How to Delete Column Names in Pandas
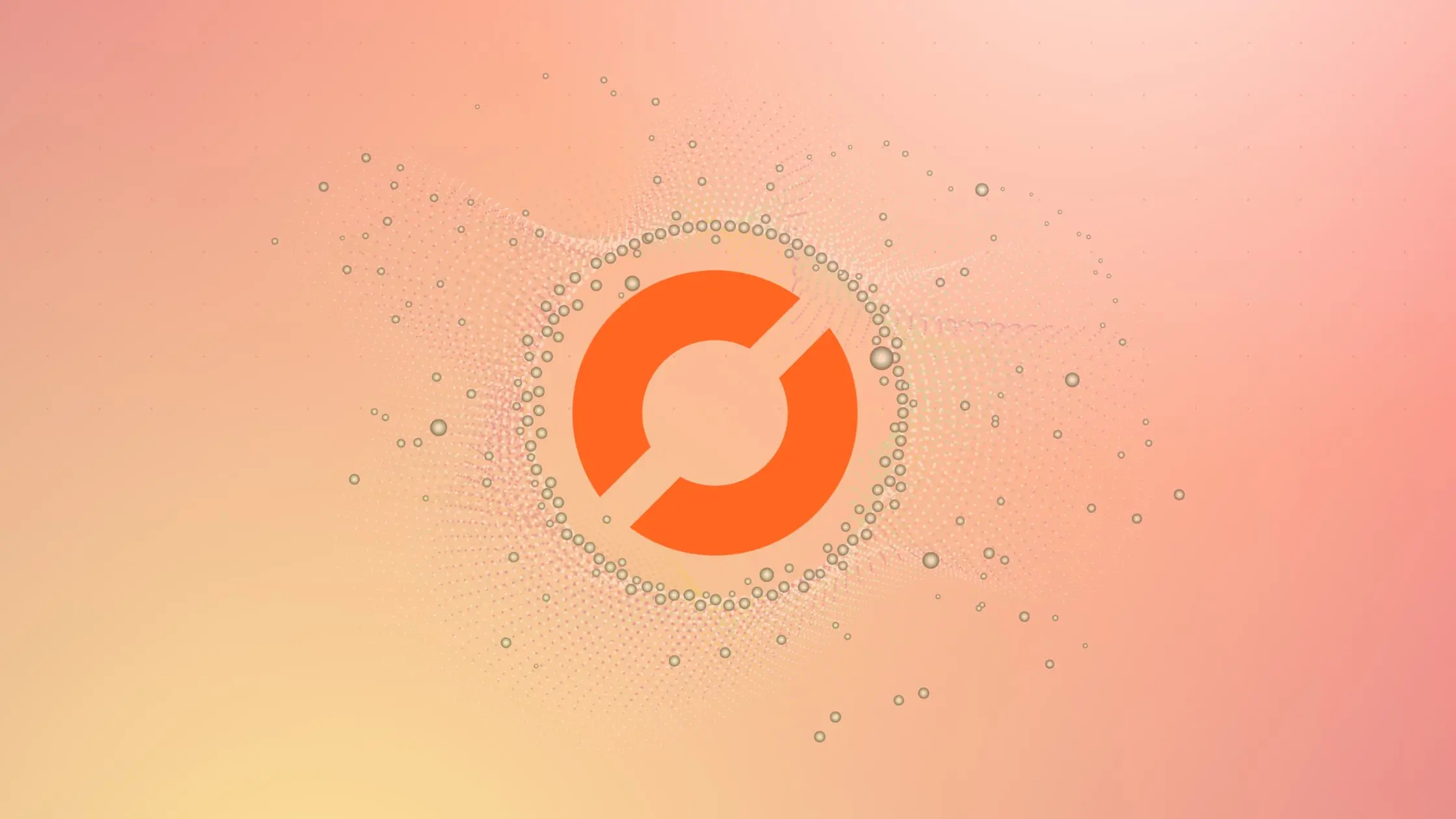
Pandas is a popular data manipulation library in Python that provides high-performance and easy-to-use data structures for data analysis. One of the frequently used operations in Pandas is to delete a column name. In this article, we will discuss various methods to delete a column name in Pandas.
Why Delete a Column Name?
There are several reasons why you may need to delete a column name in a Pandas DataFrame:
- The column name may be misspelled or have incorrect information
- The column may contain irrelevant or redundant information
- The column may contain sensitive or confidential information that needs to be removed
- The column may be causing issues when performing data analysis or visualization
Method 1: Using the drop() Method
The drop()
method in Pandas allows us to remove a column or row from a DataFrame. To delete a column name, we can use the axis
parameter with a value of 1, which indicates the column axis.
import pandas as pd
# create a sample DataFrame
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6], 'C': [7, 8, 9]})
# display the DataFrame
print(df)
# delete column 'B'
df = df.drop('B', axis=1)
# display the updated DataFrame
print(df)
Output:
A B C
0 1 4 7
1 2 5 8
2 3 6 9
A C
0 1 7
1 2 8
2 3 9
In this example, we created a sample DataFrame with three columns (‘A’, ‘B’, and ‘C’) and then deleted the column ‘B’ using the drop()
method with axis=1
. This resulted in a new DataFrame with only two columns (‘A’ and ‘C’).
