How to create new values in a pandas dataframe column based on values from another column
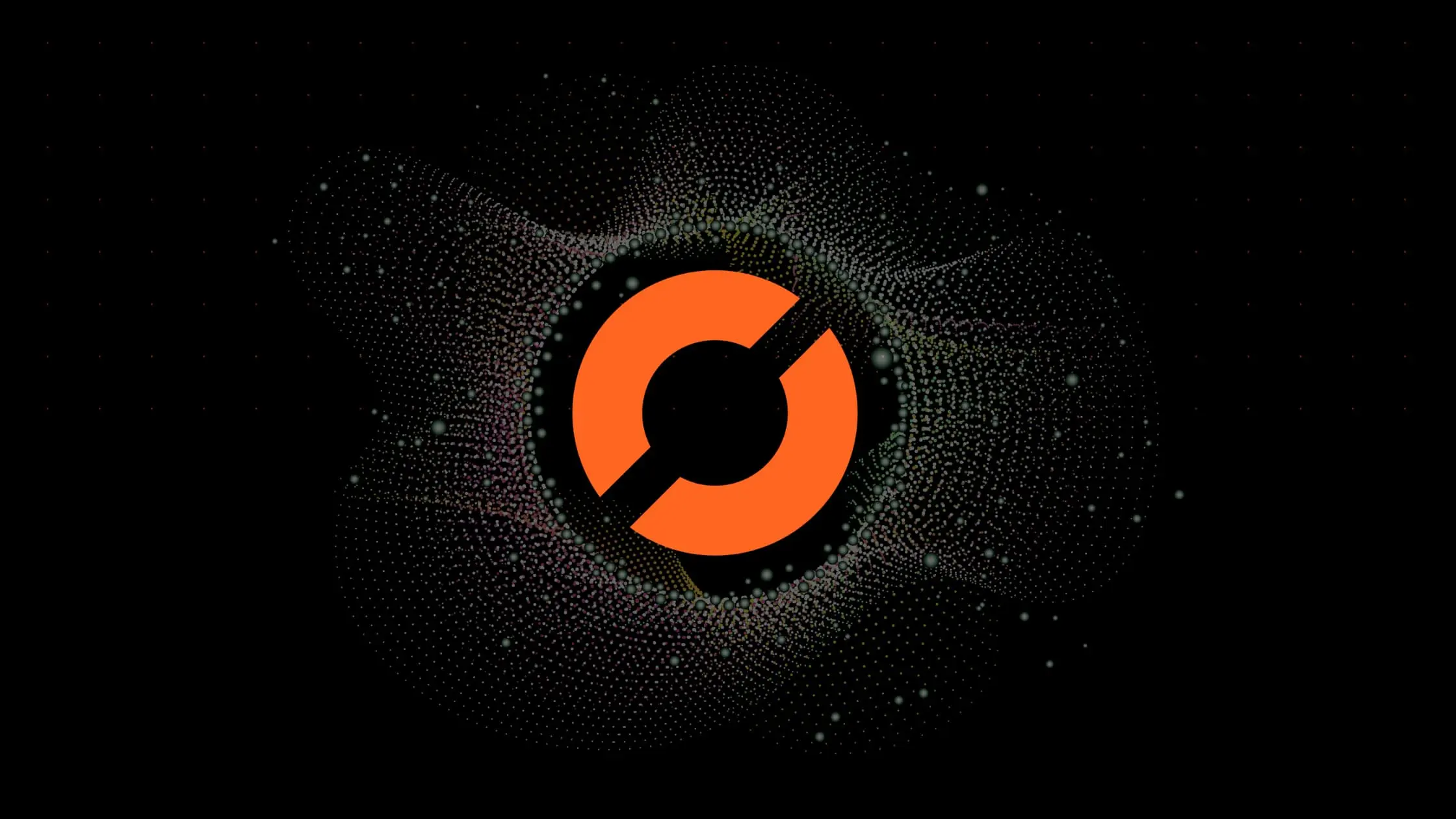
As a data scientist or software engineer, you often encounter situations where you need to manipulate data in a pandas dataframe. One common task is to create new values in a dataframe column based on values from another column. In this article, we will explore how to achieve this using pandas.
