How to Format Certain Floating Dataframe Columns into Percentage in Pandas
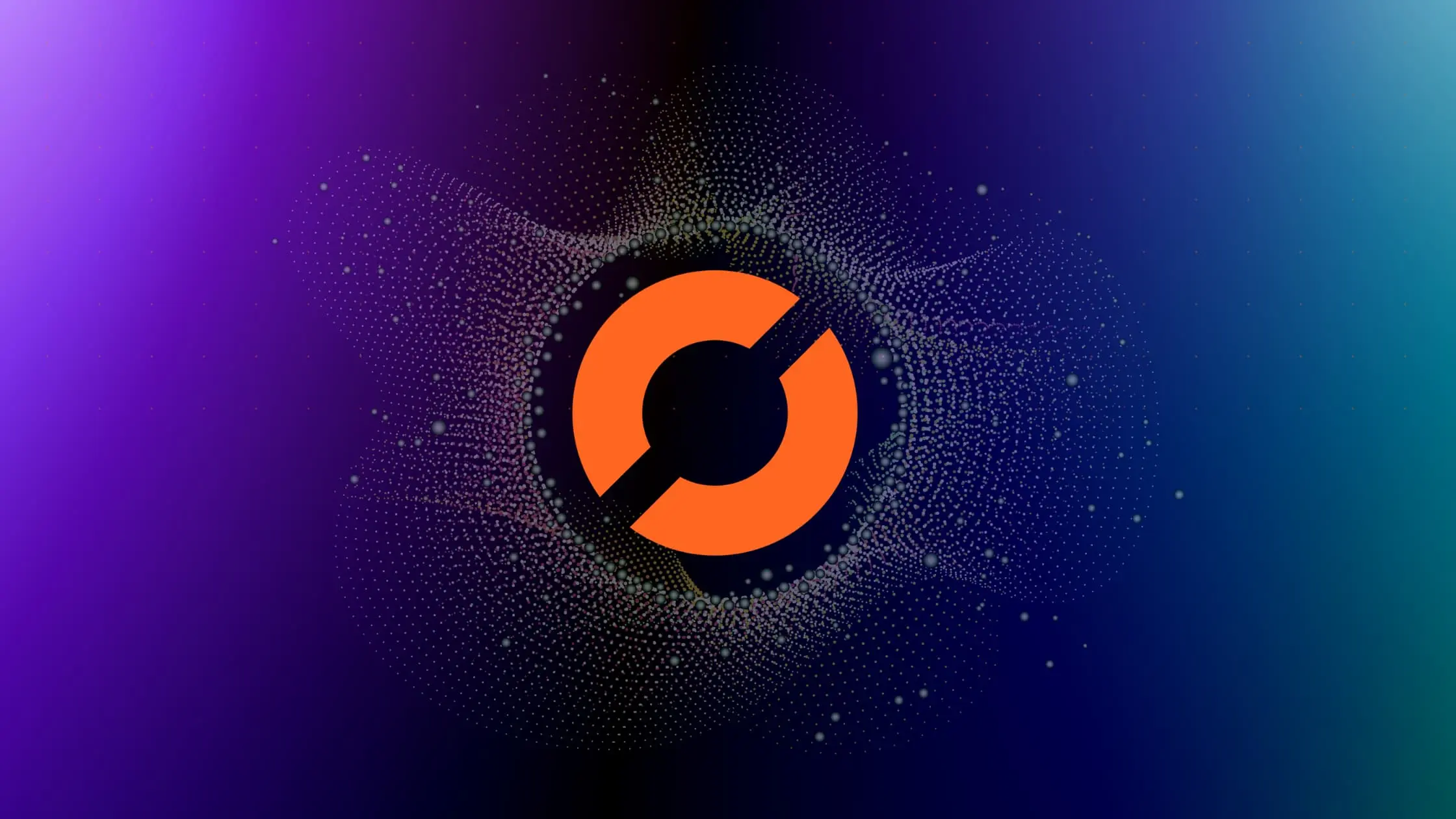
As a data scientist, one of the most important tasks you will encounter is formatting your data in a way that is easy to read and understand. This is especially true when working with dataframes in Pandas. In this article, we will discuss how to format certain floating dataframe columns into percentages in Pandas.
Table of Contents
- What is Pandas?
- Formatting Floating Dataframe Columns into Percentages
- Pros and Cons
- Common Errors and How to Handle Them
- Conclusion
What is Pandas?
