How to Convert Pandas Column Names to a List in Python
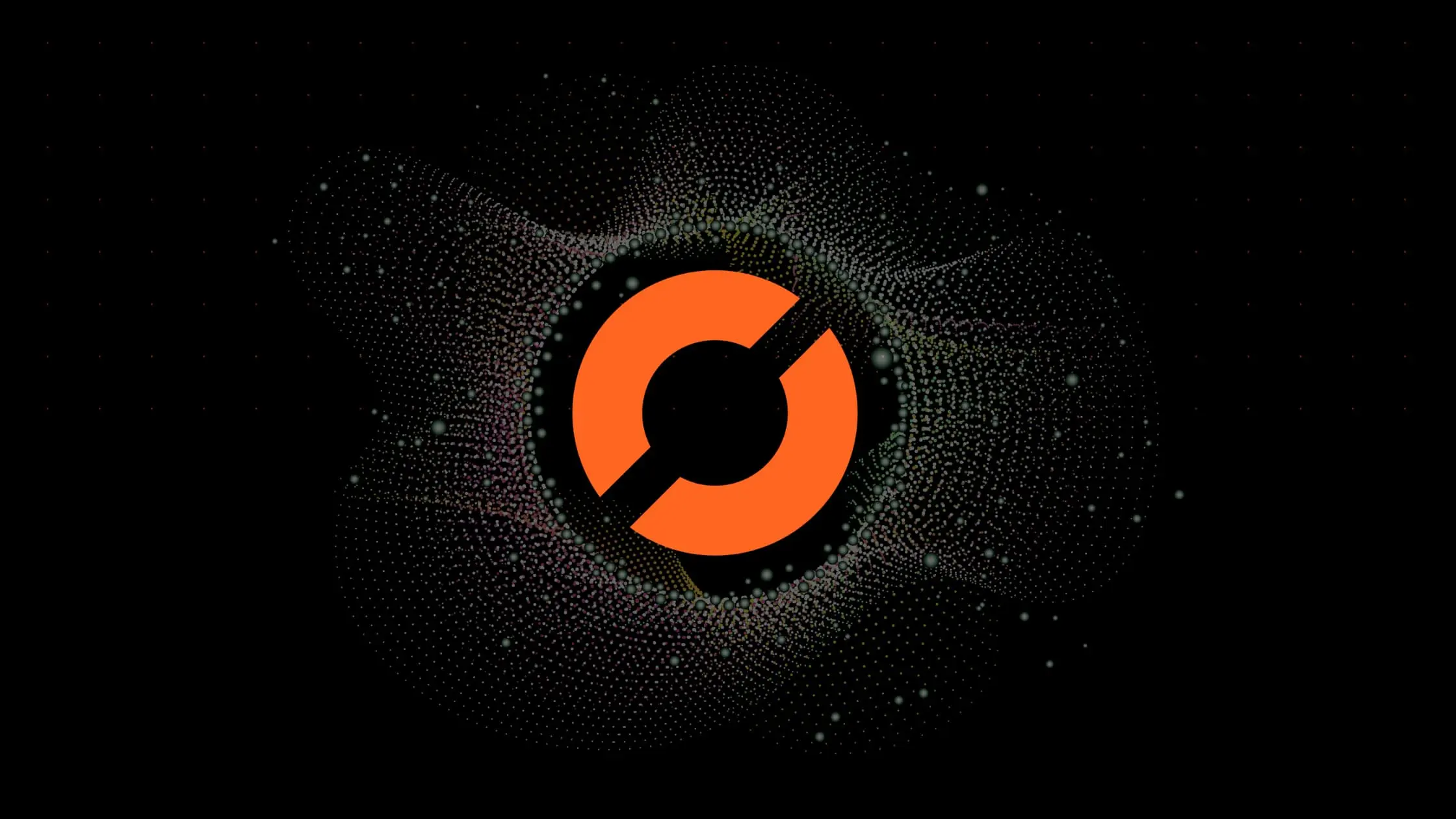
As a data scientist or software engineer working with Python, you will often find yourself working with pandas, a popular library for data manipulation and analysis. One common task you may encounter is the need to convert pandas column names to a list. In this blog post, we will explore how to accomplish this task using pandas, and provide some tips and tricks for working with pandas column names.
Table of Contents
- What is pandas?
- How to convert pandas column names to a list
- Tips and tricks for working with pandas column names
- Handling Errors
- Conclusion
What is pandas?
