How to Encrypt and Decrypt Payload Using AES/GCM/NoPadding Algorithm in Node.js and Java
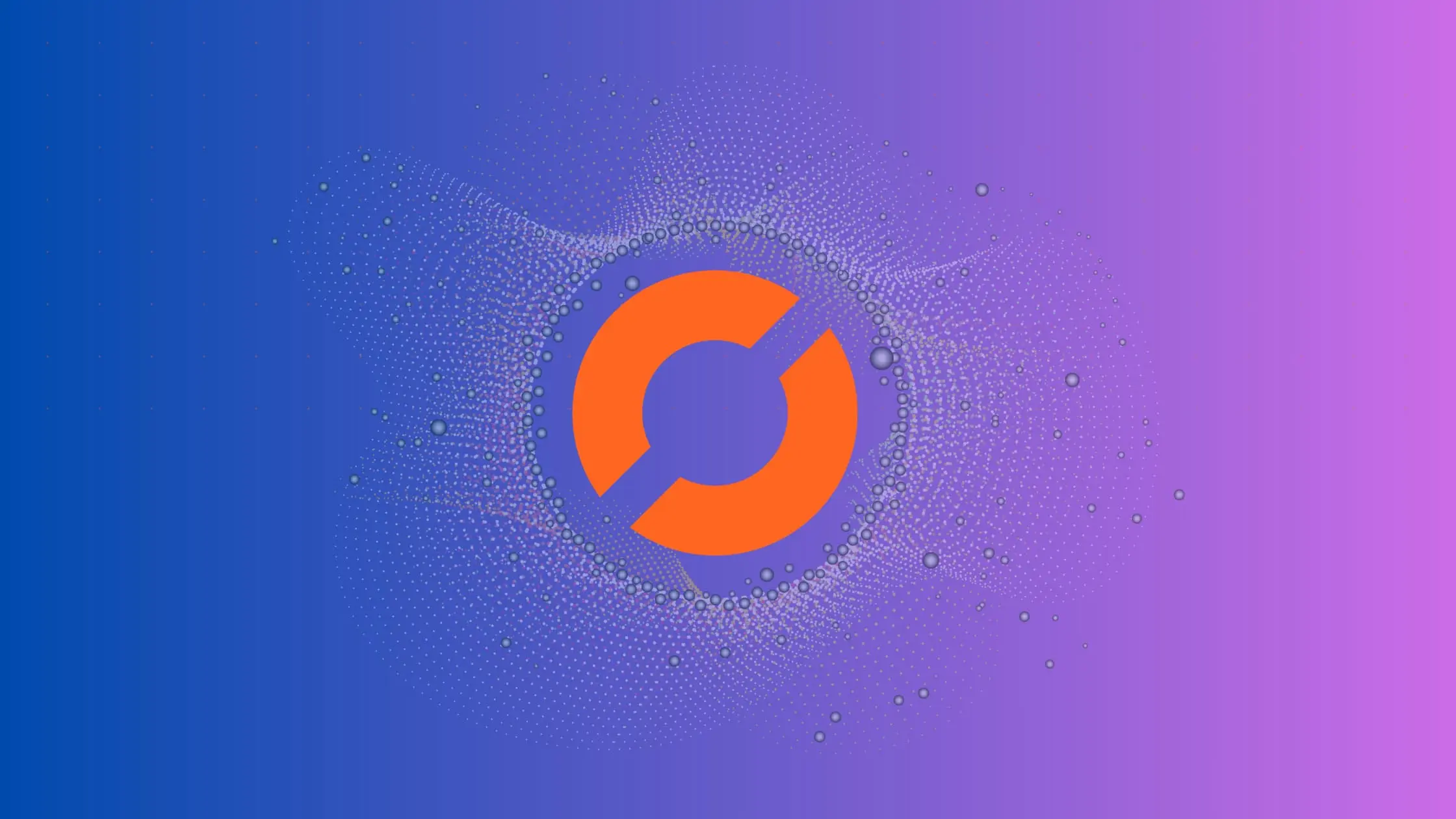
As a data scientist or software engineer working with sensitive data, ensuring the security and privacy of your payload is of utmost importance. One of the widely used encryption algorithms is AES (Advanced Encryption Standard), specifically using the GCM (Galois Counter Mode) mode of operation with NoPadding. In this article, we will explore how to encrypt a payload using a key and initialization vector (IV) in Node.js and decrypt it in Java, providing a secure data transfer mechanism.
