How to Find Elements Index in Pandas Series
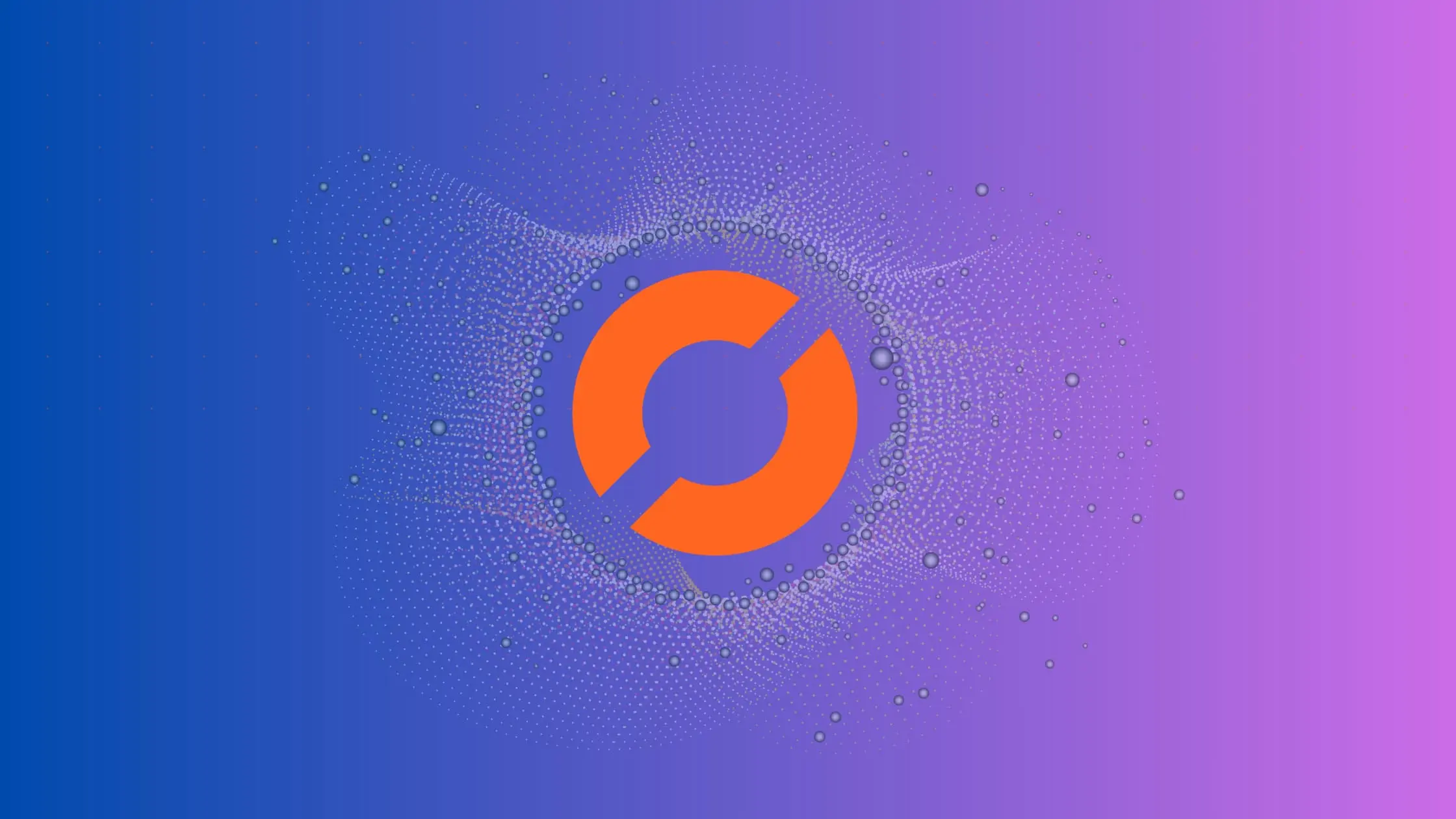
As a data scientist or software engineer, you may frequently work with data in the form of Pandas Series. Pandas is a popular data manipulation library in Python that provides powerful data structures and functions for working with tabular and time-series data. In this article, we will explore how to find the index of an element in a Pandas Series.