Pandas is a powerful library in Python for data manipulation and analysis. It provides data structures for efficiently storing and manipulating large datasets, and functions for performing common data operations such as filtering, grouping, and aggregation. Pandas is widely used in data science and machine learning projects, as it provides an intuitive and efficient interface for working with structured data.
How to convert pandas column names to a list
Converting pandas column names to a list is a simple and straightforward task. The columns
attribute of a pandas DataFrame contains a list of the column names. To convert these column names to a list, you can simply call the tolist()
method on the columns
attribute.
import pandas as pd
# create a sample DataFrame
data = {'name': ['Alice', 'Bob', 'Charlie'], 'age': [25, 30, 35], 'gender': ['female', 'male', 'male']}
df = pd.DataFrame(data)
# convert column names to a list
cols_list = df.columns.tolist()
print(cols_list)
# Output: ['name', 'age', 'gender']
In the above code, we first create a sample DataFrame with three columns: ‘name’, ‘age’, and ‘gender’. We then call the columns
attribute to get a list of the column names, and convert this list to a Python list using the tolist()
method. Finally, we print the resulting list of column names.
Tips and tricks for working with pandas column names
Working with pandas column names can sometimes be tricky, especially when dealing with complex datasets or data preprocessing tasks. Here are some tips and tricks to keep in mind when working with pandas column names:
1. Renaming columns
You can rename columns in a pandas DataFrame using the rename()
method. This method takes a dictionary of the form {old_name: new_name}
as its argument. For example:
# rename the 'name' column to 'full_name'
df.rename(columns={'name': 'full_name'}, inplace=True)
2. Dropping columns
You can drop columns from a pandas DataFrame using the drop()
method. This method takes a list of column names to drop as its argument. For example:
# drop the 'gender' column
df.drop(columns=['gender'], inplace=True)
3. Accessing columns by name
You can access columns in a pandas DataFrame by name using either bracket notation or dot notation. For example:
# access the 'name' column using bracket notation
names = df['name']
# access the 'age' column using dot notation
ages = df.age
4. Handling missing column names
If your DataFrame has missing column names, you can assign column names using the columns
attribute. For example:
# create a DataFrame with missing column names
data = [[1, 2], [3, 4]]
df = pd.DataFrame(data)
# assign column names
df.columns = ['col1', 'col2']
5. Selecting multiple columns
You can select multiple columns from a pandas DataFrame using a list of column names. For example:
# select the 'name' and 'age' columns
subset = df[['name', 'age']]
Handling Errors
- Case Sensitivity: When working with column names, it’s essential to be aware that pandas is case-sensitive. Users may encounter issues if they try to access or manipulate column names using the wrong case. For example:
# Incorrect: 'Name' instead of 'name'
names = df['Name']
- Whitespace in Column Names: Column names with leading or trailing whitespaces can be problematic. Users might not realize the presence of whitespaces, leading to errors when performing operations. It’s good practice to clean column names from whitespaces. For instance:
# Incorrect: ' name' with leading whitespace
names = df[' name']
- Nonexistent Column Names: Users might attempt to access or manipulate columns that do not exist in the DataFrame. This can lead to KeyError or unexpected behavior. It’s advisable to check the existence of a column before performing operations. For example:
# Incorrect: 'salary' does not exist in the DataFrame
df.drop(columns=['salary'], inplace=True)
- Handling Null Values: When converting column names to a list, users should consider scenarios where the DataFrame itself might contain null values. The
tolist()
method would raise an error if applied directly to columns with null values. It’s good practice to handle null values appropriately. For instance:
# Incorrect: if 'name' column contains null values
cols_list = df['name'].tolist()
Avoiding inplace=True in Examples: The use of
inplace=True
in examples might be misleading for new users, as it modifies the DataFrame in place. While it’s suitable for some scenarios, it’s crucial to emphasize the potential risks of usinginplace=True
, especially in a tutorial setting.List Comprehension for Multiple Columns: While the article correctly demonstrates converting column names to a list using the
tolist()
method, users might also be interested in using list comprehension for multiple columns. This approach is useful when a subset of columns needs to be selected:
# Alternative: Using list comprehension
cols_list = [col for col in df.columns if col not in ['gender']]
Conclusion
In this blog post, we explored how to convert pandas column names to a list in Python. We also provided some tips and tricks for working with pandas column names, including renaming columns, dropping columns, accessing columns by name, handling missing column names, and selecting multiple columns. With these tools in your toolbox, you’ll be able to efficiently work with pandas column names in your data science and software engineering projects.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.