How to Find Unique Values in a Pandas Dataframe Irrespective of Row or Column Location
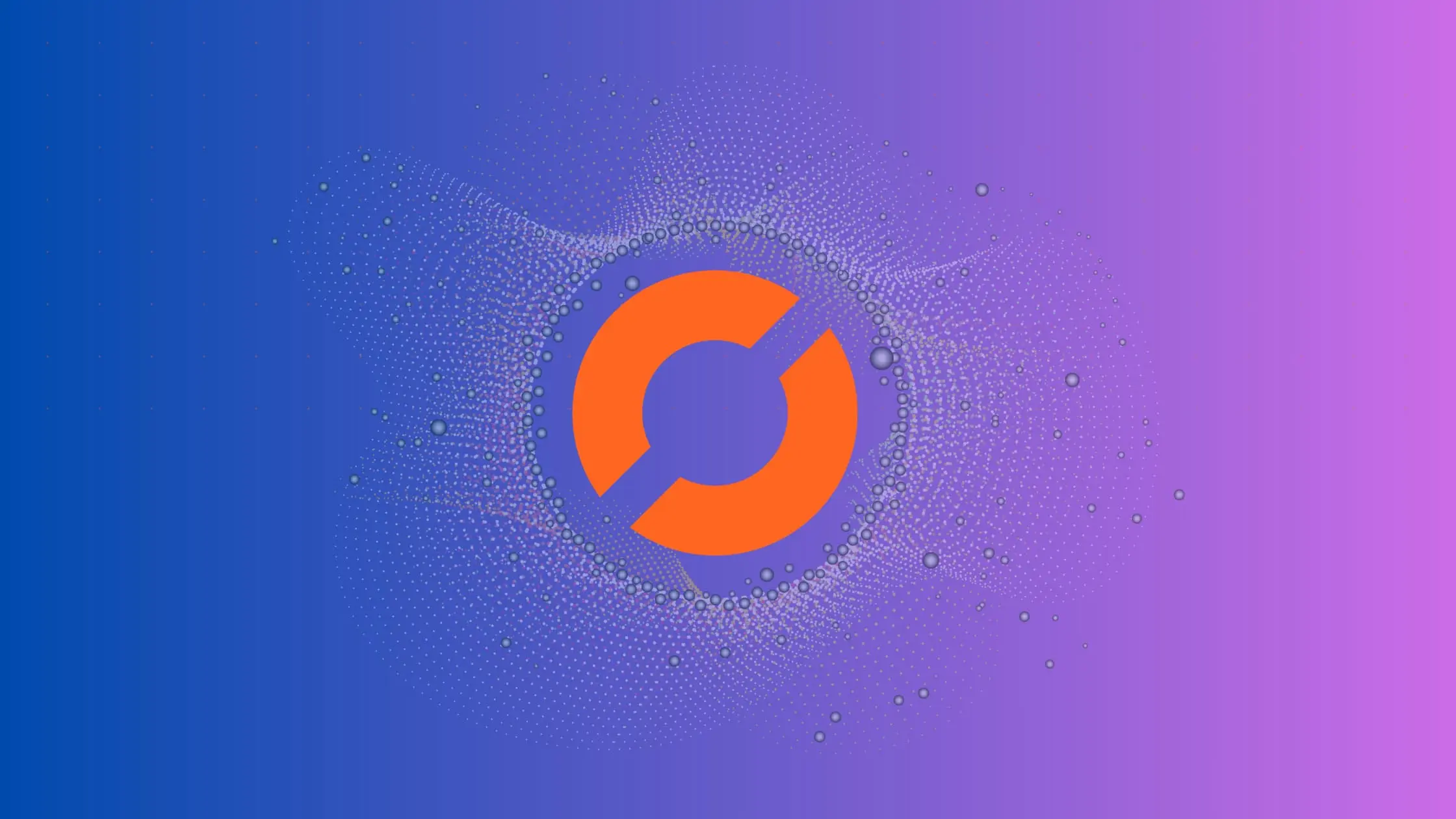
As a data scientist or software engineer, you will often work with large datasets where you need to find unique values irrespective of their location in the dataframe. This could be to identify outliers, clean data, or perform other data analysis tasks. In this article, we will explore how to find unique values in a Pandas dataframe, irrespective of row or column location.
What is Pandas?
Pandas is a popular open-source library in Python used for data manipulation and data analysis. It provides data structures and functions for efficient data handling and analysis. Pandas is widely used in data science and machine learning for data preprocessing, cleaning, and analysis.
Finding Unique Values in a Pandas Dataframe
To find unique values in a Pandas dataframe, we can use the unique()
function. This function returns an array of unique values in the dataframe. However, this function only returns unique values within a particular column or row. To find unique values irrespective of their location in the dataframe, we need to use a different approach.
Using stack() and unique()
One way to find unique values irrespective of their location in the dataframe is to stack the dataframe and then use the unique()
function. Stacking a dataframe means converting the columns into rows, which creates a multi-level index. We can then use the unique()
function to get the unique values from the new index.
