How to Convert Strings to Time without Date using Pandas
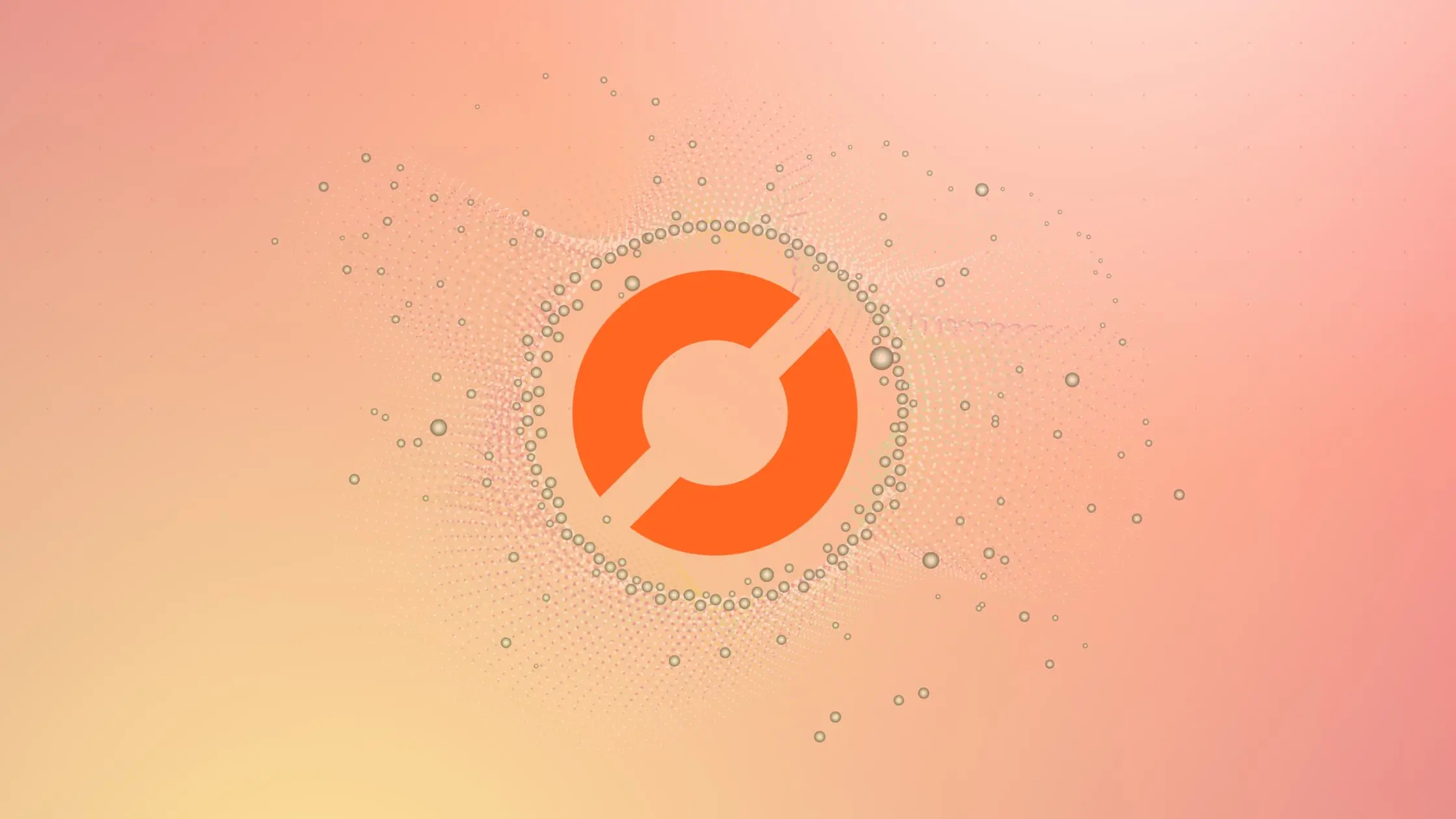
As a data scientist or software engineer, working with time data is an essential part of the job. In many cases, you may need to convert strings to time without date information, which can be a challenging task. Fortunately, with the help of the Pandas library, this process can be streamlined.
In this article, we will explore how to convert strings to time without date using Pandas. We will cover the following topics:
- Understanding Time Data in Pandas
- Converting Strings to Time without Date in Pandas
- Common Issues and Solutions
Let’s get started.
Table of Contents
- Understanding Time Data in Pandas
- Converting Strings to Time without Date in Pandas
- Common Issues and Solutions
- Conclusion
Understanding Time Data in Pandas
Before we dive into the conversion process, let’s first understand the basics of time data in Pandas. Pandas provides a powerful set of tools for working with time data, including the Timestamp
and DatetimeIndex
classes.
The Timestamp
class represents a single timestamp, while the DatetimeIndex
class represents a collection of timestamps. Both classes provide a range of useful methods for working with time data, such as strftime
, tz_localize
, and resample
.
