How to Convert Datetime to String with Series in Pandas
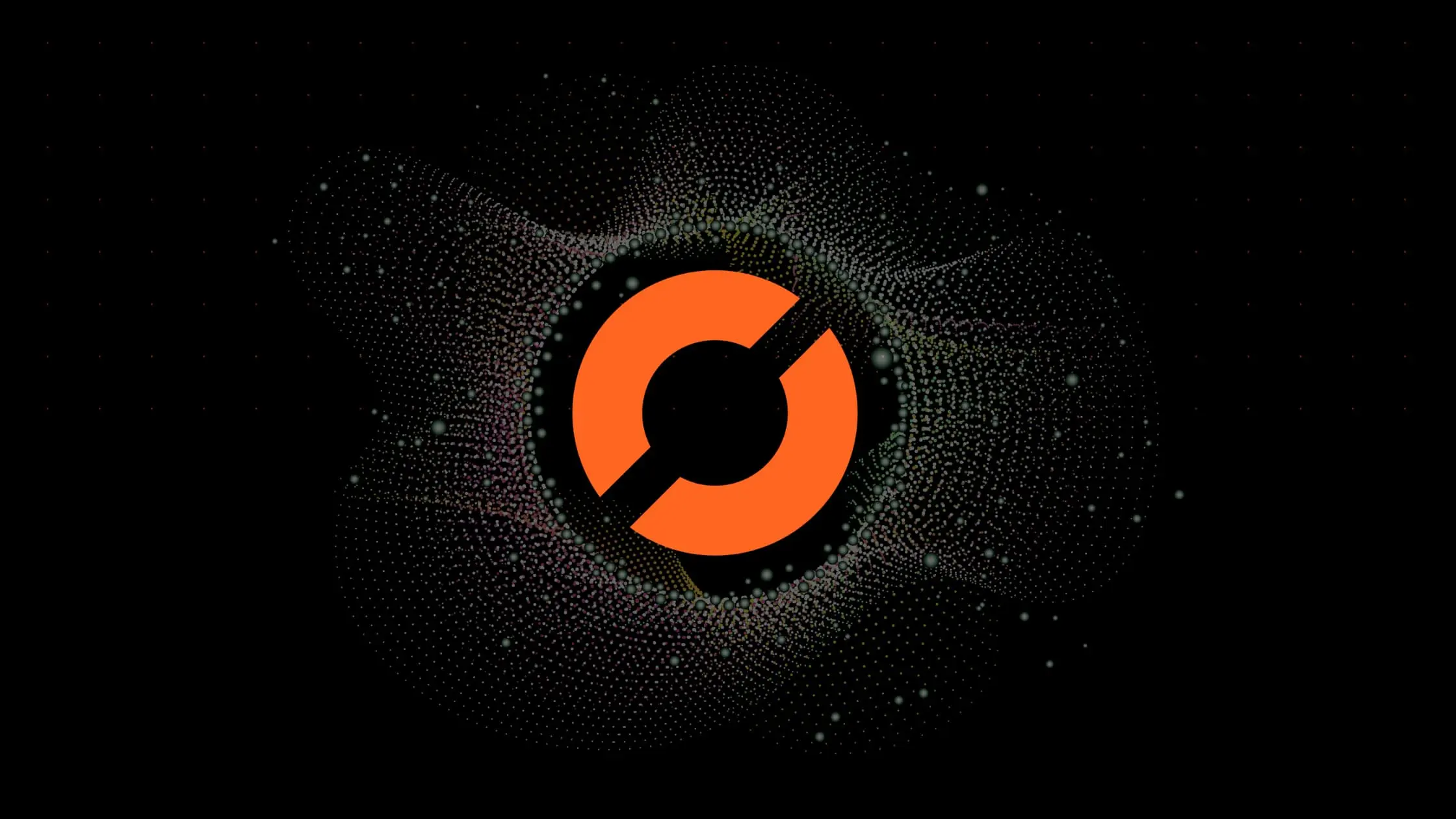
Table of Contents
- Introduction to Pandas
- Converting datetime to string in Pandas
- Common Errors: Watch Out for These Traps!
- Conclusion
Introduction to Pandas?
Pandas is an open-source data manipulation library for Python. It allows you to easily work with structured data, such as tables or spreadsheets, and perform various operations on them, such as filtering, sorting, grouping, and more. Pandas is widely used in data science, machine learning, and other fields that deal with data analysis.
Converting datetime to string in Pandas
In Pandas, datetime objects are represented by the Timestamp
data type. To convert datetime to string with series in Pandas, we can use the strftime()
method, which formats a Timestamp
object according to a specified format string.
Let’s start by creating a sample dataset with datetime data:
import pandas as pd
data = {'date': ['2022-01-01 09:00:00', '2022-01-02 10:30:00', '2022-01-03 12:15:00']}
df = pd.DataFrame(data)
df['date'] = pd.to_datetime(df['date'])
print(df)
Output:
date
0 2022-01-01 09:00:00
1 2022-01-02 10:30:00
2 2022-01-03 12:15:00
Here, we have created a simple Pandas DataFrame with a column named 'date'
containing datetime strings, and converted it to a Timestamp
object using the to_datetime()
method.
Now, let’s say we want to format the date column to display only the date in the format YYYY/MM/DD
. We can do this using the strftime()
method:
df['date'] = df['date'].dt.strftime('%Y/%m/%d')
print(df)
Output:
date
0 2022/01/01
1 2022/01/02
2 2022/01/03
Here, we have used the dt
accessor to apply the strftime()
method to each element of the 'date'
column, and passed the format string '%Y/%m/%d'
to display the year, month, and day components of the date.
We can also include the time component in the string format by adding the %H:%M:%S
placeholders for hour, minute, and second:
df['date'] = df['date'].dt.strftime('%Y/%m/%d %H:%M:%S')
print(df)
Output:
date
0 2022/01/01 09:00:00
1 2022/01/02 10:30:00
2 2022/01/03 12:15:00
Now, the 'date'
column contains both the date and time components in the specified format.
Common Errors: Watch Out for These Traps!
Missing “pandas”: Don’t forget to install “pandas” before casting your data manipulation spells!
Format Code Fumbles: Double-check your format codes in strftime(). Mixing up %m (month) and %d (day) can lead to unexpected results.
Timezone Travelers: Be mindful of different timezones! If your data spans different zones, consider using methods like .tz_localize() for proper handling.
Wrong Data Type Woes: Make sure you’re applying strftime() to actual datetime objects. Accidentally casting it on strings can cause errors.
Conclusion
In this tutorial, we have shown you how to convert datetime to string with series in Pandas using the strftime()
method. This method allows you to format datetime objects according to a specified string format, and convert them to a string representation that can be used for various purposes, such as reporting or visualization. We hope this tutorial has been helpful in your data analysis tasks with Pandas.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.