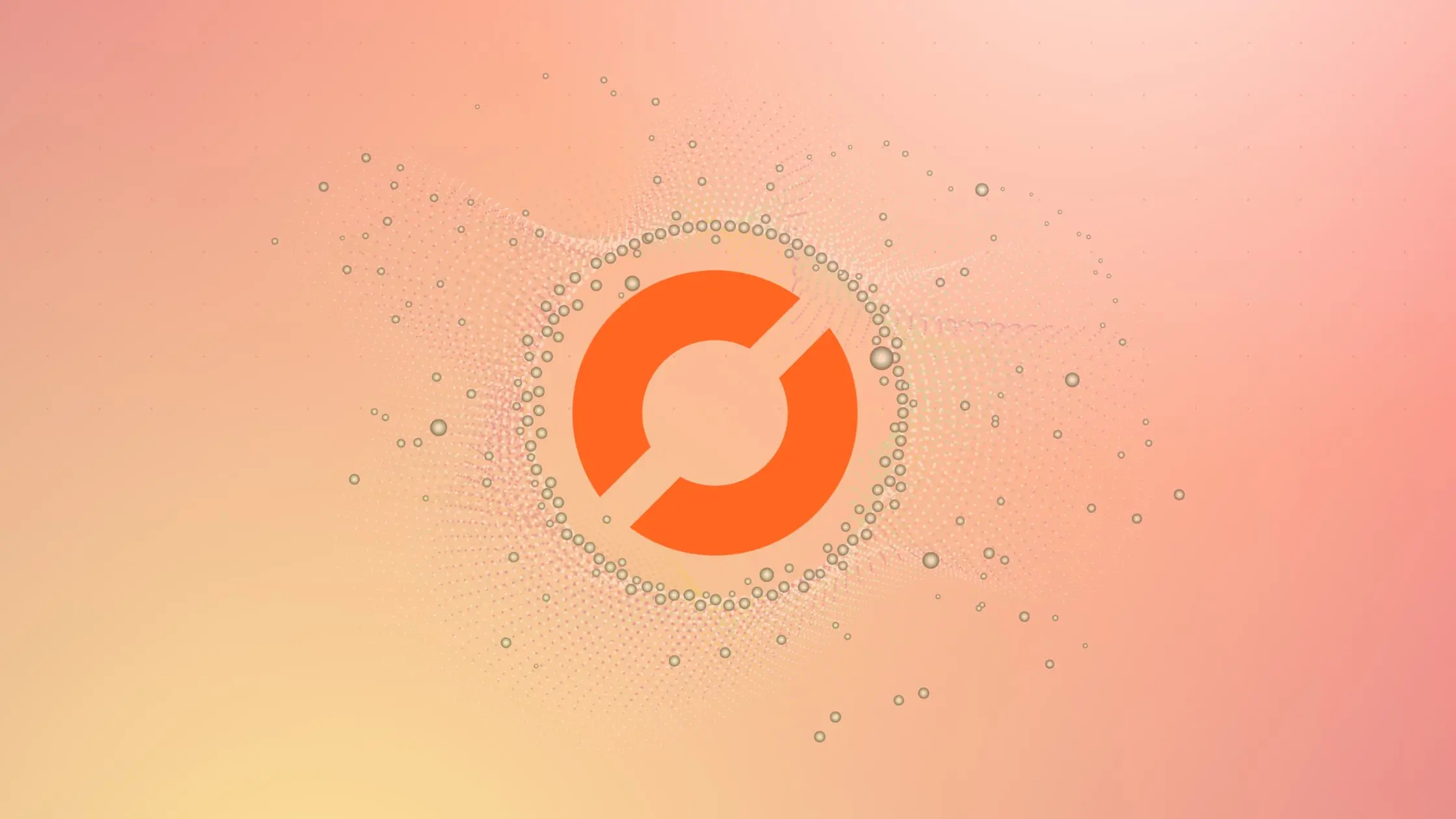
As a data scientist or software engineer, you may need to monitor the resource usage of a particular program in Python. This can help you optimize your code and ensure that it runs efficiently on various devices. In this article, we will explore how to get the current CPU, GPU, and RAM usage of a particular program in Python.
Table of Contents
- Introduction
- Why Monitor Resource Usage?
- Getting Started
- Monitoring CPU Usage
- Monitoring Memory Usage
- Monitoring Disk Usage
- GPU usage part
- Conclusion
Why Monitor Resource Usage?
Before we dive into the technical details, let’s briefly discuss why monitoring resource usage is important.
