How to Extract Date and Time from Timestamps using Pandas
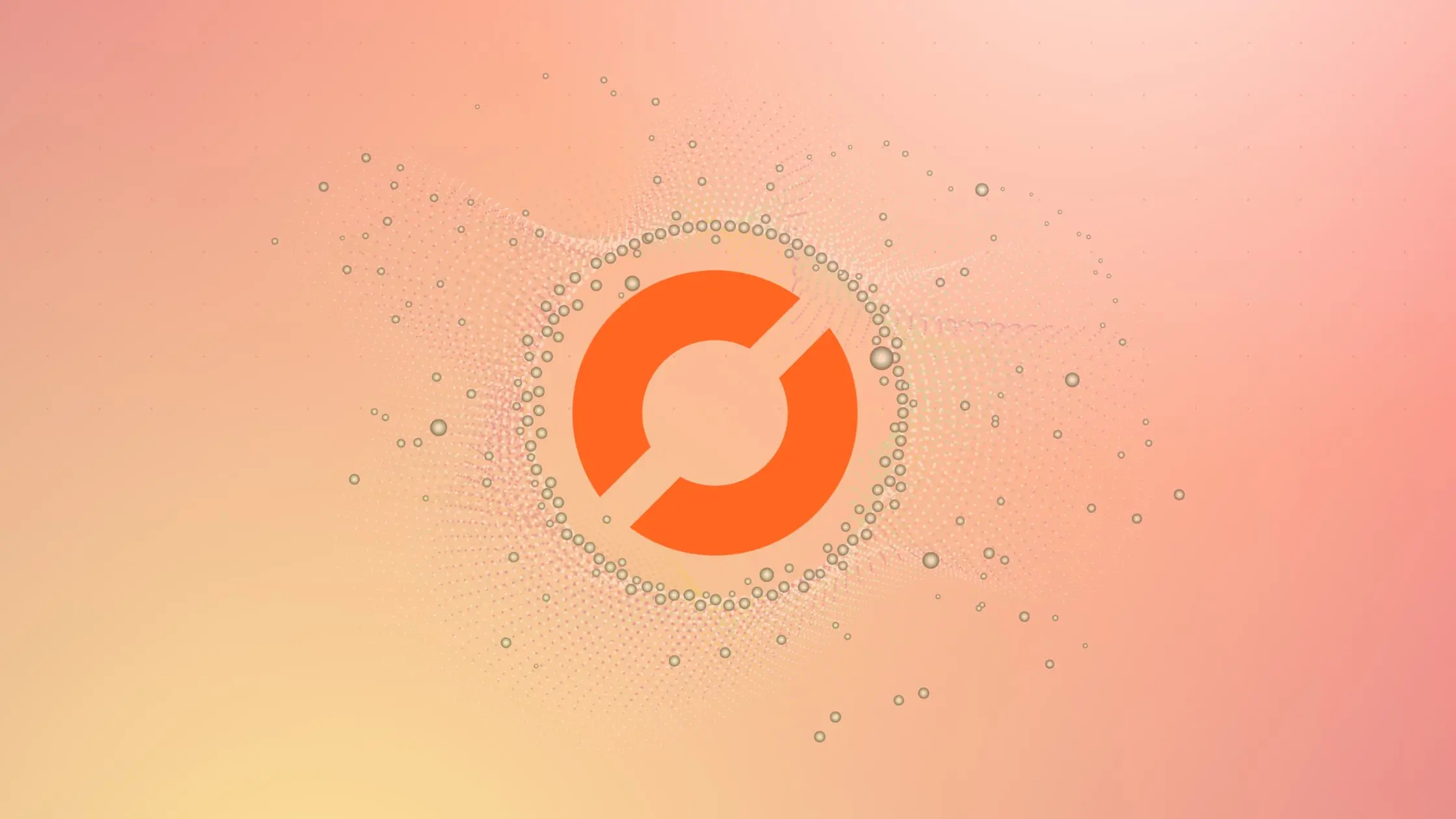
As a data scientist or software engineer working with data, you may encounter timestamps that contain valuable information, such as the date and time of an event. However, extracting this information from a timestamp can be challenging, especially when dealing with large datasets. In this article, we will show you how to extract the date and time from timestamps using the powerful Python library, Pandas.
Table of Contents
- What is Pandas?
- Why Extract Date and Time from Timestamps?
- How to Extract Date and Time from Timestamps using Pandas
- Common Errors and Solutions
- Best Practices
- Conclusion
What is Pandas?
Pandas is a Python library that provides powerful data manipulation and analysis capabilities. It is built on top of the NumPy library and provides data structures for efficiently handling large datasets. Pandas is widely used in data science, machine learning, and finance, among other fields.
Why Extract Date and Time from Timestamps?
Timestamps are commonly used to represent the time at which an event occurred. However, timestamps often contain more information than just the time. For example, a timestamp may contain the date, the time zone, or even microseconds. Extracting the date and time from a timestamp can be useful for various purposes, such as:
- Grouping data by day, week, or month
- Computing the time elapsed between two events
- Visualizing trends over time
How to Extract Date and Time from Timestamps using Pandas
Pandas provides a powerful set of tools for working with dates and times. In this section, we will show you how to extract the date and time from a timestamp using Pandas.
Let’s consider the following DataFrame:
timestamp event_data
0 2023-04-14 1.579213
1 2023-12-16 0.767435
2 2023-09-29 -0.469474
3 2023-04-18 0.542560
4 2023-03-14 -0.463418
5 2023-07-09 -0.465730
6 2023-01-22 0.241962
7 2023-04-14 -1.913280
8 2023-05-03 -1.724918
9 2023-08-04 -0.562288
Step 1: Import Pandas and Load Data
The first step is to import the Pandas library and load the data containing the timestamps. In this example, we will use a sample dataset containing timestamps representing the date and time of various events.
import pandas as pd
# Load data
df = pd.read_csv('events.csv')
Step 2: Convert Timestamps to Datetime Objects
The next step is to convert the timestamps to Pandas datetime objects. This is necessary to perform date and time operations on the data.
