How to Extract Hour from Timedelta in Pandas
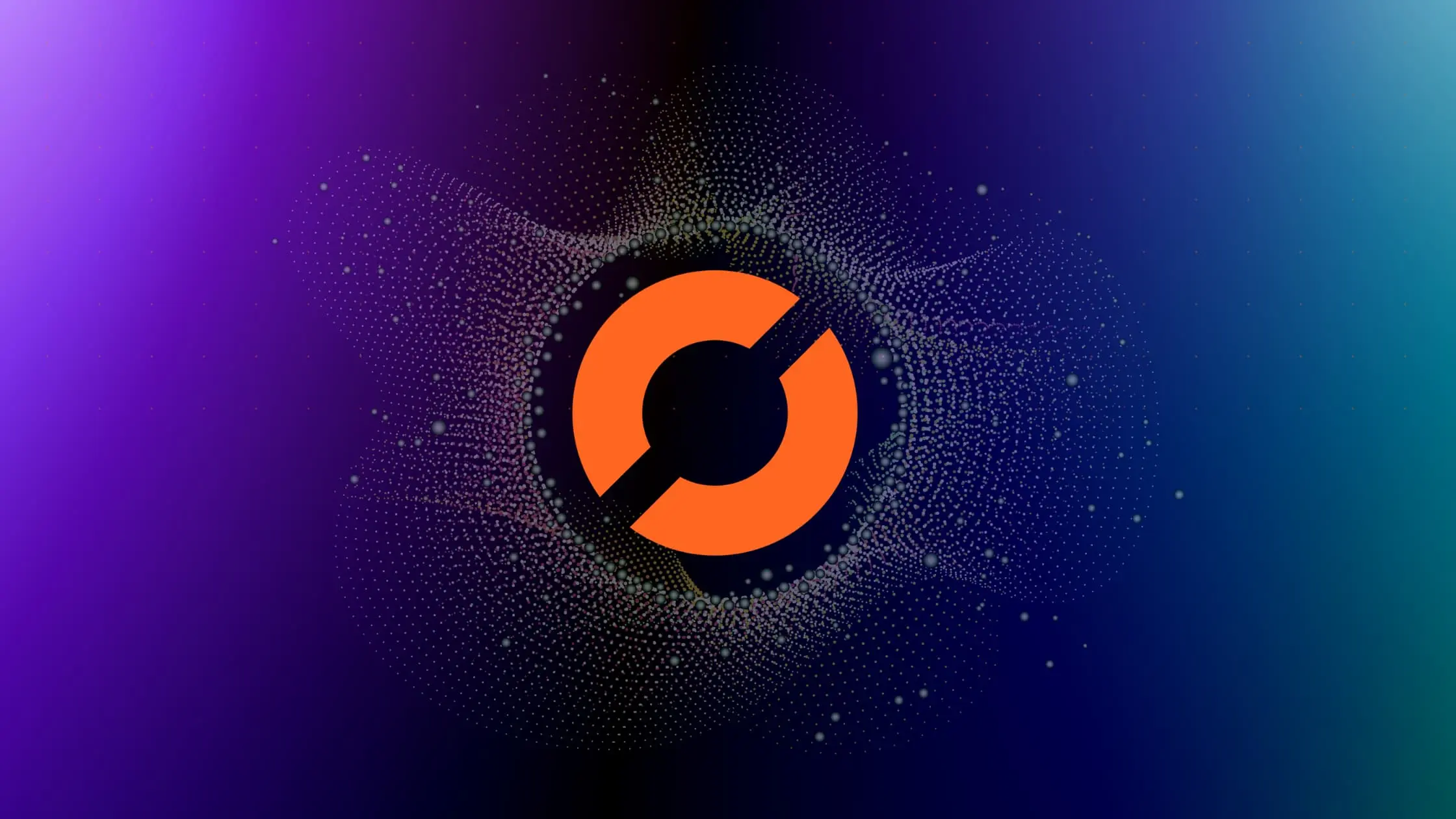
Pandas is a powerful data analysis library in Python that provides high-performance, easy-to-use data structures and data analysis tools. One of the common tasks in data analysis is to manipulate and extract information from date and time values. In this article, we will discuss how to extract hour from timedelta in Pandas.