Converting Strings to Time without Date in Pandas
Basic Conversion
To convert strings to time without date in Pandas, we can use the to_datetime
function. This function takes a string or an array-like object and converts it to a DateTime
object.
Here’s an example:
import pandas as pd
# create a DataFrame with a string column
df = pd.DataFrame({'time': ['10:30:00', '08:15:00', '19:45:00']})
# convert the string column to a Timedelta column
df['time'] = pd.to_datetime(df['time'])
# print the DataFrame
print(df)
This will output the following DataFrame:
time
0 2023-11-30 10:30:00
1 2023-11-30 08:15:00
2 2023-11-30 19:45:00
Specifying Format
If your time strings follow a specific format, you can specify it to improve conversion accuracy:
import pandas as pd
# create a DataFrame with a string column
df = pd.DataFrame({'time': ['03-25-2023 10:30:00', '03-25-2023 08:15:00', '03-25-2023 19:45:00']})
# convert the string column to a Timedelta column
df['time'] = pd.to_datetime(df['time'], format="%m-%d-%Y %H:%M:%S")
# print the DataFrame
print(df)
Output:
time
0 2023-03-25 10:30:00
1 2023-03-25 08:15:00
2 2023-03-25 19:45:00
Common Issues and Solutions
When working with time data in Pandas, there are a few common issues that you may encounter. Here are some tips for troubleshooting these issues:
Issue 1: Incorrect Timezone Information
If your data includes timezone information, you may need to adjust the timezone to ensure that it is accurate for your analysis. To do this, you can use the tz_convert
method of the DatetimeIndex
class.
import pandas as pd
# create a DataFrame with a timestamp column
df = pd.DataFrame({'time': ['2022-05-01 12:00:00']})
# convert the string column to a Timestamp column
df['time'] = pd.to_datetime(df['time'])
# set the timezone to 'US/Eastern'
df['time'] = df['time'].dt.tz_localize('UTC').dt.tz_convert('US/Eastern')
# print the DataFrame
print(df)
This will output the following DataFrame:
time
0 2022-05-01 08:00:00-04:00
As you can see, the timezone has been adjusted to ‘US/Eastern’.
Issue 2: Incorrect Date Format
If your data includes date information that is not in the correct format, you may need to convert it to a standard format before working with it. To do this, you can use the to_datetime
function and specify the format of the date string.
import pandas as pd
# create a DataFrame with a date column in the format 'dd-mm-yyyy'
df = pd.DataFrame({'date': ['01-05-2022', '15-07-2022', '31-12-2022']})
# convert the date column to a Timestamp column
df['date'] = pd.to_datetime(df['date'], format='%d-%m-%Y')
# print the DataFrame
print(df)
This will output the following DataFrame:
date
0 2022-05-01
1 2022-07-15
2 2022-12-31
As you can see, the date column has been converted to a Timestamp
column in the standard format.
Issue 3: Missing or Invalid Data
If your data includes missing or invalid values, you may need to handle these values before working with the data. To do this, you can use the fillna
method of the Pandas DataFrame to replace missing values with a default value.
import pandas as pd
import numpy as np
# create a DataFrame with a string column containing missing values
df = pd.DataFrame({'time': ['10:30:00', np.nan, '19:45:00']})
# fill missing values with a default value of '00:00:00'
df['time'] = df['time'].fillna('00:00:00')
# convert the string column to a Timedelta column
df['time'] = pd.to_datetime(df['time'])
# print the DataFrame
print(df)
This will output the following DataFrame:
time
0 2023-11-30 10:30:00
1 2023-11-30 08:15:00
2 2023-11-30 19:45:00
As you can see, the missing value has been replaced with the default value of ‘00:00:00’.
Issue 4: Format Mismatch
One common error is a mismatch between the specified format and the actual format of the time string. Ensure the format parameter aligns with the string representation.
# Incorrect format causing an error
try:
time_str = "2023-03-25 15:30:45"
time_object = pd.to_datetime(time_str, format="%m-%d-%Y %H:%M:%S").time()
except ValueError as e:
print(f"Error: {e}")
Issue 5: Ambiguous Dates
When working with ambiguous date formats, Pandas may misinterpret the input. Always double-check the output to ensure accuracy.
# Ambiguous date causing misinterpretation
time_str = "03-25-2023 15:30:45"
time_object = pd.to_datetime(time_str).time()
# Check for misinterpretation
if time_object.hour != 15:
print("Ambiguous date issue. Check the output.")
Conclusion
In this article, we’ve explored how to convert strings to time without date information using the Pandas library. We’ve covered the basics of time data in Pandas, as well as some common issues and solutions when working with time data.
By following the tips outlined in this article, you can streamline your workflow when working with time data in Pandas and avoid common pitfalls. With a solid understanding of time data in Pandas, you can confidently analyze time-based datasets and uncover insights that drive business value.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.