How to Convert RGB PIL Image to Numpy Array with 3 Channels: A Guide
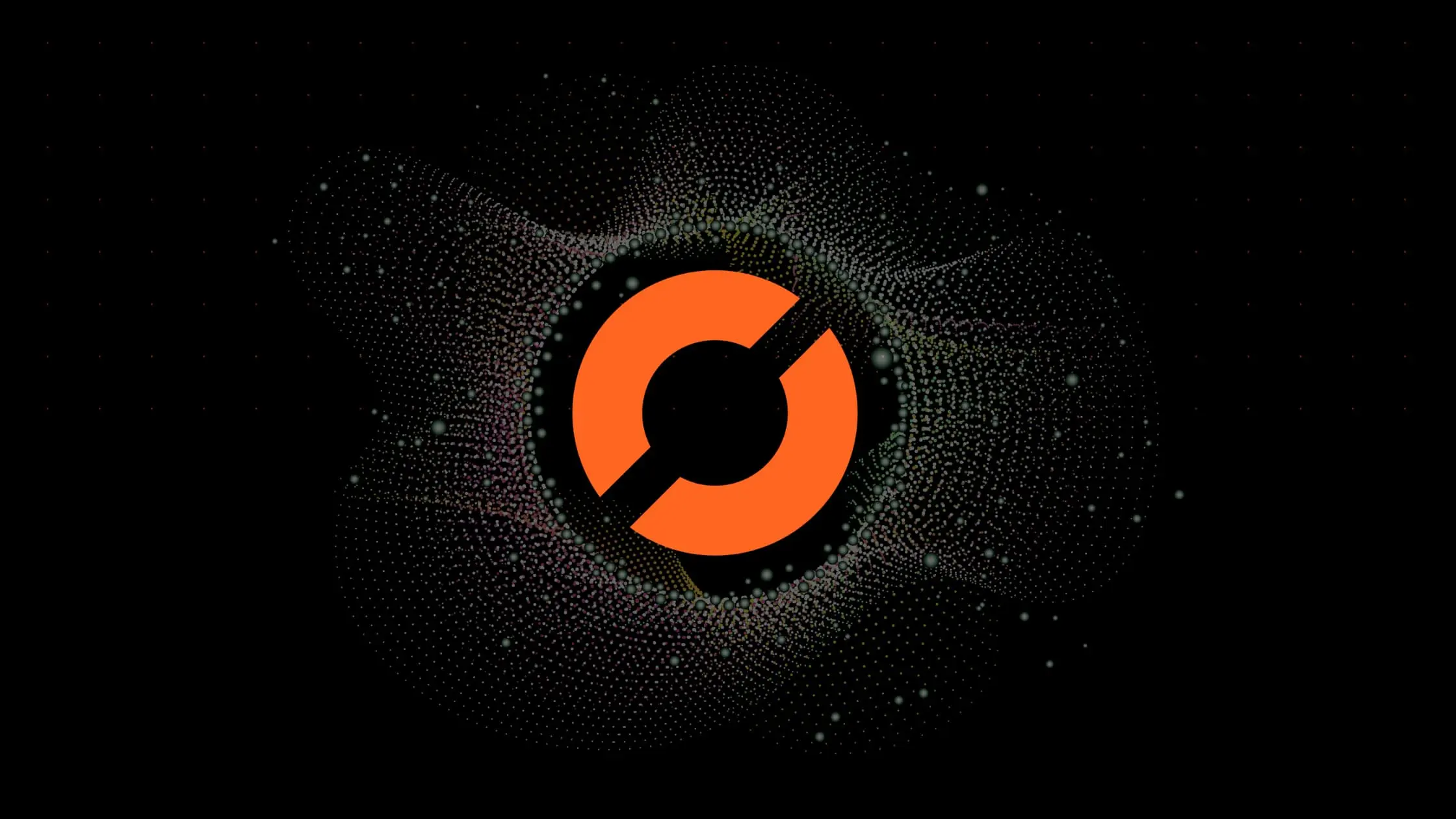
In the realm of image processing, the Python Imaging Library (PIL) and NumPy are two indispensable tools. This blog post will guide you through the process of converting an RGB PIL image to a NumPy array with 3 channels. This conversion is a common task in image processing, machine learning, and computer vision applications.
Table of Contents
- Introduction
- Prerequisites
- Step 1: Importing the Necessary Libraries
- Step 2: Loading the Image
- Step 3: Converting the Image to an RGB Image
- Step 4: Converting the RGB Image to a NumPy Array
- Step 5: Verifying the Conversion
- Pros and Cons of Converting RGB PIL Images to NumPy Arrays
- Conclusion
Prerequisites
Before we dive in, ensure you have the following installed:
- Python 3.6 or later
- PIL (Pillow)
- NumPy
You can install these packages using pip:
pip install pillow numpy
Step 1: Importing the Necessary Libraries
First, we need to import the necessary libraries. We’ll be using PIL for image handling and NumPy for array manipulation.
from PIL import Image
import numpy as np
Step 2: Loading the Image
Next, we load the image using PIL’s Image.open()
function. Replace 'image.jpg'
with the path to your image.
img = Image.open('image.jpg')
Step 3: Converting the Image to an RGB Image
To ensure our image is in RGB format, we use the convert()
function. If the image is already in RGB, this step won’t change anything.
img_rgb = img.convert('RGB')
Step 4: Converting the RGB Image to a NumPy Array
Now, we’re ready to convert our RGB image to a NumPy array. We use the np.array()
function for this.
img_array = np.array(img_rgb)
The resulting img_array
is a 3D NumPy array, where the first dimension represents the height, the second dimension represents the width, and the third dimension represents the RGB channels.
Step 5: Verifying the Conversion
To verify that our conversion was successful, we can print the data types.
print(img)
print(img_array.shape)
Output:
<class 'PIL.JpegImagePlugin.JpegImageFile'>
<class 'numpy.ndarray'>
Pros and Cons of Converting RGB PIL Images to NumPy Arrays
Pros
Versatility: The combination of PIL and NumPy provides a versatile solution for handling and manipulating images, catering to a wide range of image processing tasks.
Ease of Use: The provided code demonstrates a straightforward process, making it accessible for users with varying levels of experience in Python and image processing.
Widely Used Libraries: PIL and NumPy are widely adopted in the Python community, ensuring ample community support, documentation, and resources for troubleshooting and further development.
Cons
Library Dependencies: The reliance on external libraries like PIL and NumPy may lead to increased project dependencies, potentially affecting the project’s portability or compatibility with certain environments.
Resource Intensity: Loading large images into NumPy arrays may consume a significant amount of memory. This can be a limitation when working with high-resolution images or in memory-constrained environments.
Conclusion
And there you have it! You’ve successfully converted an RGB PIL image to a NumPy array with 3 channels. This process is a fundamental part of many image processing tasks, and understanding it can greatly enhance your data science, machine learning, and computer vision projects.
Remember, the power of Python lies in its libraries. By leveraging PIL and NumPy, you can manipulate images and arrays with ease.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.