How to Find the Index of a Value Anywhere in a Pandas DataFrame
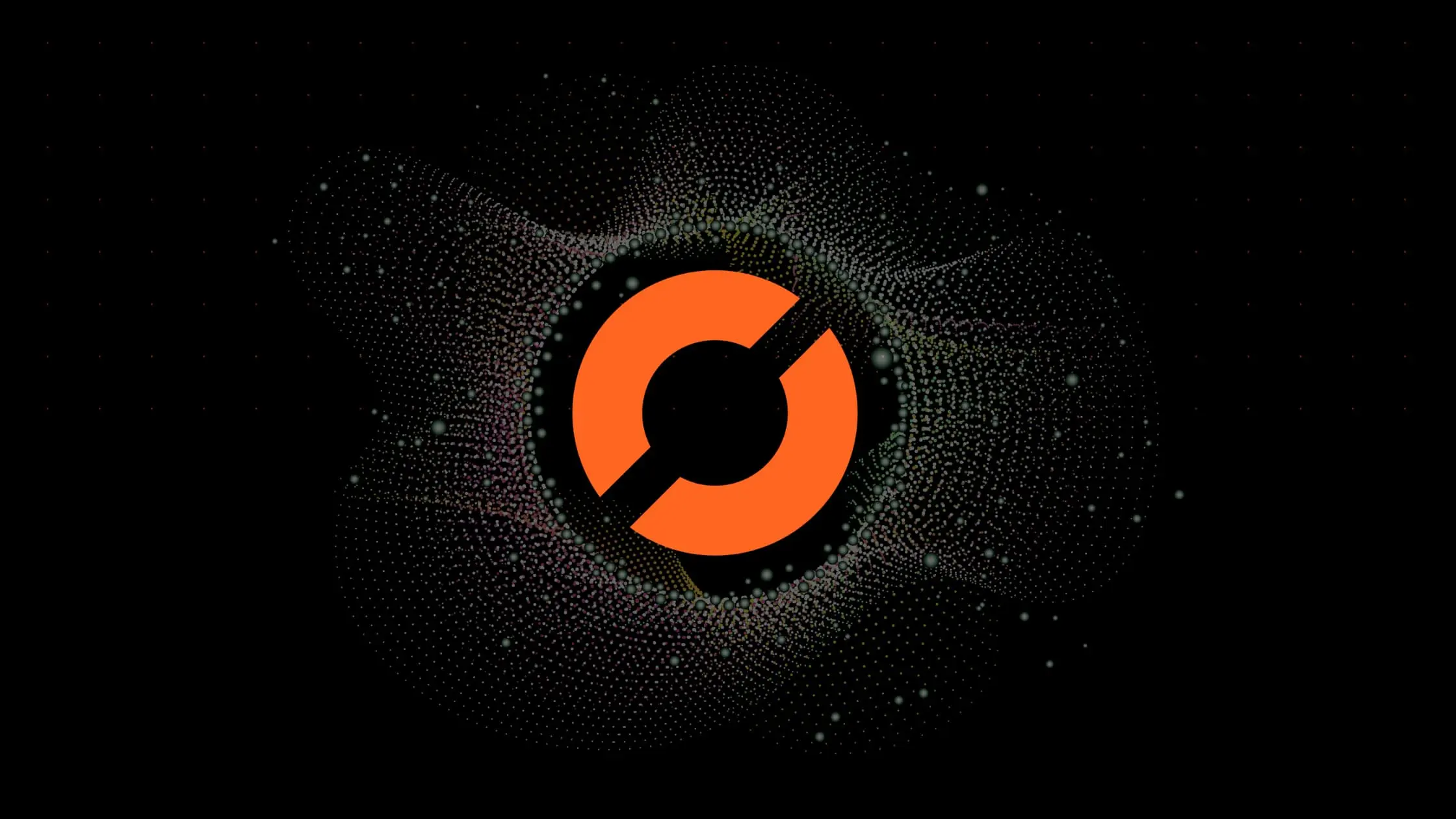
Table of Contents
- 2.1 Using DataFrame.isin() and DataFrame.any()
- 2.2 Using DataFrame.loc[] and any()
- 2.3 Using numpy.where()
Introduction to Pandas
Pandas is a powerful open-source data manipulation tool for Python. It is built on top of the NumPy library and is used for data analysis, data cleaning, and data visualization tasks. Pandas provides two primary classes for working with data: Series and DataFrame. A Series is a one-dimensional array-like object that can hold any data type, while a DataFrame is a two-dimensional tabular data structure with rows and columns.
