How to Create Multiple Columns in Pandas Dataframe from One Function
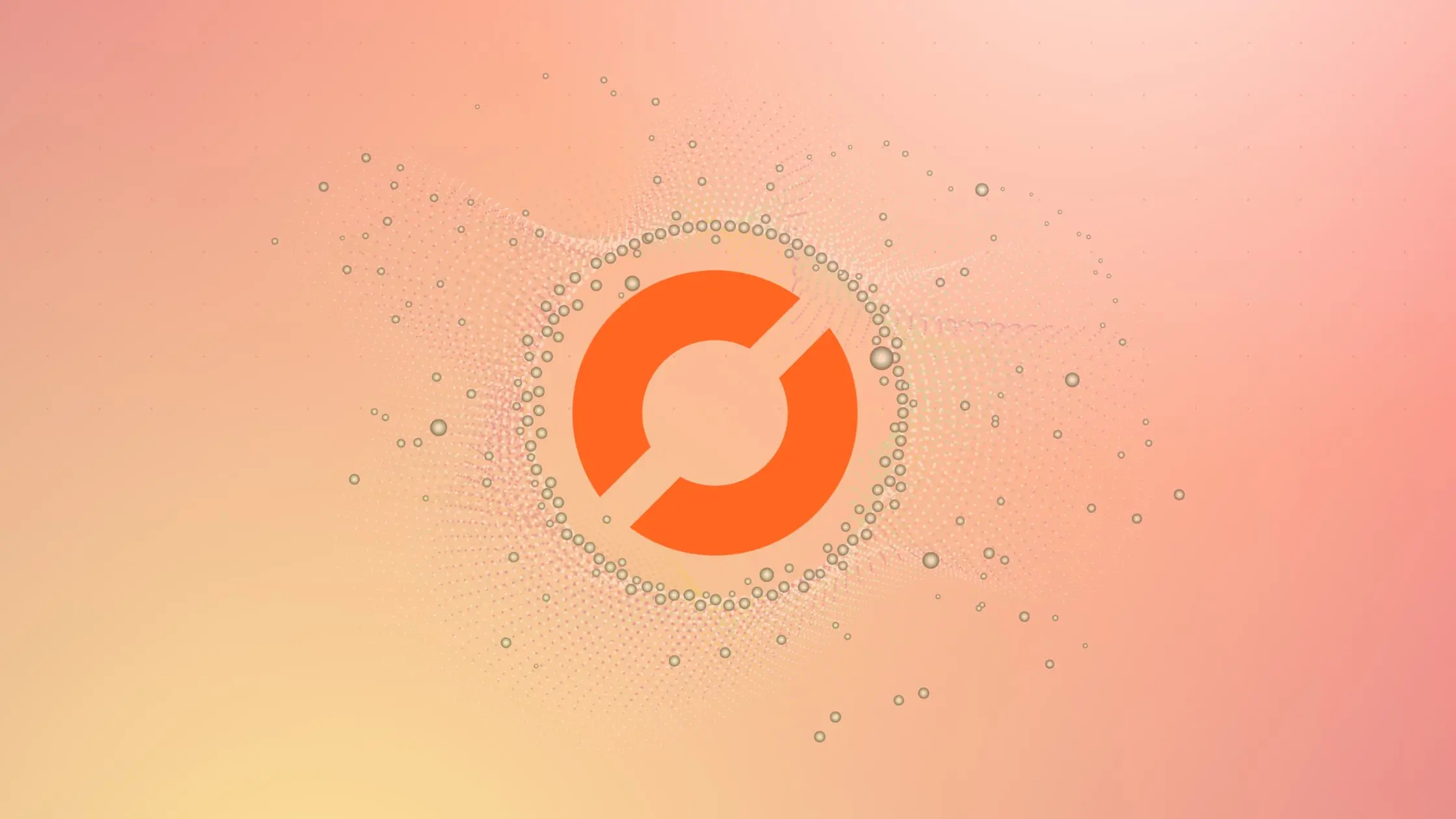
As a data scientist or software engineer, you might often come across situations where you need to create multiple columns in a Pandas dataframe from a single function. This can be a tedious and time-consuming task if done manually. In this blog post, we will explore how to create multiple columns in Pandas dataframe from one function, and automate this process, saving you valuable time and effort.