Pros
Flexibility: The
drop()
method is versatile and can be used to remove both rows and columns. It allows users to specify the axis of operation (axis=1 for columns in this case).Returns a New DataFrame: The
drop()
method returns a new DataFrame with the specified column removed, leaving the original DataFrame unchanged. This can be beneficial for creating new views or avoiding unintended modifications.
Cons
Immutability: While returning a new DataFrame can be an advantage, it may also be a drawback if users specifically want to modify the original DataFrame in place.
Requires Reassignment: The method requires reassigning the result back to the original DataFrame (
df = df.drop('B', axis=1)
), which might be considered less concise compared to in-place operations.
Method 2: Using the del Statement
Another way to delete a column name in Pandas is to use the del
statement. This method directly modifies the original DataFrame and does not return a new DataFrame.
import pandas as pd
# create a sample DataFrame
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6], 'C': [7, 8, 9]})
# display the DataFrame
print(df)
# delete column 'B'
del df['B']
# display the updated DataFrame
print(df)
Output:
A B C
0 1 4 7
1 2 5 8
2 3 6 9
A C
0 1 7
1 2 8
2 3 9
In this example, we used the del
statement to delete the column ‘B’ directly from the original DataFrame.
Pros
In-Place Modification: The
del
statement directly modifies the original DataFrame in place, which can be advantageous if the goal is to perform the deletion without creating a new DataFrame.Conciseness: The
del
statement is concise and directly conveys the intent of deleting the specified column.
Cons
No Returned DataFrame: Unlike the
drop()
method, thedel
statement does not return a new DataFrame. This means there is no reference to the removed column after deletion.Potential for Unintended Changes: In-place modifications may lead to unexpected changes if not used carefully. It might be less suitable for scenarios where traceability is crucial.
Method 3: Using the pop() Method
The pop()
method in Pandas removes and returns a column from a DataFrame. This method modifies the original DataFrame and does not return a new DataFrame.
import pandas as pd
# create a sample DataFrame
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6], 'C': [7, 8, 9]})
# display the DataFrame
print(df)
# delete column 'B'
df.pop('B')
# display the updated DataFrame
print(df)
Output:
A B C
0 1 4 7
1 2 5 8
2 3 6 9
A C
0 1 7
1 2 8
2 3 9
In this example, we used the pop()
method to remove and return the column ‘B’ from the DataFrame.
Pros
Returns Removed Column: The
pop()
method not only removes the specified column in place but also returns the removed column as a Series. This provides a way to retain and store the removed column separately if needed.In-Place Modification: Similar to the
del
statement, thepop()
method modifies the original DataFrame directly.
Cons
No Returned DataFrame: Like the
del
statement, thepop()
method does not return a new DataFrame. The primary result is the modified original DataFrame.Limited to Columns: The
pop()
method is specifically designed for column removal and cannot be used for row removal.
Error Handling for Deleting a Column in Pandas:
When deleting a column in Pandas using methods like drop(), del, or pop(), error handling can be implemented to handle potential issues that might arise during the deletion process. Here are some common scenarios and ways to handle errors:
- Column Not Found:
Check if the column exists before attempting to delete it.
Use a try-except block to catch the KeyError
that might occur if the specified column is not found.
column_name = 'B'
try:
df = df.drop(column_name, axis=1)
except KeyError:
print(f"Column '{column_name}' not found.")
- Deletion of Multiple Columns:
Ensure that all specified columns for deletion exist before attempting deletion.
Use a loop to iterate through multiple columns and catch KeyError
for each column.
columns_to_delete = ['B', 'D']
for col in columns_to_delete:
try:
df = df.drop(col, axis=1)
except KeyError:
print(f"Column '{col}' not found.")
- Deletion of Nonexistent Column Using
del
orpop()
:
Similar to the drop()
method, check if the column exists before attempting deletion using del
or pop()
.
Use an if statement to verify the existence of the column before deletion.
column_name = 'B'
if column_name in df.columns:
del df[column_name]
else:
print(f"Column '{column_name}' not found.")
- Handling Unexpected Errors:
Implement a generic except block to catch unexpected errors during deletion. Log or print an informative message to identify and debug the issue.
column_name = 'B'
try:
df = df.drop(column_name, axis=1)
except Exception as e:
print(f"An unexpected error occurred: {str(e)}")
# Log the error for further investigation
Conclusion
In this article, we discussed various methods to delete a column name in Pandas. The drop()
, del
, and pop()
methods are all effective ways to remove a column from a DataFrame. Depending on the use case, you can choose the method that suits you best. These methods are also useful for removing rows, which can be done by setting the axis
parameter to 0.
When working with data, it’s important to be able to manipulate it quickly and efficiently, and Pandas provides a powerful set of tools for achieving this. By mastering these methods, you’ll be able to clean and transform your data with ease, making it ready for analysis and visualization.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.