How to Find the Index of a Value Anywhere in a Pandas DataFrame
To find the index of a value anywhere in a Pandas DataFrame, we can use several methods. We will explore them below:
Using DataFrame.isin() and DataFrame.any()
The DataFrame.isin()
method returns a Boolean DataFrame showing whether each element in the DataFrame is contained in the passed sequence of values. The DataFrame.any()
method then returns a Boolean Series indicating whether any value in each row is True. We can then use the Series.idxmax()
method to find the index of the first occurrence of the maximum value in the Series.
Here is an example of how to find the index of a value anywhere in a Pandas DataFrame:
import pandas as pd
# create a sample DataFrame
data = {'name': ['Alice', 'Bob', 'Charlie', 'David'],
'age': [25, 30, 35, 40],
'city': ['New York', 'Los Angeles', 'Chicago', 'Houston']}
df = pd.DataFrame(data)
# find the index of the first occurrence of 'Charlie' in the DataFrame
index = df.isin(['Charlie']).any(axis=1).idxmax()
print(index)
Output:
2
In this example, we create a sample DataFrame with columns for name, age, and city. We then use the DataFrame.isin()
method to check whether each element in the DataFrame is equal to ‘Charlie’, which returns a Boolean DataFrame. We then use the DataFrame.any()
method to check whether any value in each row is True, which returns a Boolean Series. Finally, we use the Series.idxmax()
method to find the index of the first occurrence of the maximum value in the Series.
Using DataFrame.loc[] and any()
You can use the DataFrame.loc[]
method along with the any()
method to find the index of a value anywhere in a Pandas DataFrame:
Here is an example of how to execute this in Python:
import pandas as pd
# create a sample DataFrame
data = {'name': ['Alice', 'Bob', 'Charlie', 'David'],
'age': [25, 30, 35, 40],
'city': ['New York', 'Los Angeles', 'Chicago', 'Houston']}
df = pd.DataFrame(data)
# find the index of the first occurrence of 'Charlie' in the DataFrame
index = df.loc[(df == 'Charlie').any(axis=1)].index[0]
print(index)
Output:
2
In this method, we use the DataFrame.loc[]
method to select rows where any column is equal to ‘Charlie’. The resulting DataFrame contains True for rows that meet the condition and False otherwise. Then, we use the any(axis=1)
to check if any value in each row is True. Finally, we use idxmax()
to find the index of the first occurrence of True in the resulting Series.
Using numpy.where()
You can leverage the numpy.where()
function to find the index of a value anywhere in a Pandas DataFrame:
import pandas as pd
import numpy as np
# create a sample DataFrame
data = {'name': ['Alice', 'Bob', 'Charlie', 'David'],
'age': [25, 30, 35, 40],
'city': ['New York', 'Los Angeles', 'Chicago', 'Houston']}
df = pd.DataFrame(data)
# find the index of the first occurrence of 'Charlie' in the DataFrame
index = np.where(df == 'Charlie')[0][0]
print(index)
Output:
2
In this method, numpy.where()
returns a tuple of arrays. The first array contains the row indices where the condition is True. We use [0][0]
to extract the first occurrence of the index from the tuple.
Common Errors
Misunderstanding axis argument: Be cautious with the axis argument in
isin()
andany()
. Specifyaxis=1
for searching across columns in each row, andaxis=0
for searching across all rows for a specific value.Indexing non-existent values: Ensure the value you’re searching for actually exists in the DataFrame. Otherwise, methods like
idxmax()
might raise errors. For better practice you migh conside using atry-except
phrase in case you encounter non-existent values errors.
Pros and Cons
DataFrame.isin() and DataFrame.any():
- Pros: Easy to understand and implement, good for checking multiple values.
- Cons: Less efficient for large DataFrames, might require extra steps for individual index retrieval.
DataFrame.loc[] and any():
- Pros: Efficient for large DataFrames, provides direct access to rows matching the condition.
- Cons: More complex syntax, potentially less intuitive for beginners.
numpy.where():
- Pros: Extremely efficient for large DataFrames, concise and powerful.
- Cons: Requires familiarity with NumPy syntax, less readable than Pandas methods for some users.
Conclusion
In conclusion, finding the index of a value anywhere in a Pandas DataFrame can be a time-consuming task if done manually. Thankfully, Pandas provides some easy and efficient ways to accomplish this task using the DataFrame.isin()
, DataFrame.loc[]
and numpy.where()
methods. By using these methods, we can quickly locate the index of a specific value in a Pandas DataFrame, saving us valuable time and effort in our data analysis tasks.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.