Table of Contents
Understanding the problem
Before we dive into the solution, let us first understand the problem we are trying to solve. Suppose we have a pandas dataframe with the following columns:
| Name | Age |
|------|-----|
| John | 25 |
| Mary | 30 |
| Jane | 35 |
Now, suppose we want to create a new column called “Category” based on the Age column. We want to categorize people into three groups: “Young” if their age is less than 30, “Middle-aged” if their age is between 30 and 40, and “Elderly” if their age is greater than 40. The resulting dataframe should look like this:
| Name | Age | Category |
|------|-----|------------|
| John | 25 | Young |
| Mary | 30 | Middle-aged|
| Jane | 35 | Middle-aged|
Solution
Using apply()
function
To create the new column based on values from another column, we can use the apply()
function in pandas. The apply()
function applies a function to each element in a pandas series or dataframe. We can define a function that takes an age value and returns the corresponding category.
Here is the code to create the new column:
import pandas as pd
# Create the original dataframe
df = pd.DataFrame({'Name': ['John', 'Mary', 'Jane'],
'Age': [25, 30, 35]})
# Define the function to categorize ages
def categorize_age(age):
if age < 30:
return 'Young'
elif age >= 30 and age <= 40:
return 'Middle-aged'
else:
return 'Elderly'
# Apply the function to the Age column using the apply() function
df['Category'] = df['Age'].apply(categorize_age)
# Print the resulting dataframe
print(df)
The output of the above code should be:
| Name | Age | Category |
|------|-----|------------|
| John | 25 | Young |
| Mary | 30 | Middle-aged|
| Jane | 35 | Middle-aged|
Using numpy
and np.select()
While the apply()
function is a powerful tool for creating new columns based on existing ones, an alternative method using numpy
and np.select()
can provide a more concise and efficient solution, especially when dealing with multiple conditions.
Here’s how you can achieve the same result using numpy
:
import pandas as pd
import numpy as np
# Create the original dataframe
df = pd.DataFrame({'Name': ['John', 'Mary', 'Jane'],
'Age': [25, 30, 35]})
# Define the conditions and corresponding categories
conditions = [
df['Age'] < 30,
(df['Age'] >= 30) & (df['Age'] <= 40),
df['Age'] > 40
]
categories = ['Young', 'Middle-aged', 'Elderly']
# Use np.select() to create the new column
df['Category'] = np.select(conditions, categories, default='Unknown')
# Print the resulting dataframe
print(df)
This approach can be particularly useful when dealing with more complex conditions, as it allows you to express them in a more concise manner.
Output:
Name Age Category
0 John 25 Young
1 Mary 30 Middle-aged
2 Jane 35 Middle-aged
Common Errors and Solutions:
1. Error: Misusing Conditions in apply()
or np.select()
Common Mistake: Incorrectly defining conditions can lead to unexpected results. For example, using
age >= 30 and age <= 40
instead of the correctage >= 30 & age <= 40
in theapply()
function ornumpy
conditions.Solution: Ensure that you use the correct syntax for conditions. In pandas, use
&
for element-wise logical AND operations, and make sure to wrap conditions in parentheses for proper evaluation.
2. Error: Forgetting Default Value in np.select()
Common Mistake: Forgetting to provide a default value in
np.select()
can result in unexpected behavior, especially when none of the specified conditions is satisfied.Solution: Always include a default value in
np.select()
to handle cases where none of the conditions is true. This can prevent the creation of a column with unexpected null values.
Best Practices:
1. Vectorized Operations for Efficiency:
- Leverage vectorized operations provided by pandas and numpy for improved performance. These operations are optimized for handling large datasets efficiently.
2. Use loc
for DataFrame Modifications:
- When modifying a DataFrame based on conditions, consider using
df.loc[conditions, 'Column']
for assignment. This ensures that modifications occur in place, avoiding potential SettingWithCopyWarning issues.
3. Test with Sample Data:
- Before applying transformations to the entire dataset, test your code with a smaller sample to catch potential errors and ensure the desired outcome.
4. Document Your Code:
- Clearly document the conditions and logic used for creating new columns. This helps in maintaining and debugging code in the future.
Conclusion
Conclusion:
In this exploration of creating new values in a pandas dataframe based on values from another column, we’ve covered two effective methods: the classic apply()
function and a more concise approach using numpy
and np.select()
. Both methods offer flexibility, allowing data scientists and software engineers to choose the one that suits their preferences and specific requirements.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.