How to Create an Index for Python Pandas DataFrame: A Guide
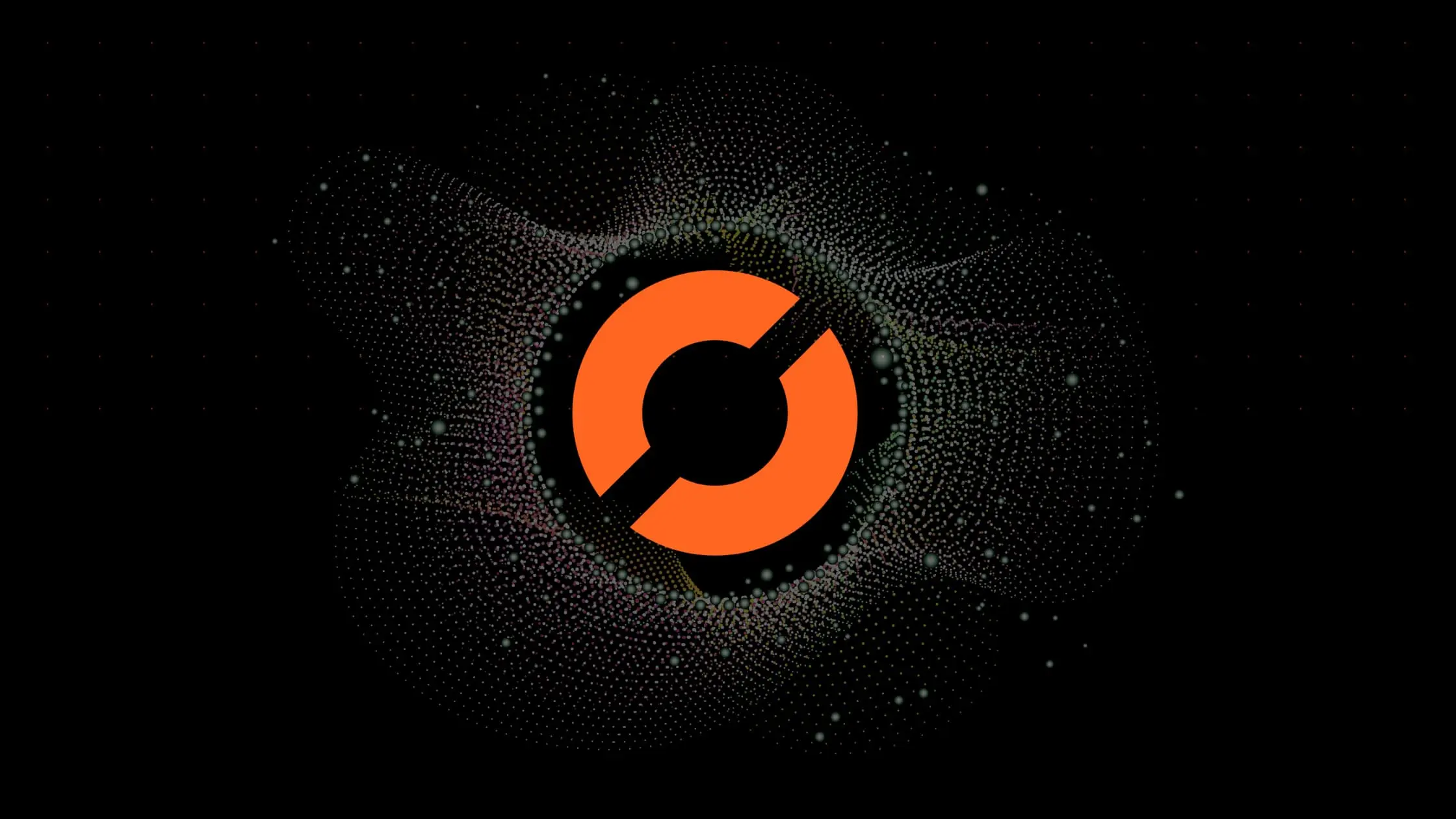
How to Create an Index for Python Pandas DataFrame: A Guide
Python’s Pandas library is a powerful tool for data manipulation and analysis. One of its most important features is the DataFrame, a two-dimensional data structure similar to a table in a relational database. In this blog post, we’ll explore how to create an index for a Pandas DataFrame, a crucial step in optimizing your data for efficient querying and analysis.
What is an Index in Pandas?
Before we dive into the how, let’s understand the what. An index in Pandas is like an address, it’s how any data point across the DataFrame or series can be accessed. Indexes can be numeric, string, or even datetime. They can also be unique or non-unique. By default, Pandas assigns a numeric, auto-incrementing index to each DataFrame you create.
Why is Indexing Important?
Indexing is important for two main reasons:
- Identification: Unique identifiers help in identifying rows with specific characteristics.
- Selection: Indexes make data selection and manipulation faster and easier.
How to Set an Index in Pandas DataFrame
Setting an index in a DataFrame is straightforward. You can use the set_index()
function, which takes a column name (or a list of column names) as an argument.
import pandas as pd
# Create a simple dataframe
df = pd.DataFrame({
'A': ['foo', 'bar', 'baz', 'qux'],
'B': ['one', 'one', 'two', 'three'],
'C': [1, 2, 3, 4],
'D': [10, 20, 30, 40]
})
# Set 'A' as the index
df.set_index('A', inplace=True)
Output:
B C D
A
foo one 1 10
bar one 2 20
baz two 3 30
qux three 4 40
The inplace=True
argument modifies the original DataFrame. If you don’t include this argument, the function will return a new DataFrame.
Multi-Indexing in Pandas
Pandas also supports multiple indexes, which can be useful for higher dimensional data. You can create a multi-index DataFrame by passing a list of column names to the set_index()
function.
# Set 'A' and 'B' as the index
df.set_index(['A', 'B'], inplace=True)
Outpu:
C D
A B
foo one 1 10
bar one 2 20
baz two 3 30
qux three 4 40
Resetting the Index
If you want to revert your DataFrame to the default integer index, you can use the reset_index()
function.
# Reset the index
df.reset_index(inplace=True)
Output:
A B C D
0 foo one 1 10
1 bar one 2 20
2 baz two 3 30
3 qux three 4 40
Indexing for Performance
Indexes are not just for identification and selection. They can also significantly improve performance. When you perform a task that uses the index, like a data lookup or a merge operation, Pandas uses a hash-based algorithm, which is extremely fast.
However, keep in mind that indexes require memory to store. If you’re working with a large DataFrame, you might need to balance the performance benefits of indexing with the memory overhead.
Conclusion
Indexing is a powerful feature of the Pandas library that allows you to optimize your data for efficient querying and analysis. By understanding how to create and use indexes, you can make your data science workflows in Python faster and more efficient.
Remember, the key to effective indexing is understanding your data and how you’re going to use it. With this knowledge, you can choose the right columns to index and optimize your data for your specific use case.
We hope this guide has helped you understand how to create an index for a Python Pandas DataFrame. Stay tuned for more tips and tricks to help you get the most out of your data science toolkit.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.