How to Filter out NaN from a Data Selection of a Column of Strings using Python Pandas
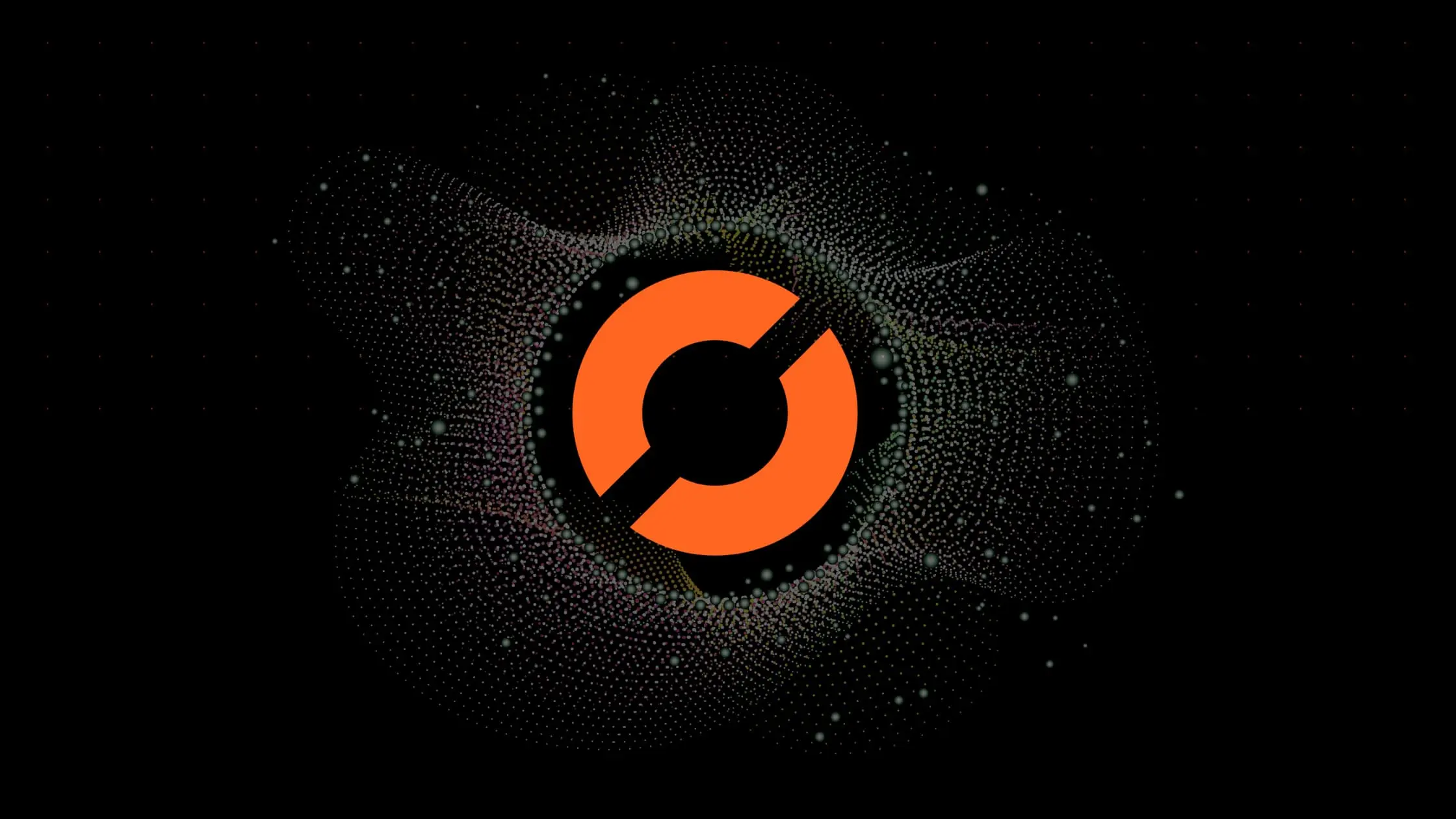
As a data scientist or software engineer, working with large datasets is a common task. Often, these datasets may contain missing or null values, which can hinder data analysis and modeling. Python Pandas is a powerful tool that can be used to clean and preprocess data, including filtering out NaN values from a data selection of a column of strings. In this article, we will explore how to do this effectively.
