How to do Gradient Clipping in PyTorch
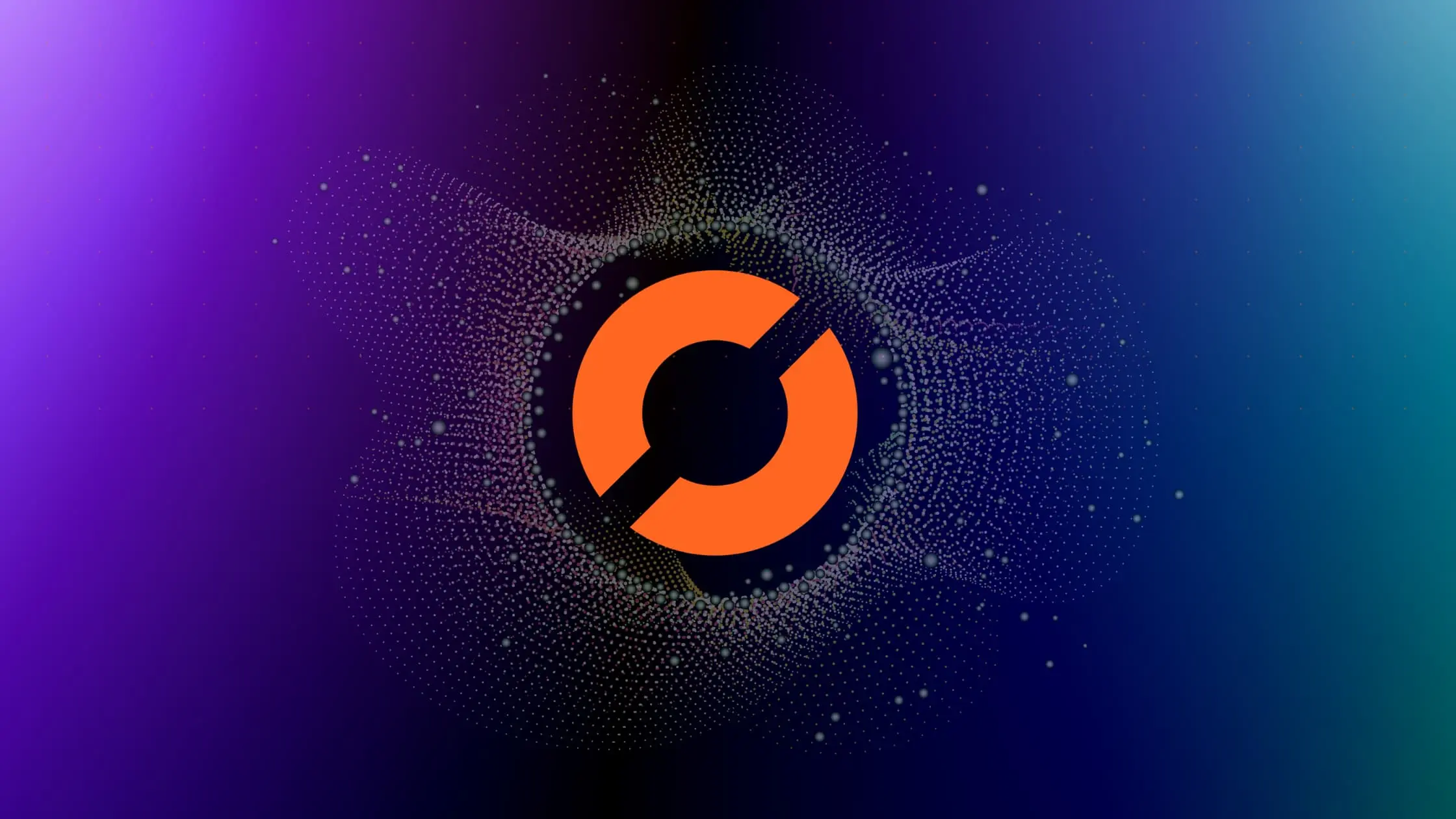
As a data scientist, you’ll likely work with machine learning models that require optimization techniques to ensure effective training. One such technique is gradient clipping, which is used to prevent exploding gradients during backpropagation. In this post, we’ll explore how to do gradient clipping in PyTorch, a popular deep learning framework.
What is Gradient Clipping?
Gradient clipping is a technique used to prevent exploding gradients, which can occur during backpropagation in deep neural networks. When the gradients are too large, the weights of the network can update too much, causing the model to diverge and fail to converge to a good solution.
To prevent this, we can clip the gradients to a maximum value. This ensures that the gradients are scaled down to a reasonable size, preventing the model from diverging. Gradient clipping is a form of regularization that can improve the generalization of the model.
How to do Gradient Clipping in PyTorch
PyTorch provides a simple way to clip gradients using the torch.nn.utils.clip_grad_norm_
function. This function takes in a list of parameters, a maximum gradient norm value, and a norm type, and clips the gradients of the parameters to the specified maximum norm value.
Here’s an example of how to use clip_grad_norm_
in PyTorch:
import torch.nn as nn
import torch.optim as optim
# Define a simple neural network
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.fc1 = nn.Linear(10, 5)
self.fc2 = nn.Linear(5, 1)
def forward(self, x):
x = nn.functional.relu(self.fc1(x))
x = self.fc2(x)
return x
# Create an instance of the neural network
net = Net()
# Define the optimizer and loss function
optimizer = optim.SGD(net.parameters(), lr=0.01)
criterion = nn.MSELoss()
# Train the model
for epoch in range(10):
for i, data in enumerate(trainloader, 0):
inputs, labels = data
# Zero the parameter gradients
optimizer.zero_grad()
# Forward + backward + optimize
outputs = net(inputs)
loss = criterion(outputs, labels)
loss.backward()
nn.utils.clip_grad_norm_(net.parameters(), 1.0)
optimizer.step()
In this example, we define a simple neural network with two fully connected layers and use the SGD
optimizer with a learning rate of 0.01. We also define the mean squared error loss function.
During training, we use the clip_grad_norm_
function to clip the gradients of the neural network parameters to a maximum norm of 1.0. This ensures that the gradients are scaled down to a reasonable size, preventing exploding gradients.
Choosing the Maximum Gradient Norm Value
The maximum gradient norm value that you use for gradient clipping depends on the specific model and dataset that you’re working with. In general, you should choose a value that is large enough to allow the model to learn quickly, but small enough to prevent exploding gradients.
A good starting point for the maximum gradient norm value is 1.0, as shown in the example above. You can experiment with different values to see what works best for your model and dataset.
Conclusion
Gradient clipping is an important technique for preventing exploding gradients during backpropagation in deep neural networks. In PyTorch, you can easily clip gradients using the torch.nn.utils.clip_grad_norm_
function.
When using gradient clipping, it’s important to choose an appropriate maximum gradient norm value that balances fast learning with preventing exploding gradients. By using gradient clipping, you can improve the stability and generalization of your machine learning models.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.