Firstly, monitoring resource usage can help you optimize your code. By identifying which parts of your program are using the most resources, you can focus on optimizing those areas to improve overall performance.
Secondly, different devices have different hardware configurations, which can impact how your program runs. Monitoring resource usage can help you identify any bottlenecks or issues that may arise on different devices, allowing you to optimize your code accordingly.
Finally, monitoring resource usage can help you identify any memory leaks or other issues that may cause your program to crash or behave unexpectedly.
Getting Started
To get started, we will be using the psutil
library, which provides an easy way to retrieve information about system utilization. You can install psutil
using pip
:
pip install psutil
The psutil
library provides a variety of functions for retrieving information about system utilization, including CPU, memory, and disk usage. We will be using the following functions:
psutil.cpu_percent()
: Returns the current CPU utilization as a percentage.psutil.virtual_memory()
: Returns information about the system’s memory usage.psutil.disk_usage()
: Returns information about disk usage.
Monitoring CPU Usage
To monitor the CPU usage of a particular program, we can use the psutil.cpu_percent()
function. This function returns the current CPU utilization as a percentage, averaged over a specified interval (in seconds).
Here’s an example of how to use the psutil.cpu_percent()
function to monitor the CPU usage of a particular program:
import psutil
import time
while True:
cpu_percent = psutil.cpu_percent(interval=1)
print(f"CPU Usage: {cpu_percent}%")
time.sleep(1)
In this example, we are using a while
loop to continuously monitor the CPU usage of the program. We are calling the psutil.cpu_percent()
function with an interval of 1 second, which means that the function will return the CPU utilization as a percentage averaged over the last second. We are then printing the CPU usage to the console and sleeping for 1 second before repeating the process.
Monitoring Memory Usage
To monitor the memory usage of a particular program, we can use the psutil.virtual_memory()
function. This function returns a namedtuple
that contains information about the system’s memory usage, including the total amount of memory available, the amount of memory used, and the amount of memory free.
Here’s an example of how to use the psutil.virtual_memory()
function to monitor the memory usage of a particular program:
import psutil
import time
while True:
memory_usage = psutil.virtual_memory()
print(f"Memory Usage: {memory_usage.percent}%")
time.sleep(1)
In this example, we are using a while
loop to continuously monitor the memory usage of the program. We are calling the psutil.virtual_memory()
function, which returns a namedtuple
containing information about the system’s memory usage. We are then printing the memory usage percentage to the console and sleeping for 1 second before repeating the process.
Monitoring Disk Usage
To monitor the disk usage of a particular program, we can use the psutil.disk_usage()
function. This function returns a namedtuple
that contains information about the disk usage, including the total amount of disk space, the amount of disk space used, and the amount of disk space free.
Here’s an example of how to use the psutil.disk_usage()
function to monitor the disk usage of a particular program:
import psutil
import time
while True:
disk_usage = psutil.disk_usage('/')
print(f"Disk Usage: {disk_usage.percent}%")
time.sleep(1)
In this example, we are using a while
loop to continuously monitor the disk usage of the program. We are calling the psutil.disk_usage()
function with the root directory ('/'
) as an argument, which returns a
namedtuple
containing information about the disk usage. We are then printing the disk usage percentage to the console and sleeping for 1 second before repeating the process.
GPU usage part
## Import the gpustat library to monitor GPU usage
import gpustat
Here, the gpustat
library is imported, which is a Python library for querying GPU statistics. This library allows you to gather information about the utilization and status of GPUs.
## Get GPU statistics
gpu_stats = gpustat.GPUStatCollection.new_query()
This line creates an instance of the GPUStatCollection
class from the gpustat
library. The new_query()
method is used to obtain the current statistics of all available GPUs.
## Print GPU information
for gpu in gpu_stats.gpus:
print(f"GPU {gpu.index}: {gpu.name}, Utilization: {gpu.utilization}%")
Here, a for
loop is used to iterate over the GPUs obtained from the gpu_stats.gpus attribute. For each GPU, information such as the GPU index, name, and utilization percentage is printed to the console.
gpu.index
: Represents the index or identifier of the GPU.gpu.name
: Represents the name or model of the GPU.gpu.utilization
: Represents the GPU utilization percentage.
Conclusion
In summary, the article explores how to monitor the CPU, GPU, and RAM usage of a Python program using the psutil
library. Delving into technical details, the author emphasizes the importance of this monitoring to optimize code, address issues related to diverse hardware configurations, and ensure efficient program operation. The use of the gpustat
library to monitor GPU is also introduced. By adopting these monitoring methods, developers can enhance their code’s performance and ensure broader compatibility with different devices.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.