How to Export from Pandas to Excel Without Row Names Index
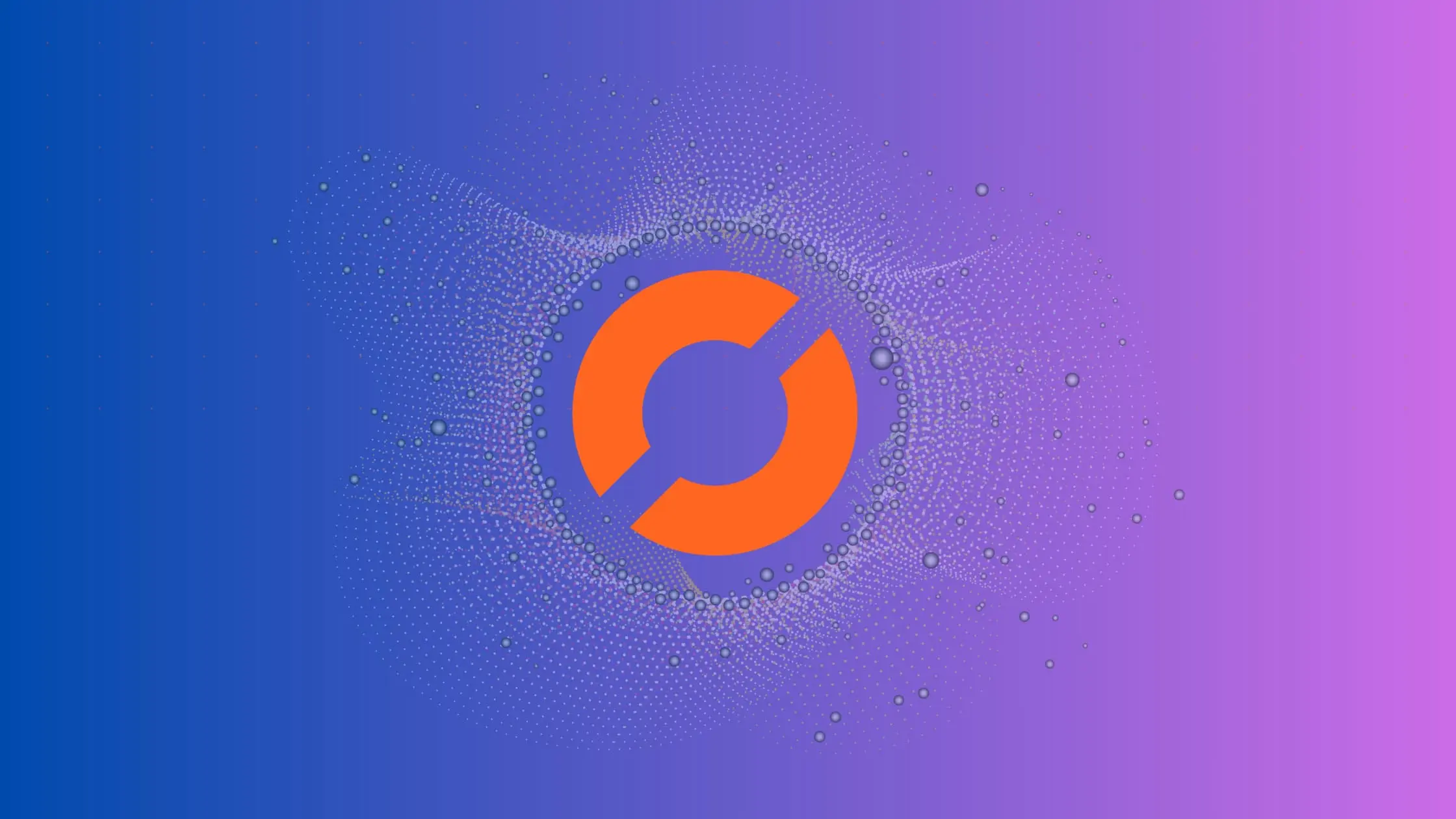
As a data scientist or software engineer working with data in Python, you may often need to export data from Pandas to Excel for further analysis or sharing with others. While Pandas provides an easy-to-use function for exporting data to Excel, one common issue that arises is the inclusion of row names, also known as the index, in the exported file. In this post, we will discuss how to export data from Pandas to Excel without row names.
The Problem
When exporting data from Pandas to Excel using the to_excel()
function, the default behavior is to include the row names in the exported file. This can be problematic, especially if the row names are not meaningful or if you want to use the exported file in other applications that do not recognize row names as a valid data field.
Consider the following example:
import pandas as pd
data = {'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35],
'Gender': ['F', 'M', 'M']}
df = pd.DataFrame(data)
df.to_excel('data.xlsx')
This code will export the df
DataFrame to an Excel file named data.xlsx
. However, if you open the exported file, you will notice that the row names, 0
, 1
, and 2
, are included in the first column of the worksheet.
The Solution
Method 1: Using index=False
Parameter
The simplest way to export data from Pandas to Excel without row names is to modify the to_excel()
function by setting the index
parameter to False
. By default, the index
parameter is set to True
, which includes the row names in the exported file.
df.to_excel('data.xlsx', index=False)
This will export the df
DataFrame to an Excel file named data.xlsx
without row names.
Method 2: Resetting Index Before Export
Another approach is to reset the index of the DataFrame before exporting. This involves using the reset_index()
method to remove the existing index and replace it with default integer index.
# Resetting index before export
df_reset = df.reset_index(drop=True)
df_reset.to_excel('output_reset.xlsx')
Common Errors in Exporting to Excel
Error 1: Duplicate Index Values
This error occurs when there are duplicate index values in the DataFrame. Excel requires unique row identifiers, and duplicate index values can lead to ambiguity.
Solution:
# Check for and handle duplicate index values
if df.duplicated().any():
df = df[~df.index.duplicated(keep='first')]
print("Duplicate index values handled.")
Error 2: Missing Dependencies
Missing dependencies, such as the openpyxl or xlrd libraries, can cause failures during export. Ensure these libraries are installed.
Solution: Ensure that the required libraries are installed:
pip install openpyxl xlrd
Error 3: Excel Truncating Data
Excel has limitations on the number of rows and columns it can handle. If your DataFrame exceeds these limits, Excel may truncate the data.
Solution: Subset the DataFrame to fit within Excel’s limitations:
# Subsetting DataFrame to fit within Excel limits
df_subset = df.iloc[:1048576, :] # Assuming Excel limit of rows is 1048576
df_subset.to_excel('output_subset.xlsx', index=False)
Conclusion
Exporting data from Pandas to Excel is a common task for data scientists and software engineers, but including row names in the exported file can be problematic in some cases. To export data from Pandas to Excel without row names, we need to set the index
parameter to False
in the to_excel()
function.
I hope this post has been helpful in solving this common issue. If you have any questions or comments, please feel free to leave them below.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.