Table of Contents
- What is a Pandas Series?
- Finding the Index of an Element in a Pandas Series
- Common Errors and Solutions
- Conclusion
What is a Pandas Series?
A Pandas Series is a one-dimensional array-like object that can hold different types of data, such as integers, floats, strings, and even Python objects. Each element in a Series has a unique label or index that allows us to access and manipulate the data. We can create a Pandas Series from a list, tuple, or dictionary using the pd.Series()
constructor. Here’s an example:
import pandas as pd
data = [3, 2, 1, 4, 5]
series = pd.Series(data)
print(series)
Output:
0 3
1 2
2 1
3 4
4 5
dtype: int64
As we can see, the Series object has both a unique index and a corresponding value for each element.
Finding the Index of an Element in a Pandas Series
There are several ways to find the index of an element in a Pandas Series. Let’s explore some of the most common methods below.
Method 1: Using the index()
Method
The simplest way to find the index of an element in a Pandas Series is to use the index()
method. This method returns the index label for the first occurrence of the specified value. Here’s an example:
import pandas as pd
data = [3, 2, 1, 4, 5]
series = pd.Series(data)
# get index of element 4
index = list(series).index(4)
print(index)
Output:
3
In this example, we first created a Pandas Series from a list of integers. We then used the index()
method to find the index of the value 4
after converting the series to list. The method returned the index label 3
, which corresponds to the fourth element in the Series.
Method 2: Using Boolean Indexing
Another way to find the index of an element in a Pandas Series is to use Boolean indexing. This involves creating a Boolean mask that identifies the elements in the Series that match the specified value and then extracting the corresponding index labels. Here’s an example:
import pandas as pd
data = [3, 2, 1, 4, 5]
series = pd.Series(data)
# get the mask
mask = (series == 4)
# use mask to find index
index = series.index[mask]
print(index)
Output:
Int64Index([3], dtype='int64')
In this example, we first created a Pandas Series from a list of integers. We then created a Boolean mask that is True
for the element with value 4
and False
for all other elements. We applied the mask to the index of the Series using the square bracket notation, which returned an Int64Index
object containing the index label 3
.
Method 3: Using the get_loc()
Method
The most efficient way to find the index of an element in a Pandas Series is to use the get_loc()
method. This method returns the integer location of the specified value in the Series, which is the same as the index label for most Series objects. Here’s an example:
import pandas as pd
data = [3, 2, 1, 4, 5]
series = pd.Series(data)
# get index using get_loc
index = pd.Index(series).get_loc(4)
print(index)
Output:
3
In this example, we first created a Pandas Series from a list of integers. We then used the get_loc()
method to find the index label for the value 4
. The method returned the integer location 3
, which corresponds to the fourth element in the Series.
Sure, let’s add sections for common errors and solutions, as well as best practices to the article.
Common Errors and Solutions
Error 1: Using index()
method on Non-Existent Value
Code:
import pandas as pd
data = [3, 2, 1, 4, 5]
series = pd.Series(data)
# Trying to find index of value that doesn't exist
index = list(series).index(6)
Error:
ValueError: 6 is not in list
Solution:
The index()
method is not meant for finding the index of a value directly; it is used to get the index object. To find the index of a value, use methods like boolean indexing or get_loc()
.
Error 2: Using get_loc()
on Non-Existent Value
Code:
import pandas as pd
data = [3, 2, 1, 4, 5]
series = pd.Series(data)
# Using get_loc() on a value that doesn't exist
index = pd.Index(series).get_loc(6)
Error:
KeyError: 6
Solution:
The get_loc()
method raises a KeyError
if the specified value is not present in the Series. To avoid this error, you can check if the value exists in the Series before using get_loc()
, or use alternative methods like boolean indexing.
Conclusion
In this article, we have explored three different methods for finding the index of an element in a Pandas Series. These methods include using the index()
method, Boolean indexing, and the get_loc()
method. Depending on the size and complexity of your data, one method may be more efficient than the others. By understanding these methods, you can extract and manipulate data in Pandas Series with greater ease and efficiency.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.