# Convert timestamps to datetime objects
df['timestamp'] = pd.to_datetime(df['timestamp'])
Step 3: Extract Date and Time Components
Now that the timestamps are in datetime format, we can easily extract the date and time components using the .dt
accessor. The .dt
accessor provides a set of datetime properties that can be used to extract various components of the datetime object.
# Extract date and time components
df['date'] = df['timestamp'].dt.date
df['time'] = df['timestamp'].dt.time
The above code creates two new columns in the dataframe df
, one for the date and one for the time. The dt.date
property extracts the date component of the datetime object, while the dt.time
property extracts the time component.
Step 4: Optional - Extract Other Components
In addition to the date and time components, Pandas provides other datetime properties that can be useful in some cases. For example, we can extract the day of the week, the month, or the year using the following code:
# Extract other datetime components
df['day_of_week'] = df['timestamp'].dt.day_name()
df['month'] = df['timestamp'].dt.month_name()
df['year'] = df['timestamp'].dt.year
The above code creates three new columns in the dataframe df
, one for the day of the week, one for the month, and one for the year. The dt.day_name()
property extracts the day of the week name, while the dt.month_name()
property extracts the month name.
Step 5: Export Data
Finally, we can export the data to a file or database for further analysis or visualization.
print(df)
# Export data
df.to_csv('events_processed.csv', index=False)
The above code exports the processed data to a CSV file named events_processed.csv
.
Output:
timestamp event_data date time day_of_week month year
0 2023-04-14 1.579213 2023-04-14 00:00:00 Friday April 2023
1 2023-12-16 0.767435 2023-12-16 00:00:00 Saturday December 2023
2 2023-09-29 -0.469474 2023-09-29 00:00:00 Friday September 2023
3 2023-04-18 0.542560 2023-04-18 00:00:00 Tuesday April 2023
4 2023-03-14 -0.463418 2023-03-14 00:00:00 Tuesday March 2023
5 2023-07-09 -0.465730 2023-07-09 00:00:00 Sunday July 2023
6 2023-01-22 0.241962 2023-01-22 00:00:00 Sunday January 2023
7 2023-04-14 -1.913280 2023-04-14 00:00:00 Friday April 2023
8 2023-05-03 -1.724918 2023-05-03 00:00:00 Wednesday May 2023
9 2023-08-04 -0.562288 2023-08-04 00:00:00 Friday August 2023
Common Errors and Solutions
1. Timestamps not Recognized:
- Error: When loading data, timestamps may not be recognized as datetime objects.
- Solution: Specify the date format while reading the CSV file using the
parse_dates
parameter.
# Load data with specified date format
df = pd.read_csv('events.csv', parse_dates=['timestamp'], date_parser=lambda x: pd.to_datetime(x, format='%Y-%m-%d'))
2. AttributeError: Can only use .dt accessor with datetimelike values
Error Description:
This error occurs when attempting to use the .dt
accessor on a column that is not recognized as containing datetime-like values. This typically happens when the column has not been converted to a datetime object using pd.to_datetime()
.
# Incorrect usage without converting to datetime
df['date'] = df['timestamp'].dt.date # This will raise AttributeError
Solution:
Ensure that you have converted the timestamp column to datetime format before applying the .dt
accessor.
# Convert timestamps to datetime objects
df['timestamp'] = pd.to_datetime(df['timestamp'])
# Now you can use .dt accessor
df['date'] = df['timestamp'].dt.date
3. TypeError: Cannot convert input to Timestamp
Error Description: This error occurs when there is an issue with the format of the timestamp data during conversion to datetime objects.
# Incorrect format causing TypeError
df['timestamp'] = pd.to_datetime(df['timestamp'], format='%d/%m/%Y') # This will raise TypeError
Solution:
Specify the correct format of the timestamp in the format
parameter of pd.to_datetime()
.
# Convert timestamps with correct format
df['timestamp'] = pd.to_datetime(df['timestamp'], format='%Y-%m-%d')
Best Practices
1. Always Convert to Datetime:
Ensure that your timestamp columns are converted to datetime objects using pd.to_datetime()
before performing any date and time operations.
# Convert timestamps to datetime objects
df['timestamp'] = pd.to_datetime(df['timestamp'])
2. Handle Missing Values:
Check for missing values in the timestamp column before performing operations to avoid unexpected errors.
# Check for missing values
if df['timestamp'].isnull().any():
# Handle missing values (e.g., fill or drop them)
3. Specify Timestamp Formats:
When converting timestamps to datetime objects, explicitly specify the format to avoid ambiguity and potential errors.
# Convert timestamps with format
df['timestamp'] = pd.to_datetime(df['timestamp'], format='%Y-%m-%d %H:%M:%S')
4. Handle Time Zone Appropriately:
Be mindful of time zones, especially when working with data from different sources. Use tz_localize
and tz_convert
methods if time zone adjustments are needed.
# Localize time zone (example: 'UTC')
df['timestamp'] = df['timestamp'].dt.tz_localize('UTC')
Conclusion
In this article, we have shown you how to extract the date and time from timestamps using Pandas. We started by importing the Pandas library and loading the data containing the timestamps. Then, we converted the timestamps to Pandas datetime objects and used the .dt
accessor to extract the date and time components. Finally, we exported the processed data to a file for further analysis or visualization.
By using Pandas to extract date and time information from timestamps, you can easily perform various date and time operations on large datasets. This can help you gain valuable insights into your data and make informed decisions.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.