How to Convert Pandas DataFrame to Spark DataFrame
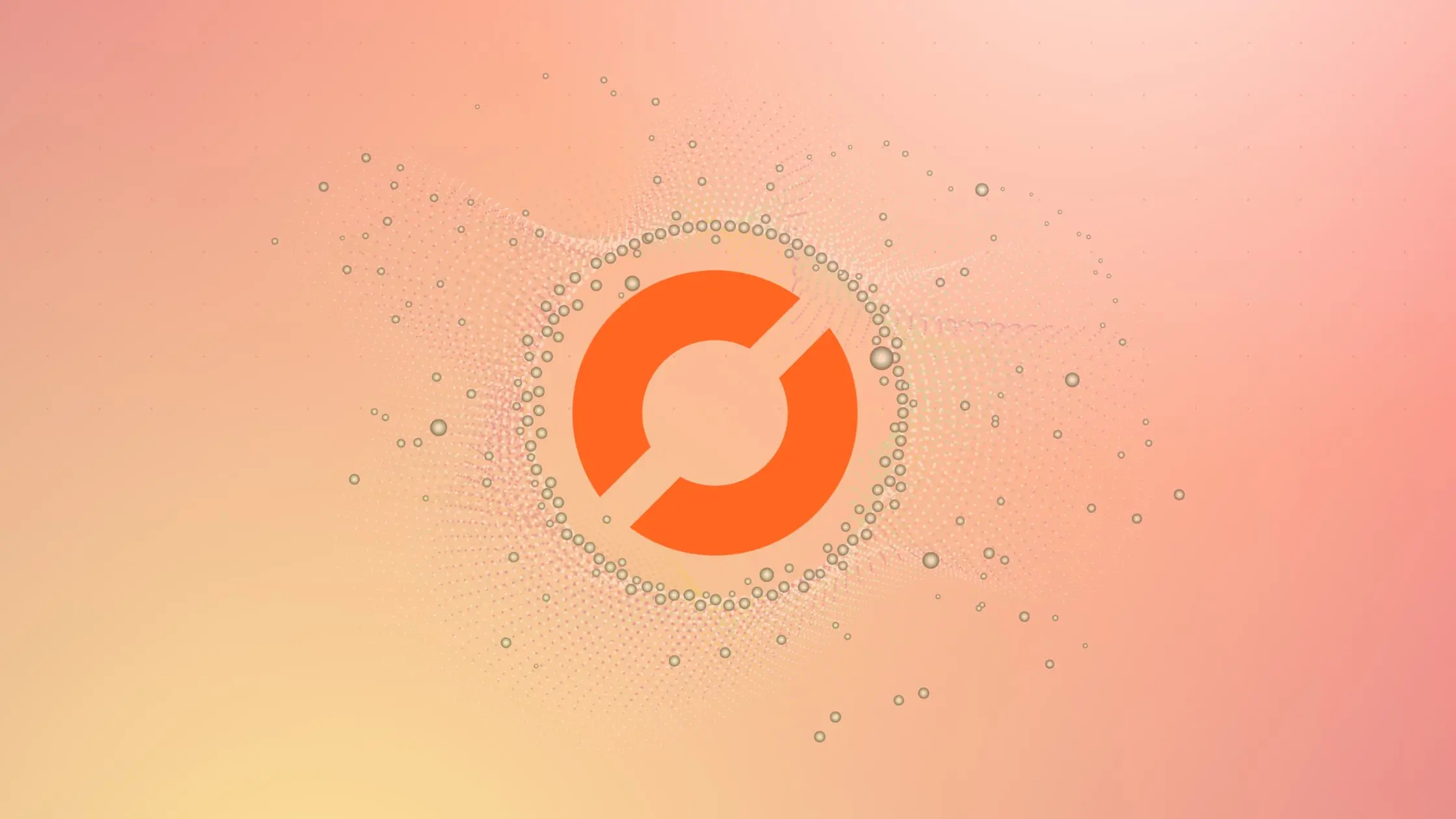
As a data scientist or software engineer, you may often find yourself working with large datasets that require distributed computing. Apache Spark is a powerful distributed computing framework that can handle big data processing tasks efficiently. One of the most common tasks in data processing is converting a Pandas DataFrame into a Spark DataFrame.
In this article, we will explore how to convert a Pandas DataFrame to a Spark DataFrame, step-by-step. We will assume that you have a basic understanding of Python, Pandas, and Spark.
Table of Contents
- Introduction
- What is a Pandas DataFrame?