Table of Contents
What is Pandas Dataframe?
Pandas is an open-source data analysis and manipulation library for Python. It provides two primary data structures - Series and Dataframe. A Pandas Dataframe is a two-dimensional labeled data structure with columns of potentially different types, similar to a spreadsheet or SQL table. It is the most commonly used Pandas object and is designed to handle tabular data.
The Problem
Let’s say you have a Pandas dataframe with a column containing a string of text. You want to create multiple columns from this text, such as extracting the first word, the last word, and the length of the text. Doing this manually would involve creating new columns and applying functions to each row of data. This can be time-consuming, especially if you have a large dataset.
The Solution
Apply same function for every columns
To streamline the process, let’s create a function that generates multiple columns based on specified logic. Here’s a simple example:
import pandas as pd
def create_multiple_columns(dataframe, column_names, logic_function):
for column_name in column_names:
dataframe[column_name] = logic_function(dataframe)
return dataframe
data = {'A': [1, 2, 3], 'B': [4, 5, 6]}
df = pd.DataFrame(data)
column_names = ['C', 'D']
logic_function = lambda df: [val * 2 for val in df['A']]
result_df = create_multiple_columns(df, column_names, logic_function)
print(result_df)
Output:
A B C D
0 1 4 2 2
1 2 5 4 4
2 3 6 6 6
In this code, we used Pandas to create a DataFrame with columns 'A'
and 'B'
, then applies a custom logic function to double the values in column 'A'
. It adds the result as new columns 'C'
and 'D'
to the DataFrame and prints the updated DataFrame.
Applying each function for each column
The solution to this problem is to use the apply()
function in Pandas. This function applies a function along an axis of the dataframe. We can use this function to apply a custom function to each row of data, and create multiple columns from the result.
Here’s an example of how to create multiple columns from a single column of text:
import pandas as pd
# create a sample dataframe
data = {'text': ['hello world', 'how are you', 'goodbye']}
df = pd.DataFrame(data)
# define a function to extract the first word
def first_word(text):
return text.split()[0]
# define a function to extract the last word
def last_word(text):
return text.split()[-1]
# define a function to calculate the length of the text
def text_length(text):
return len(text)
# apply the functions to the dataframe
df['first_word'] = df['text'].apply(first_word)
df['last_word'] = df['text'].apply(last_word)
df['text_length'] = df['text'].apply(text_length)
# display the dataframe
print(df)
Output:
text first_word last_word text_length
0 hello world hello world 11
1 how are you how you 11
2 goodbye goodbye goodbye 7
In this example, we created a sample dataframe with a column containing text. We then defined three functions - first_word()
, last_word()
, and text_length()
- to extract the first word, last word, and length of the text, respectively. We then applied these functions to the dataframe using the apply()
function and created three new columns - first_word
, last_word
, and text_length
- with the results.
Common Errors and How to Handle Them
Error 1:
"ValueError: Length of values does not match length of index"
This error occurs when the lengths of the generated columns do not match. Validate the lengths before assigning to the DataFrame.Error 2:
"KeyError: 'Column_Name'"
Check if the specified column name exists in the DataFrame before attempting to assign values to it.Error 3:
"TypeError: 'NoneType' object is not iterable"
Ensure that the function you pass to create_multiple_columns returns a iterable object (e.g., a list, array).
Conclusion
In conclusion, if it comes to applying different function for different column, the apply()
function in Pandas is a powerful tool that can be used to create multiple columns from a single function, saving you valuable time and effort. On the other hand, for applying the same function to every column, we can define it by ourselves as showned above. By applying a custom function to each row of data, you can create new columns with the results, without having to do it manually. This technique can be used to process large datasets and is an essential skill for any data scientist or software engineer working with Pandas dataframes.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.