How to Extract Value from a DataFrame: A Guide for Data Scientists
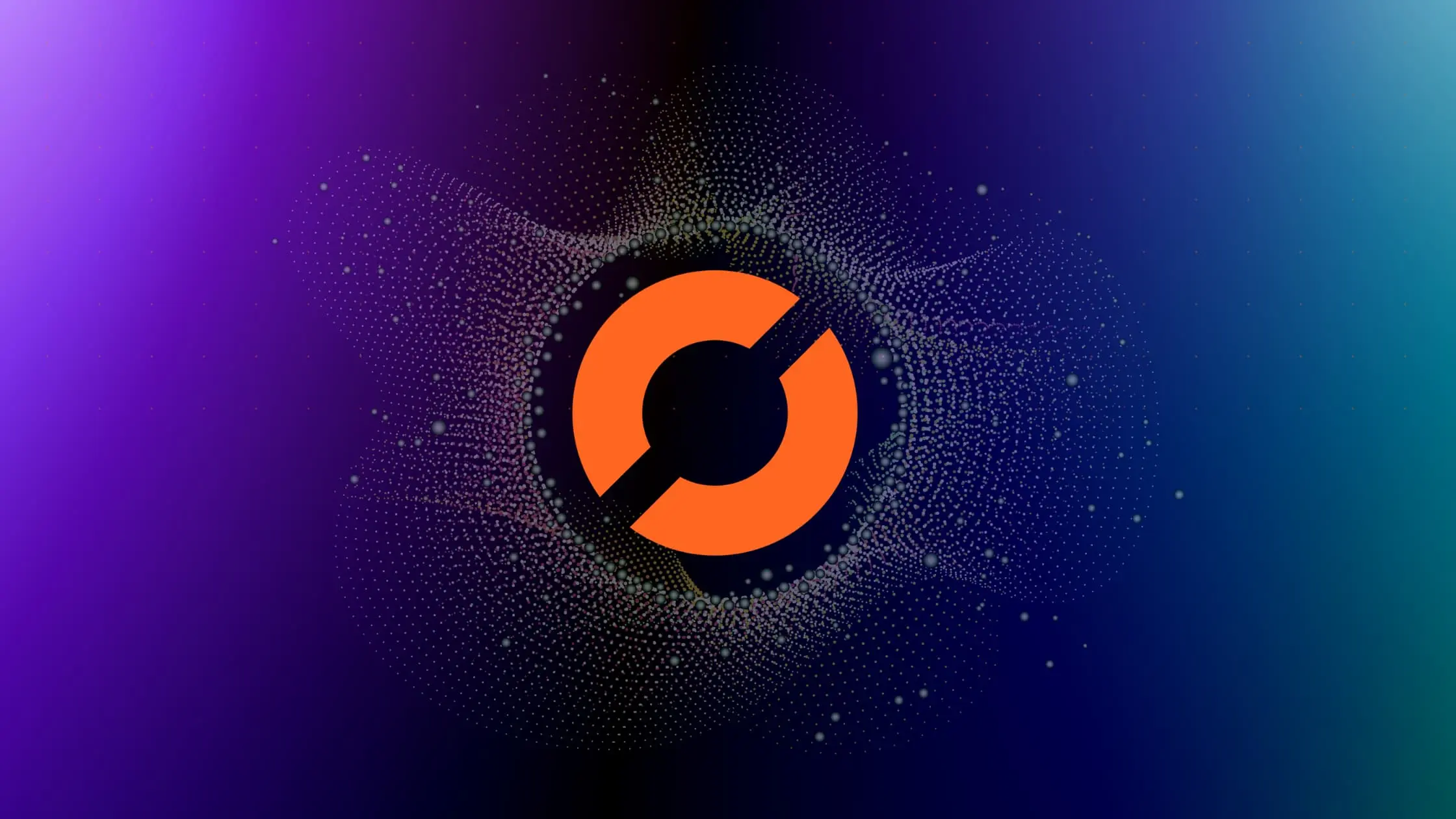
How to Extract Value from a DataFrame: A Guide for Data Scientists
DataFrames are a fundamental part of data manipulation in Python. They are two-dimensional data structures, essentially tables, that can store data of different types (including characters, integers, floating point values, factors, and more) in columns. But how do you extract value from a DataFrame? This guide will walk you through the process, step by step.
Understanding DataFrames
Before we dive into the specifics, it’s important to understand what a DataFrame is. In Python, a DataFrame is a two-dimensional labeled data structure with columns of potentially different types. It is similar to a spreadsheet or SQL table, or a dictionary of Series objects. DataFrames are generally the most commonly used pandas object.
Accessing DataFrame Values
There are several ways to access or extract data from a DataFrame. Here are the most common methods:
1. Using Column Name
You can extract a specific column from a DataFrame by using its name. For example:
import pandas as pd
# Create a simple dataframe
data = {'Name': ['John', 'Anna', 'Peter'],
'Age': [28, 24, 22]}
df = pd.DataFrame(data)
# Access the 'Name' column
print(df['Name'])
Output:
0 John
1 Anna
2 Peter
Name: Name, dtype: object
2. Using loc and iloc
loc
and iloc
are two access methods used to retrieve rows and columns. loc
is label-based, which means that you have to specify the name of the rows and columns that you need to filter out. On the other hand, iloc
is integer index-based. You have to specify rows and columns by their integer index.
# Using loc
print(df.loc[:, 'Name'])
# Using iloc
print(df.iloc[:, 0])
Output:
0 John
1 Anna
2 Peter
Name: Name, dtype: object
0 John
1 Anna
2 Peter
Name: Name, dtype: object
3. Using at and iat
at
and iat
are used to retrieve a single value at a particular row and column intersection. at
uses a label-based approach, while iat
uses an integer-based approach.
# Using at
print(df.at[0, 'Name'])
# Using iat
print(df.iat[0, 0])
Output:
John
John
Extracting Multiple Values
To extract multiple values from a DataFrame, you can use the following methods:
1. Using Multiple Column Names
print(df[['Name', 'Age']])
Output:
Name Age
0 John 28
1 Anna 24
2 Peter 22
2. Using loc and iloc for Multiple Columns
# Using loc
print(df.loc[:, ['Name', 'Age']])
# Using iloc
print(df.iloc[:, [0, 1]])
Output:
Name Age
0 John 28
1 Anna 24
2 Peter 22
Name Age
0 John 28
1 Anna 24
2 Peter 22
Conclusion
Extracting value from a DataFrame is a fundamental skill for any data scientist working with Python. Whether you’re using column names, loc, iloc, at, or iat, the ability to accurately and efficiently extract data from a DataFrame will significantly speed up your data analysis process.
Remember, the key to getting the most out of your DataFrame is understanding how it’s structured and how to access its components. With practice, you’ll be able to extract data from a DataFrame with ease.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.