Pandas is a popular Python library used for data manipulation and analysis. It provides developers with a powerful set of tools for working with tabular data, including dataframes and series objects. Pandas allows you to manipulate, slice, and aggregate data in a variety of ways, making it an essential tool for data scientists and software engineers alike.
Formatting Floating Dataframe Columns into Percentages
When working with dataframes in Pandas, you may find that some of your columns contain floating-point values that represent percentages. While these values may be accurate, they can be difficult to read and interpret. Fortunately, Pandas provides a simple solution for formatting these columns into percentages.
To format a floating dataframe column into a percentage in Pandas, you can use the map
and format
methods. Let’s start by setting up a scenario with a sample DataFrame:
import pandas as pd
# Creating a sample DataFrame
data = {
'Product': ['A', 'B', 'C'],
'Price': [1500.25, 2300.50, 1800.75],
'Discount': [0.05, 0.10, 0.15]
}
df = pd.DataFrame(data)
print("Original DataFrame:")
print(df)
Product Price Discount
0 A 1500.25 0.05
1 B 2300.50 0.10
2 C 1800.75 0.15
In this example, we start by importing the Pandas library and creating a sample dataframe with two columns: Product
, Price
and Discount
. The Discount
column contains floating-point values that represent percentages.
To format the Discount
column as a percentage, we use the map
method to apply a formatting string to each value in the column. The formatting string '{:.2%}'
specifies that we want to format each value as a percentage with two decimal places. The format
method then applies this string to each value in the column.
# format the Score column as a percentage
df['Discount'] = df['Discount'].map('{:.2%}'.format)
# display the formatted dataframe
print(df)
Product Price Discount
0 A 1500.25 5.00%
1 B 2300.50 10.00%
2 C 1800.75 15.00%
As you can see, the Discount
column has been formatted as percentages with two decimal places. This makes it much easier to read and understand the data.
Pros and Cons of Using .map('{:.2%}'.format)
Pros:
- Simplicity: The .map('{:.2%}'.format) method is straightforward and easy to implement.
- Readability: The code is concise and enhances the readability of your data processing pipeline.
Cons:
- Limited Applicability: This method is primarily suitable for formatting individual columns, and may not be as versatile for complex formatting requirements.
- Not In-Place: The operation creates a new Series, so it is important to assign the result back to the original DataFrame column.
Common Errors and How to Handle Them
Error 1: TypeError
Error Message:
TypeError: unsupported format string passed to Series.__format__
Cause:
This error occurs when attempting to apply the .map('{:.2%}'.format)
method to a column that may not contain numerical values or has mixed data types.
Solution:
Ensure that the column contains numerical values. If necessary, convert the column to a numeric type using pd.to_numeric
. This function attempts to convert the entire column to numeric values and raises an error if it encounters non-numeric elements.
Example:
import pandas as pd
# Creating a DataFrame with a column containing non-numeric values
data = {'column_name': ['0.123', 0.456, 'text']}
df = pd.DataFrame(data)
# Convert 'column_name' to numeric, handling errors with 'coerce'
df['column_name'] = pd.to_numeric(df['column_name'], errors='coerce')
# Now apply the percentage formatting
df['column_name'] = df['column_name'].map('{:.2%}'.format)
Error 2: AttributeError
Error Message:
AttributeError: 'DataFrame' object has no attribute 'map'
Cause:
This error occurs when attempting to use the .map('{:.2%}'.format)
method directly on a DataFrame instead of a Series.
Solution:
Ensure that you are applying the .map('{:.2%}'.format)
method to a Pandas Series, not a DataFrame. Select the column using df['column_name']
before applying the method. The .map()
method is not available at the DataFrame level.
Example:
import pandas as pd
# Creating a DataFrame
data = {'column_name': [0.123, 0.456, 0.789]}
df = pd.DataFrame(data)
# Incorrect usage, resulting in an AttributeError
# df = df.map('{:.2%}'.format) # This line will raise an error
# Correct usage: Select the column and apply the .map method
df['column_name'] = df['column_name'].map('{:.2%}'.format)
Conclusion
Formatting floating dataframe columns into percentages in Pandas is a simple but important task for data scientists and software engineers. By using the map
and format
methods, you can easily format your data in a way that is easy to read and understand.
I hope this article has been helpful in explaining how to format certain floating dataframe columns into percentages in Pandas. If you have any questions or comments, please feel free to leave them below.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.