Let’s take an example dataframe:
import pandas as pd
df = pd.DataFrame({
'A': [1, 2, 3, 4, 5],
'B': [6, 7, 8, 9, 10],
'C': [1, 2, 3, 4, 5],
'D': [6, 7, 8, 9, 10]
})
This dataframe looks like:
A B C D
0 1 6 1 6
1 2 7 2 7
2 3 8 3 8
3 4 9 4 9
4 5 10 5 10
To find the unique values in this dataframe, we can stack it using the stack()
function:
stacked_df = df.stack()
This creates a new dataframe with a multi-level index:
0 A 1
B 6
C 1
D 6
1 A 2
B 7
C 2
D 7
2 A 3
B 8
C 3
D 8
3 A 4
B 9
C 4
D 9
4 A 5
B 10
C 5
D 10
We can then use the unique()
function to get the unique values from the new index:
unique_values = stacked_df.unique()
This returns an array of unique values in the dataframe, irrespective of their location:
array([ 1, 6, 2, 7, 3, 8, 4, 9, 5, 10])
Using melt() and unique()
Another way to find unique values in a Pandas dataframe irrespective of their location is to use the melt()
function. The melt()
function unpivots a dataframe from wide format to long format, creating a new dataframe with a variable and value column.
Let’s take the same example dataframe as before:
import pandas as pd
df = pd.DataFrame({
'A': [1, 2, 3, 4, 5],
'B': [6, 7, 8, 9, 10],
'C': [1, 2, 3, 4, 5],
'D': [6, 7, 8, 9, 10]
})
This dataframe looks like:
A B C D
0 1 6 1 6
1 2 7 2 7
2 3 8 3 8
3 4 9 4 9
4 5 10 5 10
We can use the melt()
function to unpivot the dataframe:
melted_df = pd.melt(df)
This creates a new dataframe with variable and value columns:
variable value
0 A 1
1 A 2
2 A 3
3 A 4
4 A 5
5 B 6
6 B 7
7 B 8
8 B 9
9 B 10
10 C 1
11 C 2
12 C 3
13 C 4
14 C 5
15 D 6
16 D 7
17 D 8
18 D 9
19 D 10
We can then use the unique()
function to get the unique values from the value column:
unique_values = melted_df['value'].unique()
This returns an array of unique values in the dataframe, irrespective of their location:
array([ 1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
Handling NaN Values in a Pandas Dataframe
In real-world datasets, it’s common to encounter missing or NaN (Not a Number) values. Dealing with NaN values is a crucial aspect of data preprocessing, and it’s essential to address them before performing any analysis. As a data scientist or software engineer working with Pandas dataframes, there are several techniques to handle NaN values. In this section, we’ll discuss two common methods: interpolation and fillna.
Interpolation
Interpolation is a method to estimate missing values based on the known values in a dataframe. Pandas provides the interpolate()
function, which can be used to fill NaN values by computing intermediate values using various interpolation methods such as linear, polynomial, or time-based methods.
Here’s an example of using linear interpolation on a dataframe:
import pandas as pd
# Assume df is your dataframe with NaN values
df.interpolate(method='linear', inplace=True)
In the above code, the interpolate()
function is applied to fill NaN values using linear interpolation. The method
parameter can be adjusted based on the interpolation technique you want to use.
Fillna
The fillna()
function in Pandas allows you to fill NaN values with a specified constant or values derived from other parts of the dataframe. This method provides flexibility in customizing how NaN values are replaced.
# Assume df is your dataframe with NaN values
df.fillna(value=0, inplace=True)
In this example, NaN values are filled with the constant value 0. You can replace NaN values with the mean, median, or any other relevant value based on your specific use case.
Drop NaN Values
If the presence of NaN values doesn’t significantly impact your analysis, you may choose to simply drop rows or columns containing NaN values using the dropna()
function:
# Drop rows with NaN values
df.dropna(inplace=True)
# Drop columns with NaN values
df.dropna(axis=1, inplace=True)
Keep in mind that dropping rows or columns may lead to a loss of valuable information, so it should be done judiciously based on the nature of your data.
Forward or Backward Fill
You can propagate non-NaN values forward or backward to fill NaN values using the ffill()
and bfill()
methods:
# Forward fill NaN values
df.ffill(inplace=True)
# Backward fill NaN values
df.bfill(inplace=True)
This is especially useful in time-series data, where values from the previous or next time points can be used to fill missing values.
Custom Functions
Depending on the specifics of your dataset, you might implement custom functions to handle NaN values in a way that is most meaningful for your analysis.
# Custom function to replace NaN with a specific value
def custom_fillna(col):
# Implement your custom logic here
return col.fillna(custom_value)
# Apply the custom function to selected columns
df['column_name'] = custom_fillna(df['column_name'])
Custom functions allow you to tailor the handling of NaN values based on your domain knowledge and the characteristics of your data.
Choosing the appropriate method depends on the nature of your data, the specific requirements of your analysis, and the impact of handling missing values on the overall integrity of your dataset.
Conclusion
In this article, we explored two ways to find unique values in a Pandas dataframe, irrespective of row or column location. We used the stack()
and unique()
functions to stack the dataframe and get the unique values from the new index, and we used the melt()
and unique()
functions to unpivot the dataframe and get the unique values from the value column. These methods are useful when you need to identify outliers, clean data, or perform other data analysis tasks. With these techniques, you can easily find unique values in your Pandas dataframe, no matter where they are located.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.