How to Fix the NoneType object is not iterable Error in Pandas
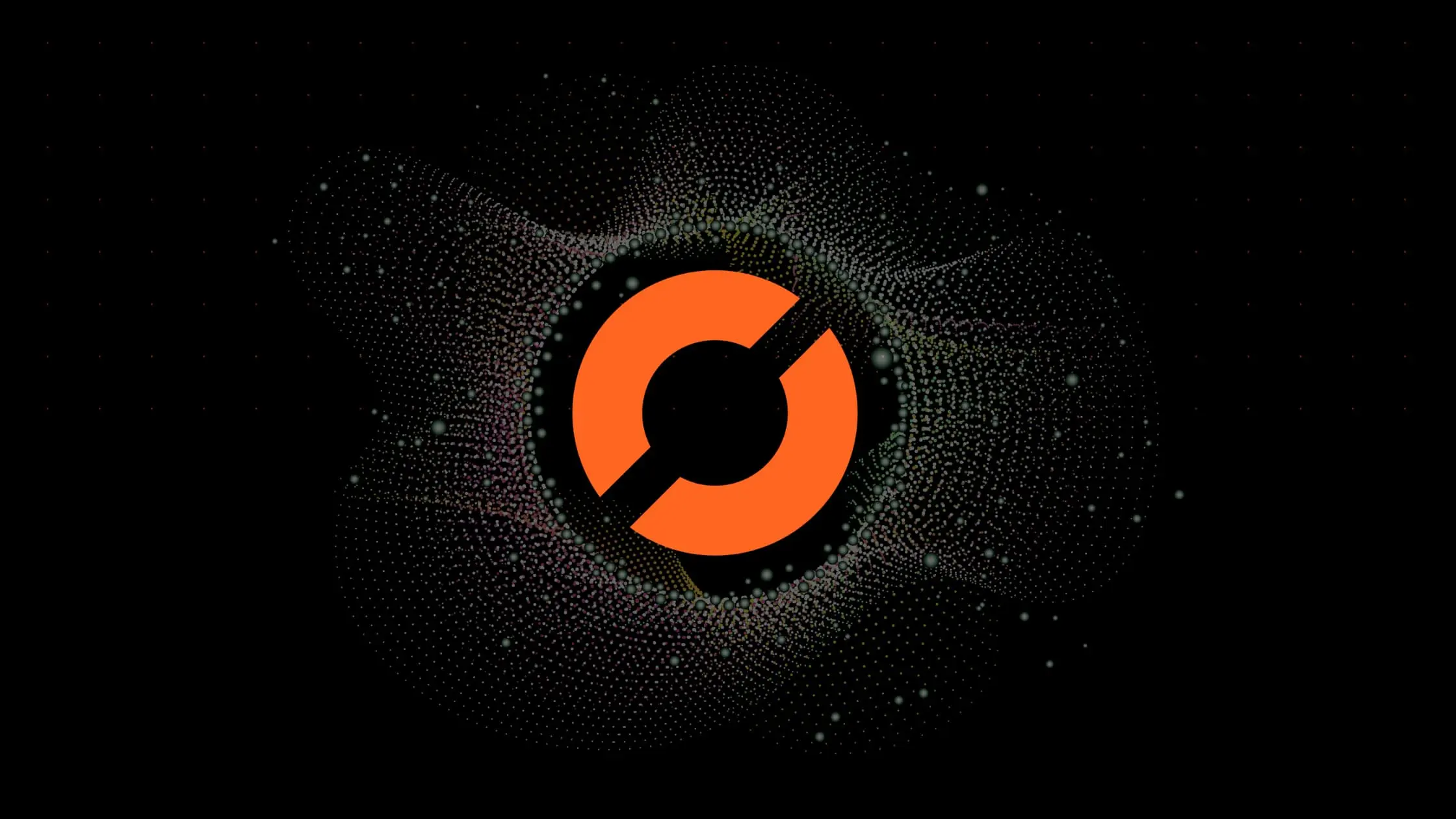
If you’re a data scientist or software engineer who works with Python programming language, then chances are you’ve encountered the “NoneType object is not iterable” error in Pandas. This error can be frustrating and can cause your code to break, preventing you from analyzing your data effectively.
In this article, we’ll explain what this error means and offer solutions to help you fix it. Whether you’re a beginner or seasoned programmer, you’ll find this guide helpful in resolving this common issue.
Table of Contents
- What is the
"NoneType object is not iterable"
Error in Pandas? - How to Fix the
"NoneType object is not iterable"
Error in Pandas - Best Practices to Avoid the Error
- Conclusion
What is the "NoneType object is not iterable"
Error in Pandas?
The "NoneType object is not iterable"
error in Pandas occurs when you try to iterate over a variable that has a None value. In other words, you are trying to loop through something that doesn’t exist, causing the error to occur.
For example, let’s say you have a Pandas DataFrame called df
and you want to iterate through its rows using a for loop. If the DataFrame is empty, then the df.iterrows()
method will return None, causing the "NoneType object is not iterable"
error to occur.
How to Fix the "NoneType object is not iterable"
Error in Pandas
There are several ways to fix the "NoneType object is not iterable"
error in Pandas. Here are some common solutions:
Solution 1: Check if the Variable is None
The first solution is to check if the variable is None before iterating over it. You can do this using an if statement.
if variable is not None:
# Iterate over the variable
Using this solution, you can avoid iterating over variables that have None values, preventing the error from occurring.
Solution 2: Check if the DataFrame is Empty
If you’re working with a Pandas DataFrame, you can check if it’s empty before iterating over it. You can do this using the empty
attribute.
if not df.empty:
for index, row in df.iterrows():
# Do something with each row
By checking if the DataFrame is empty before iterating over it, you can prevent the "NoneType object is not iterable"
error from occurring.
Solution 3: Use a Try-Except Block
Another solution to fix the "NoneType object is not iterable"
error in Pandas is to use a try-except block. This solution allows you to catch the error and handle it gracefully.
try:
for index, row in df.iterrows():
# Do something with each row
except TypeError:
pass
Using a try-except block, you can catch the TypeError that occurs when trying to iterate over a None value and handle it appropriately.
Solution 4: Use the dropna() Method
If you’re working with a Pandas DataFrame that contains missing values (NaN), you can use the dropna()
method to remove them before iterating over the DataFrame.
df.dropna(inplace=True)
for index, row in df.iterrows():
# Do something with each row
By removing the missing values before iterating over the DataFrame, you can prevent the "NoneType object is not iterable"
error from occurring.
Best Practices to Avoid the Error
Data Cleaning and Handling Missing Values
Ensure your data is clean and handle missing values appropriately before performing any iterations. Consider using methods like dropna() or fillna() to manage missing values.
Validating Data Before Iterating
Before iterating, validate the data type and structure to avoid unexpected None values. Use conditional statements to check for potential issues before starting the iteration process.
Defensive Programming
Implement defensive programming techniques, such as try-except blocks, to catch and handle exceptions gracefully. This can prevent the script from crashing when encountering unexpected situations.
Conclusion
The "NoneType object is not iterable"
error in Pandas can be frustrating, but it’s a common issue that can be easily fixed. By checking if the variable is None, checking if the DataFrame is empty, using a try-except block, or using the dropna()
method, you can prevent this error from occurring and continue analyzing your data effectively.
As a data scientist or software engineer, it’s important to be aware of these common errors and how to fix them. With these solutions in mind, you can confidently work with Pandas and avoid this error in the future.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.