Table of Contents
- What is Timedelta in Pandas?
- Extracting Hour from Timedelta in Pandas
- Extracting Hour from Timedelta in a Pandas DataFrame
- Common Errors and How to Handle Them
- Conclusion
What is Timedelta in Pandas?
Timedelta is a data type in Pandas that represents a duration or difference between two dates or times. It is similar to datetime, but instead of representing an absolute point in time, it represents a duration of time. Timedelta can be created by subtracting two datetime values or by specifying a duration in a string format.
import pandas as pd
# create a timedelta object
td = pd.Timedelta('1 day, 2 hours, 30 minutes, 15 seconds')
print(td)
# Output: 1 days 02:30:15
Extracting Hour from Timedelta in Pandas
To extract the hour component from a timedelta object in Pandas, we can use the hours
attribute.
import pandas as pd
# create a timedelta object
td = pd.Timedelta('1 day, 2 hours, 30 minutes, 15 seconds')
# extract the hour component
hour = td.hours
print(hour)
# Output: 2
In the above example, we created a timedelta object representing a duration of 1 day, 2 hours, 30 minutes, and 15 seconds. Then, we extracted the hour component using the hours
attribute of the timedelta object. The output shows that the hour component is 2.
Extracting Hour from Timedelta in a Pandas DataFrame
In data analysis, we often work with large datasets that contain date and time values in multiple columns. We may need to extract the hour component from a timedelta column in a Pandas DataFrame.
import pandas as pd
# create a sample DataFrame
df = pd.DataFrame({'start_time': ['2022-06-18 10:30:00', '2022-06-18 11:45:00', '2022-06-18 13:15:00'],
'end_time': ['2022-06-18 11:00:00', '2022-06-18 13:00:00', '2022-06-18 14:30:00']})
# convert string columns to datetime
df['start_time'] = pd.to_datetime(df['start_time'])
df['end_time'] = pd.to_datetime(df['end_time'])
# calculate duration and extract hour component
df['duration'] = df['end_time'] - df['start_time']
df['hour'] = df['duration'].dt.components.hours
print(df)
In the above example, we created a sample DataFrame with two columns of start and end times. We converted the string columns to datetime using pd.to_datetime()
function. Then, we calculated the duration of each event by subtracting the start time from the end time. Finally, we extracted the hour component from the duration column using the .dt
accessor and the hours
attribute.
The output of the above code will be:
start_time end_time duration hour
0 2022-06-18 10:30:00 2022-06-18 11:00:00 0 days 00:30:00 0
1 2022-06-18 11:45:00 2022-06-18 13:00:00 0 days 01:15:00 1
2 2022-06-18 13:15:00 2022-06-18 14:30:00 0 days 01:15:00 1
The output shows that we have successfully extracted the hour component from the duration column.
Pros and Cons
Pros:
- Simplicity: Using .components.hours is a concise and readable way to extract hours.
- Compatibility: This method is available in Pandas versions 1.1.0 and later.
Cons:
- Limited to Hours: This method only extracts hours. If you need other components, additional attributes must be used.
Common Errors and How to Handle Them
Error: 'TimedeltaProperties' object has no attribute 'hours'
This error occurs when trying to access .hours
on a Series or DataFrame directly. Ensure that you apply it to a Timedelta object.
# Incorrect usage
# hours = df['timedelta_column'].hours # This will raise an error
# Correct usage
hours = df['timedelta_column'].dt.components.hours
Note: This error occurs when attempting to use .components.hours on a version of Pandas earlier than 1.1.0. To handle this, make sure you have an up-to-date Pandas version:
pip install --upgrade pandas
Conclusion
In this article, we discussed how to extract the hour component from a timedelta object in Pandas. We have seen that we can use the hours
attribute of the timedelta object to extract the hour component. We have also seen how to extract the hour component from timedelta columns in a Pandas DataFrame using the .dt
accessor and the hours
attribute.
Extracting information from date and time values is a common task in data analysis, and Pandas provides a powerful set of tools to manipulate and extract information from date and time values. Understanding how to extract information from timedelta objects is an essential skill for any data scientist or software engineer working with time-series data.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.