How to Convert Datetime to Timestamp in Python Pandas using the dt Accessor
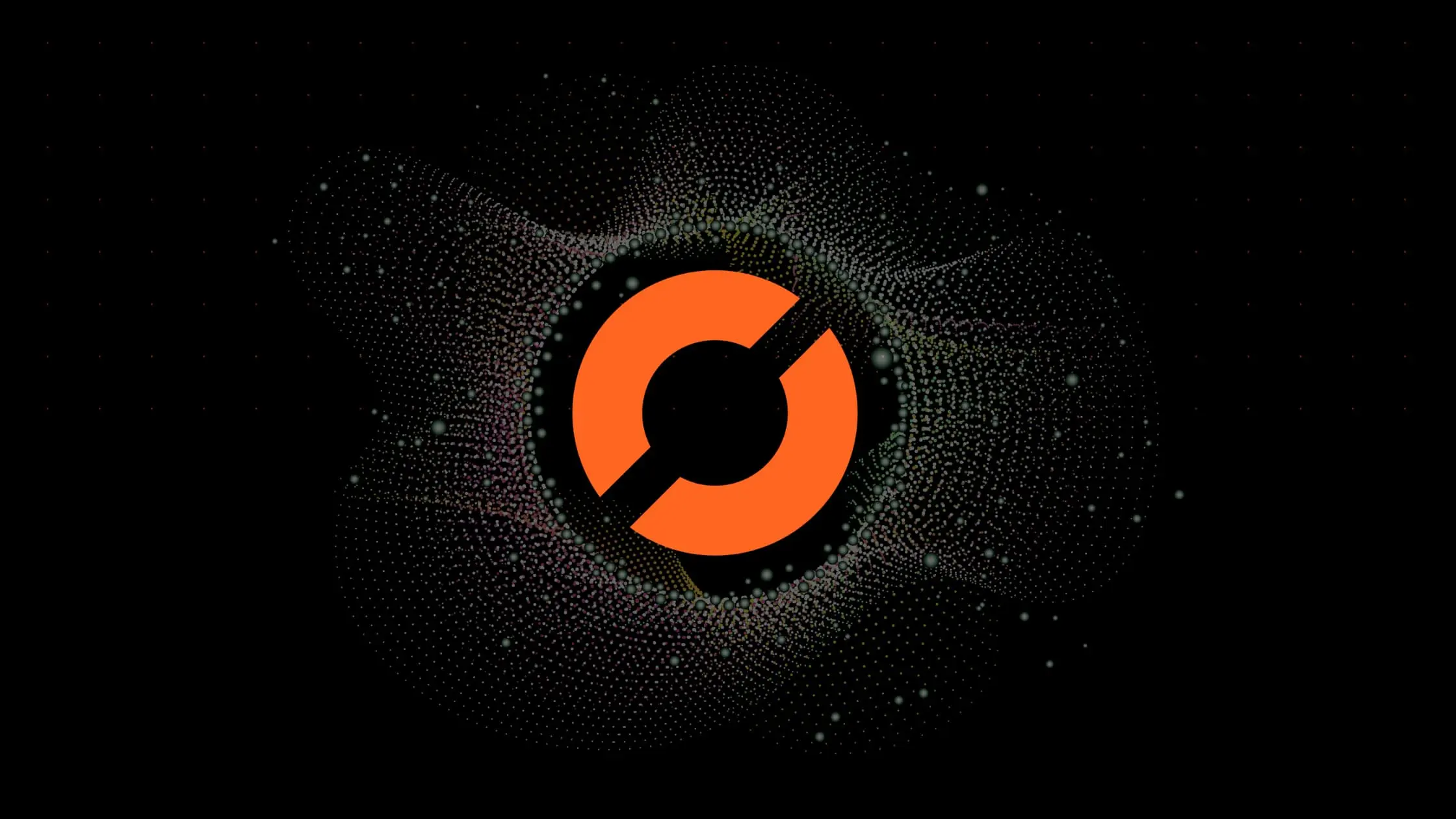
Python Pandas is a powerful data manipulation library that provides many functions to work with dates and times effortlessly. One of the most common tasks in data analysis is converting datetime to timestamp. In this article, we will explore the best practices for converting datetime to timestamp using the dt
accessor in Python Pandas.
What is Datetime and Timestamp?
Before we dive into the conversion process, let’s define what datetime and timestamp mean. In Python, datetime refers to a data type that represents a date and time. It includes the year, month, day, hour, minute, second, and microsecond. Timestamp, on the other hand, refers to the number of seconds or nanoseconds that have elapsed since January 1, 1970, at UTC.
Why Convert Datetime to Timestamp?
There are several reasons why you might want to convert datetime to timestamp. One of the main reasons is that timestamps are easier to work with when performing calculations or comparisons. Timestamps are also more efficient to store in a database or transfer over the network.
Converting Datetime to Timestamp using the dt Accessor
Python Pandas provides a convenient way to convert datetime to timestamp using the dt
accessor. The dt
accessor is a powerful tool that allows you to manipulate datetime objects with ease.
To convert datetime to timestamp using the dt
accessor, you need to follow these steps:
- Convert the datetime column to a pandas datetime object using the
to_datetime()
function. - Use the
astype()
function to convert the datetime column to an integer. - Divide the resulting integer by the number of nanoseconds in a second using the
div()
function.
Here is an example that demonstrates how to convert datetime to timestamp using the dt
accessor:
import pandas as pd
# create a sample dataframe
df = pd.DataFrame({'datetime': ['2021-01-01 00:00:00', '2021-01-01 01:00:00', '2021-01-01 02:00:00']})
# convert the datetime column to a pandas datetime object
df['datetime'] = pd.to_datetime(df['datetime'])
# convert the datetime column to an integer
df['timestamp'] = df['datetime'].astype(int)
# divide the resulting integer by the number of nanoseconds in a second
df['timestamp'] = df['timestamp'].div(10**9)
print(df)
Output:
datetime timestamp
0 2021-01-01 00:00:00 1.609942e+09
1 2021-01-01 01:00:00 1.609946e+09
2 2021-01-01 02:00:00 1.609949e+09
In this example, we create a sample dataframe that contains a datetime column. We then convert the datetime column to a pandas datetime object using the to_datetime()
function. Next, we use the astype()
function to convert the datetime column to an integer. Finally, we divide the resulting integer by the number of nanoseconds in a second using the div()
function to get the timestamp.
Conclusion
Converting datetime to timestamp is a common task in data analysis. In this article, we have shown you how to convert datetime to timestamp using the dt
accessor in Python Pandas. By following the steps outlined in this article, you can easily convert datetime to timestamp and improve the efficiency of your data analysis.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.