Table of Contents
- What is Pandas?
- Filtering out NaN from a Data Selection of a Column of Strings
- Common Errors and Solutions
- Conclusion
What is Pandas?
Pandas is an open-source library that is widely used for data analysis and manipulation. The library is built on top of two fundamental data structures: Series and DataFrame. Series is a one-dimensional array-like object that can hold any data type, while DataFrame is a two-dimensional table-like data structure that has rows and columns. With Pandas, you can perform a wide range of data manipulation tasks, such as cleaning, merging, grouping, and filtering data.
Filtering out NaN from a Data Selection of a Column of Strings
Method 1: Using dropna()
Function
The dropna()
function is a straightforward method to filter out NaN values from a column of strings. It operates directly on the specified column, providing a concise one-liner. However, it removes entire rows containing NaN, which may result in the loss of valuable information. This method is particularly useful when the primary goal is to clean the data by eliminating rows with missing values.
import pandas as pd
# Create a DataFrame
data = {'column_name': ['apple', 'banana', None, 'orange', 'grape']}
df = pd.DataFrame(data)
# Filter NaN using dropna()
filtered_data = df['column_name'].dropna()
print(filtered_data)
Output:
0 apple
1 banana
3 orange
4 grape
Name: column_name, dtype: object
Method 2: Using Boolean Indexing
Boolean indexing provides more flexibility in the filtering process. It involves creating a boolean mask based on the presence or absence of NaN values and applying this mask to the DataFrame. This method is advantageous when additional conditions need to be considered alongside NaN filtering. However, it may be less convenient for simple cases due to the need to manage a separate boolean mask.
import pandas as pd
# Create a DataFrame
data = {'column_name': ['apple', 'banana', None, 'orange', 'grape']}
df = pd.DataFrame(data)
# Create a boolean mask and apply it
mask = pd.notna(df['column_name'])
filtered_data = df[mask]
print(filtered_data)
Output:
column_name
0 apple
1 banana
3 orange
4 grape
Method 3: Combining isna()
and loc[]
This method involves combining the isna()
function, which explicitly identifies NaN values, with the loc[]
indexer for fine-grained control over row and column selection. While it requires using two separate methods, it offers precision in specifying the conditions for filtering. This approach is beneficial when there is a need for a more nuanced selection process.
import pandas as pd
# Create a DataFrame
data = {'column_name': ['apple', 'banana', None, 'orange', 'grape']}
df = pd.DataFrame(data)
# Use isna() and loc[] for filtering
filtered_data = df.loc[~df['column_name'].isna(), 'column_name']
print(filtered_data)
Output:
0 apple
1 banana
3 orange
4 grape
Name: column_name, dtype: object
Common Errors and Solutions
Before using these methods, be aware of common errors and their solutions:
Error: “AttributeError: ‘Series’ object has no attribute ‘dropna’":
- Solution: Ensure you are calling
dropna()
on a DataFrame, not a Series.
- Solution: Ensure you are calling
Error: “ValueError: cannot mask with array containing NA / NaN values”:
- Solution: Use the
pd.notna()
function to create a boolean mask without NaN values.
- Solution: Use the
Error: “IndexingError: Unalignable boolean Series provided as indexer”:
- Solution: Use
.loc[]
for both row and column selection.
- Solution: Use
Conclusion
Filtering out NaN values from a data selection of a column of strings in Pandas is a common task that can be accomplished using the notna()
method. This method returns a boolean mask that you can use to select the rows that do not contain NaN values. By removing missing values from your data, you can improve the accuracy of your data analysis and modeling.
In conclusion, Pandas is a powerful tool for data analysis and manipulation, and knowing how to filter out NaN values from a data selection of a column of strings is essential for any data scientist or software engineer.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.