Table of Contents
Prerequisites
Before we dive into the implementation details, make sure you have the following prerequisites set up:
- Node.js: Ensure you have Node.js installed on your machine.
- Java: Install the latest version of Java Development Kit (JDK) to compile and run Java code.
Encrypting the Payload in Node.js
To encrypt a payload in Node.js using the AES/GCM/NoPadding algorithm, follow the steps outlined below:
Step 1: Install Required Libraries
We will use the crypto
module in Node.js to perform encryption. Open your terminal and run the following command to install the necessary library:
npm install crypto
Step 2: Generate a Key and IV
To encrypt and decrypt the payload, we need a key and an IV. In Node.js, you can generate a cryptographically strong key and IV using the crypto.randomBytes
function. Here’s an example:
const crypto = require('crypto');
const key = crypto.randomBytes(32); // 256-bit key
const iv = crypto.randomBytes(12); // 96-bit IV
Step 3: Encrypt the Payload
Now that we have the key and IV, we can proceed with the encryption process. Here’s an example of how to encrypt a payload using the AES/GCM/NoPadding algorithm:
const plaintext = 'Your payload to encrypt';
const cipher = crypto.createCipheriv('aes-256-gcm', key, iv);
let encrypted = cipher.update(plaintext, 'utf8', 'hex');
encrypted += cipher.final('hex');
const tag = cipher.getAuthTag();
const output = Buffer.from(iv.toString('hex') + encrypted + tag.toString('hex'), 'hex').toString('base64');
In the above code, we create a cipher instance using the crypto.createCipheriv
method, passing the algorithm, key, and IV. We then encrypt the payload by calling cipher.update
and cipher.final
. Finally, we obtain the authentication tag using cipher.getAuthTag
, and concatenate the encrypted payload and the tag into a single buffe, which is then Base64-encoded.
Decrypting the Payload in Java
To decrypt the encrypted payload in Java, follow the steps outlined below:
Step 1: Set up Java Environment
Ensure that you have Java Development Kit (JDK) installed on your machine. You can check the installation by running the following command in your terminal:
javac -version
Step 2: Create a Java Project
Create a new Java project in your preferred development environment (e.g., IntelliJ, Eclipse) or use a text editor to write the code.
Step 3: Decrypt the Payload
To decrypt the payload, we will use the Java Cryptography Architecture (JCA) and the javax.crypto
package. Here’s an example code snippet to decrypt the payload:
import javax.crypto.Cipher;
import javax.crypto.spec.GCMParameterSpec;
import javax.crypto.spec.SecretKeySpec;
import java.nio.ByteBuffer;
import java.nio.charset.StandardCharsets;
import java.util.Base64;
public class PayloadDecryptor {
public static void main(String[] args) throws Exception {
byte[] encryptedData = // Encrypted data received from Node.js (Base64-encoded)
ByteBuffer buffer = ByteBuffer.wrap(encryptedData);
byte[] iv = new byte[12]; // 96-bit IV
buffer.get(iv);
byte[] payloadWithTag = new byte[buffer.remaining()];
buffer.get(payloadWithTag);
Cipher cipher = Cipher.getInstance("AES/GCM/NoPadding");
GCMParameterSpec gcmParameterSpec = new GCMParameterSpec(128, iv);
SecretKeySpec secretKeySpec = new SecretKeySpec(getKey(), "AES");
cipher.init(Cipher.DECRYPT_MODE, secretKeySpec, gcmParameterSpec);
byte[] decryptedPayload = cipher.doFinal(payloadWithTag);
String plaintext = new String(decryptedPayload, StandardCharsets.UTF_8);
System.out.println("Decrypted Payload: " + plaintext);
}
private static byte[] getKey() {
// Provide the key used for encryption (Base64-encoded)
return new byte[32]; // 256-bit key
}
}
In the above Java code, we extract the IV and encrypted payload from the received encrypted data. Then, we set up the decryption cipher using the Cipher
class with the AES/GCM/NoPadding algorithm. Finally, we decrypt the payload using cipher.doFinal
and convert it back to plaintext.
Conclusion
In this article, we explored how to encrypt a payload using the AES/GCM/NoPadding algorithm in Node.js and decrypt it in Java. By following the steps outlined, you can ensure secure data transfer between these two environments. Remember to use strong keys, IVs, and adhere to best practices for secure data handling.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.