- What is a Spark DataFrame?
- Why Convert a Pandas DataFrame to a Spark DataFrame?
- Prerequisites
- Conclusion
What is a Pandas DataFrame?
A Pandas DataFrame is a two-dimensional table-like data structure that is used to store and manipulate data in Python. It is similar to a spreadsheet or a SQL table and consists of rows and columns. You can perform various operations on a Pandas DataFrame, such as filtering, grouping, and aggregation.
What is a Spark DataFrame?
A Spark DataFrame is a distributed collection of data organized into named columns. It is similar to a Pandas DataFrame but is designed to handle big data processing tasks efficiently. Spark DataFrames are built on top of RDDs (Resilient Distributed Datasets) and are immutable, meaning that you cannot modify them once they are created.
Why convert a Pandas DataFrame to a Spark DataFrame?
There are several reasons why you may want to convert a Pandas DataFrame to a Spark DataFrame:
Scalability: Pandas is designed to work on a single machine and may not be able to handle large datasets efficiently. Spark, on the other hand, can distribute the workload across multiple machines, making it ideal for big data processing tasks.
Parallelism: Spark can perform operations on data in parallel, which can significantly improve the performance of data processing tasks.
Integration: Spark integrates seamlessly with other big data technologies, such as Hadoop and Kafka, making it a popular choice for big data processing tasks.
Prerequisites
Java: Install Java Development Kit (JDK). Set JAVA_HOME environment variable.
Hadoop (Optional for Local Development): Download and install Hadoop and set HADOOP_HOME environment variable.
Apache Spark: Download and extract Spark, set SPARK_HOME environment variable and add Spark’s bin directory to the system PATH.
Hadoop Configuration (Optional for Local Development): Edit core-site.xml and hdfs-site.xml in Hadoop’s etc/hadoop directory, format Hadoop Namenode (First Time Only) and run hdfs namenode -format in the Hadoop bin directory.
Step-by-Step Guide to Converting a Pandas DataFrame to a Spark DataFrame
Now that we understand why we may want to convert a Pandas DataFrame to a Spark DataFrame, let’s dive into the step-by-step guide:
Step 1: Install PySpark
PySpark is the Python API for Apache Spark. You will need to install PySpark before you can use it to convert a Pandas DataFrame to a Spark DataFrame. You can install PySpark using pip:
pip install pyspark
Step 2: Create a SparkSession
A SparkSession is the entry point to using Spark. It provides a way to interact with Spark using APIs. You can create a SparkSession using the following code:
from pyspark.sql import SparkSession
spark = SparkSession.builder \
.appName("Pandas to Spark") \
.getOrCreate()
Step 3: Load the Pandas DataFrame
Before we can convert a Pandas DataFrame to a Spark DataFrame, we need to load the Pandas DataFrame into memory. You can do this using the Pandas read_csv()
method or by creating a Pandas DataFrame manually.
import pandas as pd
df = pd.read_csv("data.csv")
Step 4: Create a Spark DataFrame
Now that we have loaded the Pandas DataFrame, we can create a Spark DataFrame using the createDataFrame()
method of the SparkSession object.
spark_df = spark.createDataFrame(df)
Step 5: Inspect the Spark DataFrame
You can inspect the Spark DataFrame using the printSchema()
method. This will show you the schema of the Spark DataFrame, including the data types of each column.
spark_df.printSchema()
Output:
|-- name: string (nullable = true)
|-- age: long (nullable = true)
|-- city: string (nullable = true)
Step 6: Perform Operations on the Spark DataFrame
Now that we have created a Spark DataFrame, we can perform various operations on it. For example, we can filter the data using the filter()
method:
filtered_df = spark_df.filter(spark_df.age > 30)
filtered_df.show()
Output:
+-------+---+-------------+
| name|age| city|
+-------+---+-------------+
| Alice| 32|San Francisco|
| Eve| 35| Chicago|
|Charlie| 40| Houston|
+-------+---+-------------+
Step 7: Write the Spark DataFrame to a File
Finally, we can write the Spark DataFrame to a file using the write()
method:
filtered_df.write.csv("filtered_data.csv")
Conclusion
In this article, we have explored how to convert a Pandas DataFrame to a Spark DataFrame step-by-step. We have also discussed why you may want to convert a Pandas DataFrame to a Spark DataFrame and the benefits of using Spark for big data processing tasks.
By following the steps outlined in this article, you should now be able to convert a Pandas DataFrame to a Spark DataFrame and leverage the power of Spark for your big data processing tasks.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.