How to Create a Dictionary of Two Pandas DataFrame Columns
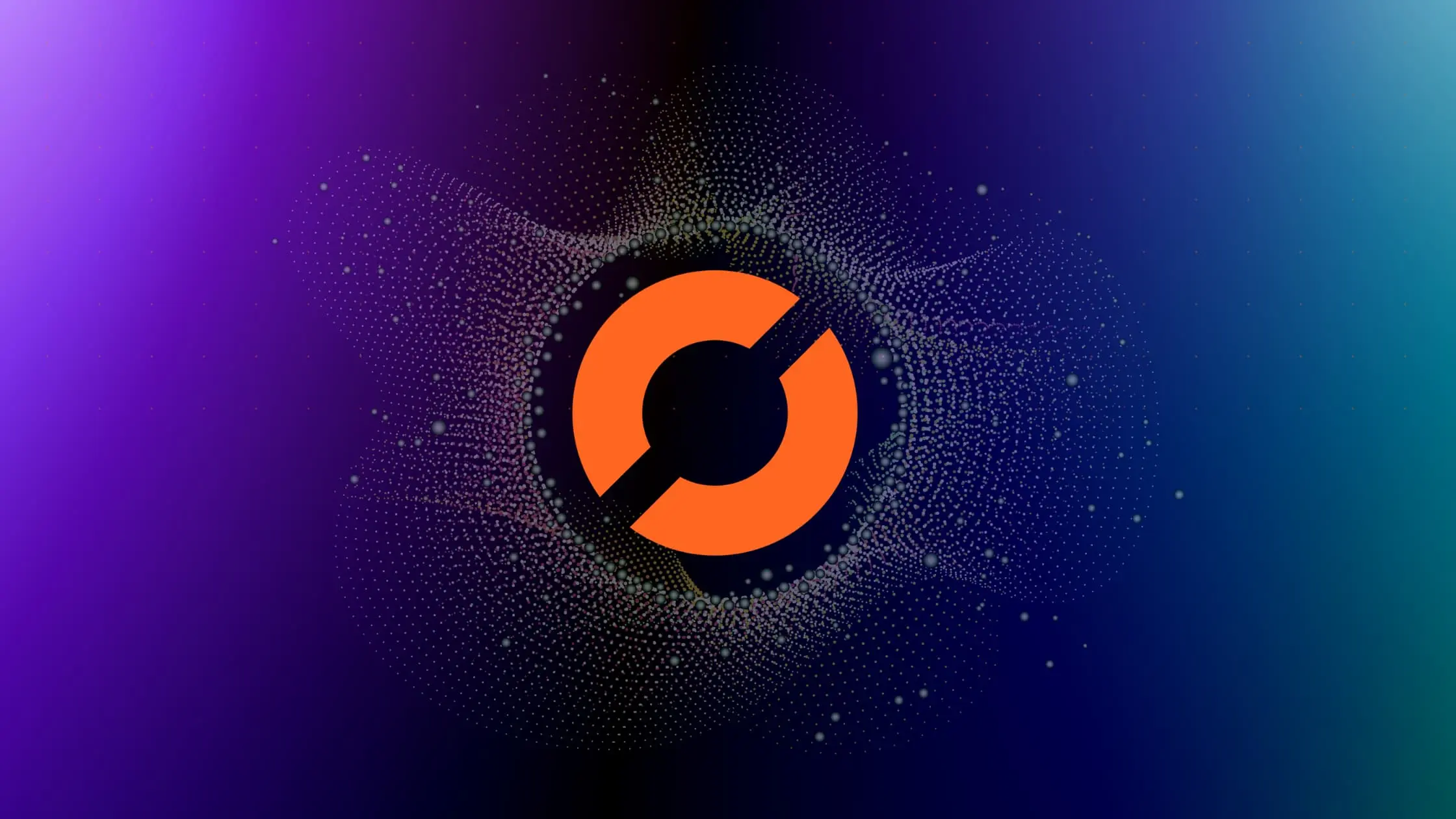
As a data scientist or software engineer, you will often come across situations where you need to create a dictionary of two pandas DataFrame columns. This can be a tricky task, especially if you are new to pandas or Python. In this article, we will explain how to create a dictionary of two pandas DataFrame columns step-by-step.
What is a Pandas DataFrame?
Before we dive into the details of creating a dictionary of two pandas DataFrame columns, let’s first understand what a pandas DataFrame is. In simple terms, a pandas DataFrame is a two-dimensional table-like data structure with rows and columns. It is similar to a spreadsheet or a SQL table and is a fundamental data structure in pandas.
How to Create a Dictionary of Two Pandas DataFrame Columns
Now, let’s get to the main topic of this article - how to create a dictionary of two pandas DataFrame columns. Here are the steps you need to follow:
Step 1: Import the Required Libraries
The first step is to import the required libraries - pandas and numpy. You can do this using the following code:
import pandas as pd
import numpy as np
Step 2: Create a Pandas DataFrame
The next step is to create a pandas DataFrame. You can do this using the following code:
df = pd.DataFrame({'col1': [1, 2, 3, 4, 5],
'col2': ['a', 'b', 'c', 'd', 'e']})
This will create a pandas DataFrame with two columns - ‘col1’ and ‘col2’.
Step 3: Convert the DataFrame to a Dictionary
Using to_dict()
method
Pandas DataFrames come with a to_dict
method that allows for flexible conversion of DataFrame elements to dictionaries. This method can be employed to create a dictionary from two specific columns.
dict_df = df.set_index('col1')['col2'].to_dict()
print(dict_df)
Output:
{1: 'a', 2: 'b', 3: 'c', 4: 'd', 5: 'e'}
This code sets the index of the DataFrame to col1
and then converts the col2
column to a dictionary using the to_dict()
method.
Using zip
method
The zip
function is a versatile tool for combining two iterables. In the context of Pandas DataFrames, we can leverage it to create a dictionary from two columns.
# Using zip and dict
dict_df = dict(zip(df1['col1'], df1['col2']))
print(dict_df)
Output:
{1: 'a', 2: 'b', 3: 'c', 4: 'd', 5: 'e'}
Conclusion
In conclusion, creating a dictionary from two Pandas DataFrame columns can be achieved through various methods, including using the zip
function, and the to_dict
method. The choice of method depends on the specific requirements of your task and the structure of your data.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.