How to Filter Pandas DataFrame with Specific Column Names in Python
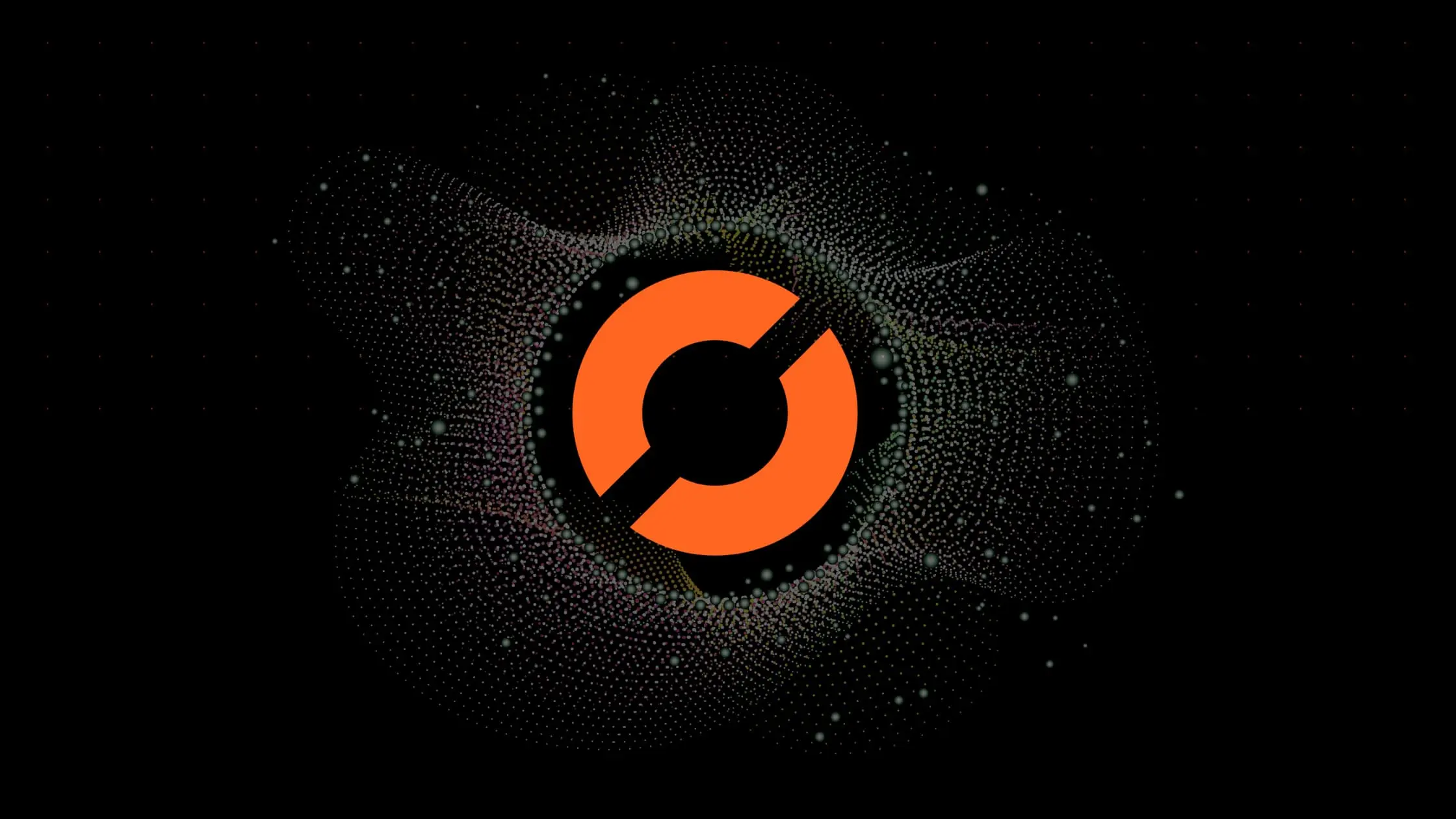
As a data scientist or software engineer, you know that data manipulation is an essential part of any data analysis project. One of the most common tasks in data manipulation is filtering a pandas DataFrame based on specific column names. In this tutorial, we will cover the basics of how to filter a pandas DataFrame with specific column names in Python.
What is Pandas DataFrame?
The Pandas library provides a powerful data structure called DataFrame, which is a two-dimensional table that contains rows and columns. Each column can have a different data type, such as integer, float, or string.
Pandas DataFrame is widely used in data analysis and data manipulation tasks due to its flexibility and ease of use. It provides a variety of functions and methods that allow you to perform complex data manipulations with ease.
Filtering Pandas DataFrame with Specific Column Names
Filtering a pandas DataFrame with specific column names is a common task in data analysis. For example, you may want to filter a DataFrame to only include specific columns that are relevant to your analysis.
Using Square Brackets
One of the most straightforward methods to filter a Pandas DataFrame is by using square brackets to select columns of interest. For instance, consider a DataFrame named df with columns Name
, Age
, Gender
, and Salary
:
import pandas as pd
data = {'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35],
'Gender': ['Female', 'Male', 'Male'],
'Salary': [50000, 60000, 75000]}
df = pd.DataFrame(data)
To filter columns based on specific names, you can use square brackets as follows:
selected_columns = df[['Name', 'Age']]
print(selected_columns)
Output:
Name Age
0 Alice 25
1 Bob 30
2 Charlie 35
Using the filter
Method
The filter
method in Pandas allows for more advanced column selection based on specific criteria. You can specify the columns to include or exclude using wildcards or regular expressions.
selected_columns = df.filter(items=['Name', 'Gender'])
print(selected_columns)
Output:
Name Gender
0 Alice Female
1 Bob Male
2 Charlie Male
Using the loc
Method
The loc
method in Pandas is primarily used for label-based indexing, but it can also be employed to filter columns based on their names.
selected_columns = df.loc[:, ['Name', 'Salary']]
print(selected_columns)
Output:
Name Salary
0 Alice 50000
1 Bob 60000
2 Charlie 75000
Here, loc[:, ['Name', 'Salary']]
selects all rows (:) and only the specified columns ('Name' and 'Salary')
.
Using List Comprehension
For more flexibility and customization, you can use list comprehension to filter columns dynamically based on specific criteria.
desired_columns = ['Name', 'Gender']
selected_columns = df[[col for col in df.columns if col in desired_columns]]
print(selected_columns)
Output:
Name Gender
0 Alice Female
1 Bob Male
2 Charlie Male
Conclusion
In conclusion, Pandas provides multiple methods to filter a DataFrame based on specific column names, catering to different use cases and preferences. Whether you prefer the simplicity of square brackets or the flexibility of the filter
method, Pandas offers a solution for every data manipulation need. Choose the method that best suits your requirements and enhances the efficiency of your data analysis workflows.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.